The Cayenne MQTT mbed Library provides functions to easily connect to the Cayenne IoT project builder.
Dependents: Cayenne-ESP8266Interface Cayenne-WIZnet_Library Cayenne-WIZnetInterface Cayenne-X-NUCLEO-IDW01M1 ... more
CayenneUtils.h
00001 /* 00002 The MIT License(MIT) 00003 00004 Cayenne MQTT Client Library 00005 Copyright (c) 2016 myDevices 00006 00007 Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated 00008 documentation files(the "Software"), to deal in the Software without restriction, including without limitation 00009 the rights to use, copy, modify, merge, publish, distribute, sublicense, and / or sell copies of the Software, 00010 and to permit persons to whom the Software is furnished to do so, subject to the following conditions : 00011 The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. 00012 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE 00013 WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT.IN NO EVENT SHALL THE AUTHORS OR 00014 COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00015 ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00016 00017 */ 00018 00019 #ifndef _CAYENNEUTILS_h 00020 #define _CAYENNEUTILS_h 00021 00022 #if defined(__cplusplus) 00023 extern "C" { 00024 #endif 00025 00026 #if defined(WIN32_DLL) || defined(WIN64_DLL) 00027 #define DLLImport __declspec(dllimport) 00028 #define DLLExport __declspec(dllexport) 00029 #elif defined(LINUX_SO) 00030 #define DLLImport extern 00031 #define DLLExport __attribute__ ((visibility ("default"))) 00032 #else 00033 #define DLLImport 00034 #define DLLExport 00035 #endif 00036 00037 #include <stdio.h> 00038 #include "CayenneDefines.h" 00039 00040 enum CayenneReturnCode { CAYENNE_BUFFER_OVERFLOW = -2, CAYENNE_FAILURE = -1, CAYENNE_SUCCESS = 0 }; 00041 00042 /** 00043 * Build a specified topic string. 00044 * @param[out] topicName Returned topic string 00045 * @param[in] length CayenneTopic buffer length 00046 * @param[in] username Cayenne username 00047 * @param[in] clientID Cayennne client ID 00048 * @param[in] topic Cayenne topic 00049 * @param[in] channel The topic channel, use CAYENNE_NO_CHANNEL if none is required, CAYENNE_ALL_CHANNELS if a wildcard is required 00050 * @return CAYENNE_SUCCESS if topic string was created, error code otherwise 00051 */ 00052 DLLExport int CayenneBuildTopic(char* topicName, size_t length, const char* username, const char* clientID, CayenneTopic topic, unsigned int channel); 00053 00054 /** 00055 * Build a specified data payload. 00056 * @param[out] payload Returned payload 00057 * @param[in,out] length Payload buffer length 00058 * @param[in] type Optional type to use for type,unit=value payload, can be NULL 00059 * @param[in] unit Payload unit 00060 * @param[in] value Payload value 00061 * @return CAYENNE_SUCCESS if topic string was created, error code otherwise 00062 */ 00063 DLLExport int CayenneBuildDataPayload(char* payload, size_t* length, const char* type, const char* unit, const char* value); 00064 00065 /** 00066 * Build a specified response payload. 00067 * @param[out] payload Returned payload 00068 * @param[in,out] length Payload buffer length 00069 * @param[in] id ID of message the response is for 00070 * @param[in] error Optional error message, NULL for success 00071 * @return CAYENNE_SUCCESS if topic string was created, error code otherwise 00072 */ 00073 DLLExport int CayenneBuildResponsePayload(char* payload, size_t* length, const char* id, const char* error); 00074 00075 /** 00076 * Parse a topic string in place. This may modify the topic string. 00077 * @param[out] topic Returned Cayenne topic 00078 * @param[out] channel Returned channel, CAYENNE_NO_CHANNEL if there is none 00079 * @param[out] clientID Returned client ID 00080 * @param[in] username Cayenne username 00081 * @param[in] topicName Topic name string 00082 * @param[in] length Topic name string length 00083 * @return CAYENNE_SUCCESS if topic was parsed, error code otherwise 00084 */ 00085 DLLExport int CayenneParseTopic(CayenneTopic* topic, unsigned int* channel, const char** clientID, const char* username, char* topicName, size_t length); 00086 00087 /** 00088 * Parse a null terminated payload in place. This may modify the payload string. 00089 * @param[out] type Returned type, NULL if there is none 00090 * @param[out] unit Returned unit, NULL if there is none 00091 * @param[out] value Returned value, NULL if there is none 00092 * @param[out] id Returned message id, empty string if there is none 00093 * @param[in] topic Cayenne topic 00094 * @param[in] payload Payload string, must be null terminated. 00095 * @return CAYENNE_SUCCESS if topic string was created, error code otherwise 00096 */ 00097 DLLExport int CayenneParsePayload(const char** type, const char** unit, const char** value, const char** id, CayenneTopic topic, char* payload); 00098 00099 #if defined(__cplusplus) 00100 } 00101 #endif 00102 00103 #endif
Generated on Wed Jul 13 2022 15:49:28 by
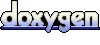