SNIC UART Interface library: Serial to Wi-Fi library for Murata TypeYD Wi-Fi module. For more information about TypeYD: http://www.murata.co.jp/products/microwave/module/lbwb1zzydz/index.html
Dependents: SNIC-xively-jumpstart-demo SNIC-FluentLogger-example TCPEchoServer murataDemo ... more
Fork of YDwifiInterface by
SNIC_WifiInterface.cpp
00001 /* Copyright (C) 2014 Murata Manufacturing Co.,Ltd., MIT License 00002 * muRata, SWITCH SCIENCE Wi-FI module TypeYD-SNIC UART. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00005 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00006 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00007 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00008 * furnished to do so, subject to the following conditions: 00009 * 00010 * The above copyright notice and this permission notice shall be included in all copies or 00011 * substantial portions of the Software. 00012 * 00013 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00014 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00015 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00016 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00017 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00018 */ 00019 #include "SNIC_WifiInterface.h" 00020 #include "SNIC_UartMsgUtil.h" 00021 00022 #define UART_CONNECT_BUF_SIZE 512 00023 unsigned char gCONNECT_BUF[UART_CONNECT_BUF_SIZE]; 00024 static char ip_addr[17] = "\0"; 00025 00026 C_SNIC_WifiInterface::C_SNIC_WifiInterface( PinName tx, PinName rx, PinName cts, PinName rts, PinName reset, PinName alarm, int baud) 00027 { 00028 mUART_tx = tx; 00029 mUART_rx = rx; 00030 mUART_cts = cts; 00031 mUART_rts = rts;; 00032 mUART_baud = baud; 00033 mModuleReset = reset; 00034 } 00035 00036 void C_SNIC_WifiInterface::create( PinName tx, PinName rx, PinName cts, PinName rts, PinName reset, PinName alarm, int baud) 00037 { 00038 mUART_tx = tx; 00039 mUART_rx = rx; 00040 mUART_cts = cts; 00041 mUART_rts = rts;; 00042 mUART_baud = baud; 00043 mModuleReset = reset; 00044 } 00045 00046 C_SNIC_WifiInterface::~C_SNIC_WifiInterface() 00047 { 00048 } 00049 00050 int C_SNIC_WifiInterface::init() 00051 { 00052 C_SNIC_Core *snic_core_p = C_SNIC_Core::getInstance(); 00053 C_SNIC_UartCommandManager *uartCmdMgr_p = snic_core_p->getUartCommand(); 00054 00055 /* Initialize UART */ 00056 snic_core_p->initUart( mUART_tx, mUART_rx, mUART_baud ); 00057 00058 /* Module reset */ 00059 snic_core_p->resetModule( mModuleReset ); 00060 00061 wait(1); 00062 /* Initialize SNIC API */ 00063 // Get buffer for response payload from MemoryPool 00064 tagMEMPOOL_BLOCK_T *payload_buf_p = snic_core_p->allocCmdBuf(); 00065 if( payload_buf_p == NULL ) 00066 { 00067 DEBUG_PRINT("snic_init payload_buf_p NULL\r\n"); 00068 return -1; 00069 } 00070 00071 C_SNIC_Core::tagSNIC_INIT_REQ_T req; 00072 // Make request 00073 req.cmd_sid = UART_CMD_SID_SNIC_INIT_REQ; 00074 req.seq = mUartRequestSeq++; 00075 req.buf_size[0] = 0x08; 00076 req.buf_size[1] = 0x00; 00077 00078 unsigned char *command_array_p = snic_core_p->getCommandBuf(); 00079 unsigned int command_len; 00080 // Preparation of command 00081 command_len = snic_core_p->preparationSendCommand( UART_CMD_ID_SNIC, req.cmd_sid, (unsigned char *)&req 00082 , sizeof(C_SNIC_Core::tagSNIC_INIT_REQ_T), payload_buf_p->buf, command_array_p ); 00083 00084 // Send uart command request 00085 snic_core_p->sendUart( command_len, command_array_p ); 00086 00087 int ret; 00088 // Wait UART response 00089 ret = uartCmdMgr_p->wait(); 00090 if( ret != 0 ) 00091 { 00092 DEBUG_PRINT( "snic_init failed\r\n" ); 00093 snic_core_p->freeCmdBuf( payload_buf_p ); 00094 return -1; 00095 } 00096 00097 if( uartCmdMgr_p->getCommandStatus() != 0 ) 00098 { 00099 DEBUG_PRINT("snic_init status:%02x\r\n", uartCmdMgr_p->getCommandStatus()); 00100 snic_core_p->freeCmdBuf( payload_buf_p ); 00101 return -1; 00102 } 00103 snic_core_p->freeCmdBuf( payload_buf_p ); 00104 00105 return ret; 00106 } 00107 00108 int C_SNIC_WifiInterface::getFWVersion( unsigned char *version_p ) 00109 { 00110 C_SNIC_Core *snic_core_p = C_SNIC_Core::getInstance(); 00111 C_SNIC_UartCommandManager *uartCmdMgr_p = snic_core_p->getUartCommand(); 00112 00113 // Get buffer for response payload from MemoryPool 00114 tagMEMPOOL_BLOCK_T *payload_buf_p = snic_core_p->allocCmdBuf(); 00115 if( payload_buf_p == NULL ) 00116 { 00117 DEBUG_PRINT("getFWVersion payload_buf_p NULL\r\n"); 00118 return -1; 00119 } 00120 00121 C_SNIC_Core::tagGEN_FW_VER_GET_REQ_T req; 00122 // Make request 00123 req.cmd_sid = UART_CMD_SID_GEN_FW_VER_GET_REQ; 00124 req.seq = mUartRequestSeq++; 00125 00126 unsigned char *command_array_p = snic_core_p->getCommandBuf(); 00127 unsigned int command_len; 00128 // Preparation of command 00129 command_len = snic_core_p->preparationSendCommand( UART_CMD_ID_GEN, req.cmd_sid, (unsigned char *)&req 00130 , sizeof(C_SNIC_Core::tagGEN_FW_VER_GET_REQ_T), payload_buf_p->buf, command_array_p ); 00131 00132 int ret; 00133 00134 // Send uart command request 00135 snic_core_p->sendUart( command_len, command_array_p ); 00136 00137 // Wait UART response 00138 ret = uartCmdMgr_p->wait(); 00139 if( ret != 0 ) 00140 { 00141 DEBUG_PRINT( "getFWversion failed\r\n" ); 00142 snic_core_p->freeCmdBuf( payload_buf_p ); 00143 return -1; 00144 } 00145 00146 if( uartCmdMgr_p->getCommandStatus() == 0 ) 00147 { 00148 unsigned char version_len = payload_buf_p->buf[3]; 00149 memcpy( version_p, &payload_buf_p->buf[4], version_len ); 00150 } 00151 snic_core_p->freeCmdBuf( payload_buf_p ); 00152 return 0; 00153 } 00154 00155 int C_SNIC_WifiInterface::connect(const char *ssid_p, unsigned char ssid_len, E_SECURITY sec_type 00156 , const char *sec_key_p, unsigned char sec_key_len) 00157 { 00158 C_SNIC_Core *snic_core_p = C_SNIC_Core::getInstance(); 00159 C_SNIC_UartCommandManager *uartCmdMgr_p = snic_core_p->getUartCommand(); 00160 00161 // Parameter check(SSID) 00162 if( (ssid_p == NULL) || (ssid_len == 0) ) 00163 { 00164 DEBUG_PRINT( "connect failed [ parameter NG:SSID ]\r\n" ); 00165 return -1; 00166 } 00167 00168 // Parameter check(Security key) 00169 if( (sec_type != e_SEC_OPEN) && ( (sec_key_len == 0) || (sec_key_p == NULL) ) ) 00170 { 00171 DEBUG_PRINT( "connect failed [ parameter NG:Security key ]\r\n" ); 00172 return -1; 00173 } 00174 00175 // Get buffer for response payload from MemoryPool 00176 tagMEMPOOL_BLOCK_T *payload_buf_p = snic_core_p->allocCmdBuf(); 00177 if( payload_buf_p == NULL ) 00178 { 00179 DEBUG_PRINT("connect payload_buf_p NULL\r\n"); 00180 return -1; 00181 } 00182 00183 unsigned char *buf = &gCONNECT_BUF[0]; 00184 unsigned int buf_len = 0; 00185 unsigned int command_len; 00186 00187 memset( buf, 0, UART_CONNECT_BUF_SIZE ); 00188 // Make request 00189 buf[0] = UART_CMD_SID_WIFI_JOIN_REQ; 00190 buf_len++; 00191 buf[1] = mUartRequestSeq++; 00192 buf_len++; 00193 // SSID 00194 memcpy( &buf[2], ssid_p, ssid_len ); 00195 buf_len += ssid_len; 00196 buf_len++; 00197 00198 // Security mode 00199 buf[ buf_len ] = (unsigned char)sec_type; 00200 buf_len++; 00201 00202 // Security key 00203 if( sec_type != e_SEC_OPEN ) 00204 { 00205 buf[ buf_len ] = sec_key_len; 00206 buf_len++; 00207 if( sec_key_len > 0 ) 00208 { 00209 memcpy( &buf[buf_len], sec_key_p, sec_key_len ); 00210 buf_len += sec_key_len; 00211 } 00212 } 00213 00214 unsigned char *command_array_p = snic_core_p->getCommandBuf(); 00215 // Preparation of command 00216 command_len = snic_core_p->preparationSendCommand( UART_CMD_ID_WIFI, UART_CMD_SID_WIFI_JOIN_REQ, buf 00217 , buf_len, payload_buf_p->buf, command_array_p ); 00218 00219 // Send uart command request 00220 snic_core_p->sendUart( command_len, command_array_p ); 00221 00222 int ret; 00223 // Wait UART response 00224 ret = uartCmdMgr_p->wait(); 00225 if(uartCmdMgr_p->getCommandStatus() != UART_CMD_RES_WIFI_ERR_ALREADY_JOINED) 00226 { 00227 DEBUG_PRINT( "Already connected\r\n" ); 00228 } 00229 else 00230 { 00231 if( ret != 0 ) 00232 { 00233 DEBUG_PRINT( "join failed\r\n" ); 00234 snic_core_p->freeCmdBuf( payload_buf_p ); 00235 return -1; 00236 } 00237 } 00238 00239 if(uartCmdMgr_p->getCommandStatus() != 0) 00240 { 00241 DEBUG_PRINT("join status:%02x\r\n", uartCmdMgr_p->getCommandStatus()); 00242 snic_core_p->freeCmdBuf( payload_buf_p ); 00243 return -1; 00244 } 00245 snic_core_p->freeCmdBuf( payload_buf_p ); 00246 00247 return ret; 00248 } 00249 00250 int C_SNIC_WifiInterface::disconnect() 00251 { 00252 C_SNIC_Core *snic_core_p = C_SNIC_Core::getInstance(); 00253 C_SNIC_UartCommandManager *uartCmdMgr_p = snic_core_p->getUartCommand(); 00254 00255 // Get buffer for response payload from MemoryPool 00256 tagMEMPOOL_BLOCK_T *payload_buf_p = snic_core_p->allocCmdBuf(); 00257 if( payload_buf_p == NULL ) 00258 { 00259 DEBUG_PRINT("disconnect payload_buf_p NULL\r\n"); 00260 return -1; 00261 } 00262 00263 C_SNIC_Core::tagWIFI_DISCONNECT_REQ_T req; 00264 // Make request 00265 req.cmd_sid = UART_CMD_SID_WIFI_DISCONNECT_REQ; 00266 req.seq = mUartRequestSeq++; 00267 00268 unsigned char *command_array_p = snic_core_p->getCommandBuf(); 00269 unsigned int command_len; 00270 // Preparation of command 00271 command_len = snic_core_p->preparationSendCommand( UART_CMD_ID_WIFI, req.cmd_sid, (unsigned char *)&req 00272 , sizeof(C_SNIC_Core::tagWIFI_DISCONNECT_REQ_T), payload_buf_p->buf, command_array_p ); 00273 00274 // Send uart command request 00275 snic_core_p->sendUart( command_len, command_array_p ); 00276 00277 int ret; 00278 // Wait UART response 00279 ret = uartCmdMgr_p->wait(); 00280 if( ret != 0 ) 00281 { 00282 DEBUG_PRINT( "disconnect failed\r\n" ); 00283 snic_core_p->freeCmdBuf( payload_buf_p ); 00284 return -1; 00285 } 00286 00287 if( uartCmdMgr_p->getCommandStatus() != 0 ) 00288 { 00289 DEBUG_PRINT("disconnect status:%02x\r\n", uartCmdMgr_p->getCommandStatus()); 00290 ret = -1; 00291 } 00292 snic_core_p->freeCmdBuf( payload_buf_p ); 00293 return ret; 00294 } 00295 00296 int C_SNIC_WifiInterface::scan( const char *ssid_p, unsigned char *bssid_p 00297 , void (*result_handler_p)(tagSCAN_RESULT_T *scan_result) ) 00298 { 00299 C_SNIC_Core *snic_core_p = C_SNIC_Core::getInstance(); 00300 C_SNIC_UartCommandManager *uartCmdMgr_p = snic_core_p->getUartCommand(); 00301 00302 // Get buffer for response payload from MemoryPool 00303 tagMEMPOOL_BLOCK_T *payload_buf_p = snic_core_p->allocCmdBuf(); 00304 if( payload_buf_p == NULL ) 00305 { 00306 DEBUG_PRINT("scan payload_buf_p NULL\r\n"); 00307 return -1; 00308 } 00309 00310 C_SNIC_Core::tagWIFI_SCAN_REQ_T req; 00311 unsigned int buf_len = 0; 00312 00313 memset( &req, 0, sizeof(C_SNIC_Core::tagWIFI_SCAN_REQ_T) ); 00314 // Make request 00315 req.cmd_sid = UART_CMD_SID_WIFI_SCAN_REQ; 00316 buf_len++; 00317 req.seq = mUartRequestSeq++; 00318 buf_len++; 00319 00320 // Set scan type(Active scan) 00321 req.scan_type = 0; 00322 buf_len++; 00323 // Set bss type(any) 00324 req.bss_type = 2; 00325 buf_len++; 00326 // Set BSSID 00327 if( bssid_p != NULL ) 00328 { 00329 memcpy( req.bssid, bssid_p, BSSID_MAC_LENTH ); 00330 } 00331 buf_len += BSSID_MAC_LENTH; 00332 // Set channel list(0) 00333 req.chan_list = 0; 00334 buf_len++; 00335 //Set SSID 00336 if( ssid_p != NULL ) 00337 { 00338 strcpy( (char *)req.ssid, ssid_p ); 00339 buf_len += strlen(ssid_p); 00340 } 00341 buf_len++; 00342 00343 unsigned char *command_array_p = snic_core_p->getCommandBuf(); 00344 unsigned int command_len; 00345 // Preparation of command 00346 command_len = snic_core_p->preparationSendCommand( UART_CMD_ID_WIFI, req.cmd_sid, (unsigned char *)&req 00347 , buf_len, payload_buf_p->buf, command_array_p ); 00348 00349 // Set scan result callback 00350 uartCmdMgr_p->setScanResultHandler( result_handler_p ); 00351 00352 // Send uart command request 00353 snic_core_p->sendUart( command_len, command_array_p ); 00354 00355 int ret; 00356 // Wait UART response 00357 ret = uartCmdMgr_p->wait(); 00358 DEBUG_PRINT( "scan wait:%d\r\n", ret ); 00359 if( ret != 0 ) 00360 { 00361 DEBUG_PRINT( "scan failed\r\n" ); 00362 snic_core_p->freeCmdBuf( payload_buf_p ); 00363 return -1; 00364 } 00365 00366 if( uartCmdMgr_p->getCommandStatus() != 0 ) 00367 { 00368 DEBUG_PRINT("scan status:%02x\r\n", uartCmdMgr_p->getCommandStatus()); 00369 snic_core_p->freeCmdBuf( payload_buf_p ); 00370 return -1; 00371 } 00372 00373 snic_core_p->freeCmdBuf( payload_buf_p ); 00374 00375 return ret; 00376 } 00377 00378 int C_SNIC_WifiInterface::wifi_on( const char *country_p ) 00379 { 00380 C_SNIC_Core *snic_core_p = C_SNIC_Core::getInstance(); 00381 C_SNIC_UartCommandManager *uartCmdMgr_p = snic_core_p->getUartCommand(); 00382 00383 // Parameter check 00384 if( country_p == NULL ) 00385 { 00386 DEBUG_PRINT("wifi_on parameter error\r\n"); 00387 return -1; 00388 } 00389 00390 // Get buffer for response payload from MemoryPool 00391 tagMEMPOOL_BLOCK_T *payload_buf_p = snic_core_p->allocCmdBuf(); 00392 if( payload_buf_p == NULL ) 00393 { 00394 DEBUG_PRINT("wifi_on payload_buf_p NULL\r\n"); 00395 return -1; 00396 } 00397 00398 C_SNIC_Core::tagWIFI_ON_REQ_T req; 00399 // Make request 00400 req.cmd_sid = UART_CMD_SID_WIFI_ON_REQ; 00401 req.seq = mUartRequestSeq++; 00402 memcpy( req.country, country_p, COUNTRYC_CODE_LENTH ); 00403 00404 unsigned char *command_array_p = snic_core_p->getCommandBuf(); 00405 unsigned int command_len; 00406 // Preparation of command 00407 command_len = snic_core_p->preparationSendCommand( UART_CMD_ID_WIFI, req.cmd_sid, (unsigned char *)&req 00408 , sizeof(C_SNIC_Core::tagWIFI_ON_REQ_T), payload_buf_p->buf, command_array_p ); 00409 00410 // Send uart command request 00411 snic_core_p->sendUart( command_len, command_array_p ); 00412 00413 int ret; 00414 // Wait UART response 00415 ret = uartCmdMgr_p->wait(); 00416 if( ret != 0 ) 00417 { 00418 DEBUG_PRINT( "wifi_on failed\r\n" ); 00419 snic_core_p->freeCmdBuf( payload_buf_p ); 00420 return -1; 00421 } 00422 00423 if( uartCmdMgr_p->getCommandStatus() != 0 ) 00424 { 00425 DEBUG_PRINT("wifi_on status:%02x\r\n", uartCmdMgr_p->getCommandStatus()); 00426 snic_core_p->freeCmdBuf( payload_buf_p ); 00427 return -1; 00428 } 00429 snic_core_p->freeCmdBuf( payload_buf_p ); 00430 00431 return ret; 00432 } 00433 00434 int C_SNIC_WifiInterface::wifi_off() 00435 { 00436 C_SNIC_Core *snic_core_p = C_SNIC_Core::getInstance(); 00437 C_SNIC_UartCommandManager *uartCmdMgr_p = snic_core_p->getUartCommand(); 00438 00439 // Get buffer for response payload from MemoryPool 00440 tagMEMPOOL_BLOCK_T *payload_buf_p = snic_core_p->allocCmdBuf(); 00441 if( payload_buf_p == NULL ) 00442 { 00443 DEBUG_PRINT("wifi_off payload_buf_p NULL\r\n"); 00444 return -1; 00445 } 00446 00447 C_SNIC_Core::tagWIFI_OFF_REQ_T req; 00448 // Make request 00449 req.cmd_sid = UART_CMD_SID_WIFI_OFF_REQ; 00450 req.seq = mUartRequestSeq++; 00451 00452 unsigned char *command_array_p = snic_core_p->getCommandBuf(); 00453 unsigned int command_len; 00454 // Preparation of command 00455 command_len = snic_core_p->preparationSendCommand( UART_CMD_ID_WIFI, req.cmd_sid, (unsigned char *)&req 00456 , sizeof(C_SNIC_Core::tagWIFI_OFF_REQ_T), payload_buf_p->buf, command_array_p ); 00457 00458 // Send uart command request 00459 snic_core_p->sendUart( command_len, command_array_p ); 00460 00461 int ret; 00462 // Wait UART response 00463 ret = uartCmdMgr_p->wait(); 00464 if( ret != 0 ) 00465 { 00466 DEBUG_PRINT( "wifi_off failed\r\n" ); 00467 snic_core_p->freeCmdBuf( payload_buf_p ); 00468 return -1; 00469 } 00470 00471 if( uartCmdMgr_p->getCommandStatus() != 0 ) 00472 { 00473 DEBUG_PRINT("wifi_off status:%02x\r\n", uartCmdMgr_p->getCommandStatus()); 00474 snic_core_p->freeCmdBuf( payload_buf_p ); 00475 return -1; 00476 } 00477 snic_core_p->freeCmdBuf( payload_buf_p ); 00478 00479 return ret; 00480 } 00481 00482 int C_SNIC_WifiInterface::getRssi( signed char *rssi_p ) 00483 { 00484 C_SNIC_Core *snic_core_p = C_SNIC_Core::getInstance(); 00485 C_SNIC_UartCommandManager *uartCmdMgr_p = snic_core_p->getUartCommand(); 00486 if( rssi_p == NULL ) 00487 { 00488 DEBUG_PRINT("getRssi parameter error\r\n"); 00489 return -1; 00490 } 00491 00492 // Get buffer for response payload from MemoryPool 00493 tagMEMPOOL_BLOCK_T *payload_buf_p = snic_core_p->allocCmdBuf(); 00494 if( payload_buf_p == NULL ) 00495 { 00496 DEBUG_PRINT("getRssi payload_buf_p NULL\r\n"); 00497 return -1; 00498 } 00499 00500 C_SNIC_Core::tagWIFI_GET_STA_RSSI_REQ_T req; 00501 00502 // Make request 00503 req.cmd_sid = UART_CMD_SID_WIFI_GET_STA_RSSI_REQ; 00504 req.seq = mUartRequestSeq++; 00505 00506 unsigned char *command_array_p = snic_core_p->getCommandBuf(); 00507 unsigned int command_len; 00508 command_len = snic_core_p->preparationSendCommand( UART_CMD_ID_WIFI, req.cmd_sid, (unsigned char *)&req 00509 , sizeof(C_SNIC_Core::tagWIFI_GET_STA_RSSI_REQ_T), payload_buf_p->buf, command_array_p ); 00510 00511 int ret; 00512 // Send uart command request 00513 snic_core_p->sendUart( command_len, command_array_p ); 00514 00515 // Wait UART response 00516 ret = uartCmdMgr_p->wait(); 00517 if( ret != 0 ) 00518 { 00519 DEBUG_PRINT( "getRssi failed\r\n" ); 00520 snic_core_p->freeCmdBuf( payload_buf_p ); 00521 return -1; 00522 } 00523 00524 *rssi_p = (signed char)payload_buf_p->buf[2]; 00525 00526 snic_core_p->freeCmdBuf( payload_buf_p ); 00527 return 0; 00528 } 00529 00530 int C_SNIC_WifiInterface::getWifiStatus( tagWIFI_STATUS_T *status_p) 00531 { 00532 C_SNIC_Core *snic_core_p = C_SNIC_Core::getInstance(); 00533 C_SNIC_UartCommandManager *uartCmdMgr_p = snic_core_p->getUartCommand(); 00534 00535 if( status_p == NULL ) 00536 { 00537 DEBUG_PRINT("getWifiStatus parameter error\r\n"); 00538 return -1; 00539 } 00540 00541 // Get buffer for response payload from MemoryPool 00542 tagMEMPOOL_BLOCK_T *payload_buf_p = snic_core_p->allocCmdBuf(); 00543 if( payload_buf_p == NULL ) 00544 { 00545 DEBUG_PRINT("getWifiStatus payload_buf_p NULL\r\n"); 00546 return -1; 00547 } 00548 00549 C_SNIC_Core::tagWIFI_GET_STATUS_REQ_T req; 00550 // Make request 00551 req.cmd_sid = UART_CMD_SID_WIFI_GET_STATUS_REQ; 00552 req.seq = mUartRequestSeq++; 00553 req.interface = 0; 00554 00555 unsigned char *command_array_p = snic_core_p->getCommandBuf(); 00556 unsigned int command_len; 00557 command_len = snic_core_p->preparationSendCommand( UART_CMD_ID_WIFI, req.cmd_sid, (unsigned char *)&req 00558 , sizeof(C_SNIC_Core::tagWIFI_GET_STATUS_REQ_T), payload_buf_p->buf, command_array_p ); 00559 00560 // Send uart command request 00561 snic_core_p->sendUart( command_len, command_array_p ); 00562 00563 int ret; 00564 // Wait UART response 00565 ret = uartCmdMgr_p->wait(); 00566 if( ret != 0 ) 00567 { 00568 DEBUG_PRINT( "getWifiStatus failed\r\n" ); 00569 snic_core_p->freeCmdBuf( payload_buf_p ); 00570 return -1; 00571 } 00572 00573 // set status 00574 status_p->status = (E_WIFI_STATUS)payload_buf_p->buf[2]; 00575 00576 // set Mac address 00577 if( status_p->status != e_STATUS_OFF ) 00578 { 00579 memcpy( status_p->mac_address, &payload_buf_p->buf[3], BSSID_MAC_LENTH ); 00580 } 00581 00582 // set SSID 00583 if( ( status_p->status == e_STA_JOINED ) || ( status_p->status == e_AP_STARTED ) ) 00584 { 00585 memcpy( status_p->ssid, &payload_buf_p->buf[9], strlen( (char *)&payload_buf_p->buf[9]) ); 00586 } 00587 00588 snic_core_p->freeCmdBuf( payload_buf_p ); 00589 return 0; 00590 } 00591 00592 int C_SNIC_WifiInterface::setIPConfig( bool is_DHCP 00593 , const char *ip_p, const char *mask_p, const char *gateway_p ) 00594 { 00595 // Parameter check 00596 if( is_DHCP == false ) 00597 { 00598 if( (ip_p == NULL) || (mask_p == NULL) ||(gateway_p == NULL) ) 00599 { 00600 DEBUG_PRINT("setIPConfig parameter error\r\n"); 00601 return -1; 00602 } 00603 } 00604 00605 C_SNIC_Core *snic_core_p = C_SNIC_Core::getInstance(); 00606 C_SNIC_UartCommandManager *uartCmdMgr_p = snic_core_p->getUartCommand(); 00607 00608 // Get buffer for response payload from MemoryPool 00609 tagMEMPOOL_BLOCK_T *payload_buf_p = snic_core_p->allocCmdBuf(); 00610 if( payload_buf_p == NULL ) 00611 { 00612 DEBUG_PRINT("setIPConfig payload_buf_p NULL\r\n"); 00613 return -1; 00614 } 00615 00616 unsigned char *command_array_p = snic_core_p->getCommandBuf(); 00617 unsigned int command_len; 00618 if( is_DHCP == true ) 00619 { 00620 C_SNIC_Core::tagSNIC_IP_CONFIG_REQ_DHCP_T req; 00621 // Make request 00622 req.cmd_sid = UART_CMD_SID_SNIC_IP_CONFIG_REQ; 00623 req.seq = mUartRequestSeq++; 00624 req.interface = 0; 00625 req.dhcp = 1; 00626 00627 // Preparation of command 00628 command_len = snic_core_p->preparationSendCommand( UART_CMD_ID_SNIC, req.cmd_sid, (unsigned char *)&req 00629 , sizeof(C_SNIC_Core::tagSNIC_IP_CONFIG_REQ_DHCP_T), payload_buf_p->buf, command_array_p ); 00630 } 00631 else 00632 { 00633 C_SNIC_Core::tagSNIC_IP_CONFIG_REQ_STATIC_T req; 00634 // Make request 00635 req.cmd_sid = UART_CMD_SID_SNIC_IP_CONFIG_REQ; 00636 req.seq = mUartRequestSeq++; 00637 req.interface = 0; 00638 req.dhcp = 0; 00639 00640 // Set paramter of address 00641 int addr_temp; 00642 addr_temp = C_SNIC_UartMsgUtil::addrToInteger( ip_p ); 00643 C_SNIC_UartMsgUtil::convertIntToByteAdday( addr_temp, (char *)req.ip_addr ); 00644 addr_temp = C_SNIC_UartMsgUtil::addrToInteger( mask_p ); 00645 C_SNIC_UartMsgUtil::convertIntToByteAdday( addr_temp, (char *)req.netmask ); 00646 addr_temp = C_SNIC_UartMsgUtil::addrToInteger( gateway_p ); 00647 C_SNIC_UartMsgUtil::convertIntToByteAdday( addr_temp, (char *)req.gateway ); 00648 00649 // Preparation of command 00650 command_len = snic_core_p->preparationSendCommand( UART_CMD_ID_SNIC, req.cmd_sid, (unsigned char *)&req 00651 , sizeof(C_SNIC_Core::tagSNIC_IP_CONFIG_REQ_STATIC_T), payload_buf_p->buf, command_array_p ); 00652 } 00653 // Send uart command request 00654 snic_core_p->sendUart( command_len, command_array_p ); 00655 00656 int ret; 00657 // Wait UART response 00658 ret = uartCmdMgr_p->wait(); 00659 if( ret != 0 ) 00660 { 00661 DEBUG_PRINT( "setIPConfig failed\r\n" ); 00662 snic_core_p->freeCmdBuf( payload_buf_p ); 00663 return -1; 00664 } 00665 00666 if( uartCmdMgr_p->getCommandStatus() != 0 ) 00667 { 00668 DEBUG_PRINT("setIPConfig status:%02x\r\n", uartCmdMgr_p->getCommandStatus()); 00669 snic_core_p->freeCmdBuf( payload_buf_p ); 00670 return -1; 00671 } 00672 00673 snic_core_p->freeCmdBuf( payload_buf_p ); 00674 return ret; 00675 } 00676 00677 char* C_SNIC_WifiInterface::getIPAddress() { 00678 C_SNIC_Core *snic_core_p = C_SNIC_Core::getInstance(); 00679 C_SNIC_UartCommandManager *uartCmdMgr_p = snic_core_p->getUartCommand(); 00680 00681 snic_core_p->lockAPI(); 00682 // Get local ip address. 00683 // Get buffer for response payload from MemoryPool 00684 tagMEMPOOL_BLOCK_T *payload_buf_p = snic_core_p->allocCmdBuf(); 00685 if( payload_buf_p == NULL ) 00686 { 00687 DEBUG_PRINT("getIPAddress payload_buf_p NULL\r\n"); 00688 snic_core_p->unlockAPI(); 00689 return 0; 00690 } 00691 00692 C_SNIC_Core::tagSNIC_GET_DHCP_INFO_REQ_T req; 00693 // Make request 00694 req.cmd_sid = UART_CMD_SID_SNIC_GET_DHCP_INFO_REQ; 00695 req.seq = mUartRequestSeq++; 00696 req.interface = 0; 00697 00698 unsigned char *command_array_p = snic_core_p->getCommandBuf(); 00699 unsigned int command_len; 00700 // Preparation of command 00701 command_len = snic_core_p->preparationSendCommand( UART_CMD_ID_SNIC, req.cmd_sid, (unsigned char *)&req 00702 , sizeof(C_SNIC_Core::tagSNIC_GET_DHCP_INFO_REQ_T), payload_buf_p->buf, command_array_p ); 00703 // Send uart command request 00704 snic_core_p->sendUart( command_len, command_array_p ); 00705 // Wait UART response 00706 int ret = uartCmdMgr_p->wait(); 00707 if( ret != 0 ) 00708 { 00709 DEBUG_PRINT( "getIPAddress failed\r\n" ); 00710 snic_core_p->freeCmdBuf( payload_buf_p ); 00711 snic_core_p->unlockAPI(); 00712 return 0; 00713 } 00714 00715 if( uartCmdMgr_p->getCommandStatus() != UART_CMD_RES_SNIC_SUCCESS ) 00716 { 00717 snic_core_p->freeCmdBuf( payload_buf_p ); 00718 snic_core_p->unlockAPI(); 00719 return 0; 00720 } 00721 00722 snic_core_p->freeCmdBuf( payload_buf_p ); 00723 snic_core_p->unlockAPI(); 00724 00725 sprintf(ip_addr, "%d.%d.%d.%d\0", payload_buf_p->buf[9], payload_buf_p->buf[10], payload_buf_p->buf[11], payload_buf_p->buf[12]); 00726 00727 return ip_addr; 00728 }
Generated on Thu Jul 14 2022 10:07:54 by
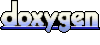