Cayenne Low Power Payload
Dependents: LORAWAN-TTN-CAYENNE-LM35 ER4Lora gps_accelerometer sgam_mdw_test ... more
CayenneLPP.h
00001 #ifndef _CAYENNE_LPP_H_ 00002 #define _CAYENNE_LPP_H_ 00003 00004 #include "mbed.h" 00005 00006 //LPP_BATTERY = // TODO Unsupported in IPSO Smart Object 00007 //LPP_PROXIMITY = // TODO Unsupported in IPSO Smart Object 00008 00009 #define LPP_DIGITAL_INPUT 0 // 1 byte 00010 #define LPP_DIGITAL_OUTPUT 1 // 1 byte 00011 #define LPP_ANALOG_INPUT 2 // 2 bytes, 0.01 signed 00012 #define LPP_ANALOG_OUTPUT 3 // 2 bytes, 0.01 signed 00013 #define LPP_LUMINOSITY 101 // 2 bytes, 1 lux unsigned 00014 #define LPP_PRESENCE 102 // 1 byte, 1 00015 #define LPP_TEMPERATURE 103 // 2 bytes, 0.1°C signed 00016 #define LPP_RELATIVE_HUMIDITY 104 // 1 byte, 0.5% unsigned 00017 #define LPP_ACCELEROMETER 113 // 2 bytes per axis, 0.001G 00018 #define LPP_BAROMETRIC_PRESSURE 115 // 2 bytes 0.1 hPa Unsigned 00019 #define LPP_GYROMETER 134 // 2 bytes per axis, 0.01 °/s 00020 #define LPP_GPS 136 // 3 byte lon/lat 0.0001 °, 3 bytes alt 0.01 meter 00021 00022 00023 // Data ID + Data Type + Data Size 00024 #define LPP_DIGITAL_INPUT_SIZE 3 // 1 byte 00025 #define LPP_DIGITAL_OUTPUT_SIZE 3 // 1 byte 00026 #define LPP_ANALOG_INPUT_SIZE 4 // 2 bytes, 0.01 signed 00027 #define LPP_ANALOG_OUTPUT_SIZE 4 // 2 bytes, 0.01 signed 00028 #define LPP_LUMINOSITY_SIZE 4 // 2 bytes, 1 lux unsigned 00029 #define LPP_PRESENCE_SIZE 3 // 1 byte, 1 00030 #define LPP_TEMPERATURE_SIZE 4 // 2 bytes, 0.1°C signed 00031 #define LPP_RELATIVE_HUMIDITY_SIZE 3 // 1 byte, 0.5% unsigned 00032 #define LPP_ACCELEROMETER_SIZE 8 // 2 bytes per axis, 0.001G 00033 #define LPP_BAROMETRIC_PRESSURE_SIZE 4 // 2 bytes 0.1 hPa Unsigned 00034 #define LPP_GYROMETER_SIZE 8 // 2 bytes per axis, 0.01 °/s 00035 #define LPP_GPS_SIZE 11 // 3 byte lon/lat 0.0001 °, 3 bytes alt 0.01 meter 00036 00037 00038 class CayenneLPP { 00039 public: 00040 CayenneLPP(uint8_t size); 00041 ~CayenneLPP(); 00042 00043 void reset(void); 00044 uint8_t getSize(void); 00045 uint8_t* getBuffer(void); 00046 uint8_t copy(uint8_t* buffer); 00047 00048 uint8_t addDigitalInput(uint8_t channel, uint8_t value); 00049 uint8_t addDigitalOutput(uint8_t channel, uint8_t value); 00050 00051 uint8_t addAnalogInput(uint8_t channel, float value); 00052 uint8_t addAnalogOutput(uint8_t channel, float value); 00053 00054 uint8_t addLuminosity(uint8_t channel, uint16_t lux); 00055 uint8_t addPresence(uint8_t channel, uint8_t value); 00056 uint8_t addTemperature(uint8_t channel, float celsius); 00057 uint8_t addRelativeHumidity(uint8_t channel, float rh); 00058 uint8_t addAccelerometer(uint8_t channel, float x, float y, float z); 00059 uint8_t addBarometricPressure(uint8_t channel, float hpa); 00060 uint8_t addGyrometer(uint8_t channel, float x, float y, float z); 00061 uint8_t addGPS(uint8_t channel, float latitude, float longitude, float meters); 00062 00063 private: 00064 uint8_t *buffer; 00065 uint8_t maxsize; 00066 uint8_t cursor; 00067 00068 00069 }; 00070 00071 00072 #endif
Generated on Wed Jul 13 2022 20:35:54 by
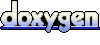