
Demo application of SNICInterface library for Murata TypeYD, which reports sensor data periodically to Xively cloud server . Hardware platform: mbed application board (https://mbed.org/cookbook/mbed-application-board), mbed LPC1768 (https://mbed.org/platforms/mbed-LPC1768/) and TypeYD.
Dependencies: C12832 LM75B MMA7660 SNICInterface libxively mbed-rtos mbed
PowerControl.h
00001 /* mbed PowerControl Library 00002 * Copyright (c) 2010 Michael Wei 00003 */ 00004 00005 #ifndef MBED_POWERCONTROL_H 00006 #define MBED_POWERCONTROL_H 00007 00008 //shouldn't have to include, but fixes weird problems with defines 00009 //#include "LPC1768/LPC17xx.h" 00010 #include "mbed.h" 00011 00012 //System Control Register 00013 // bit 0: Reserved 00014 // bit 1: Sleep on Exit 00015 #define LPC1768_SCR_SLEEPONEXIT 0x2 00016 // bit 2: Deep Sleep 00017 #define LPC1768_SCR_SLEEPDEEP 0x4 00018 // bit 3: Resereved 00019 // bit 4: Send on Pending 00020 #define LPC1768_SCR_SEVONPEND 0x10 00021 // bit 5-31: Reserved 00022 00023 //Power Control Register 00024 // bit 0: Power mode control bit 0 (power-down mode) 00025 #define LPC1768_PCON_PM0 0x1 00026 // bit 1: Power mode control bit 1 (deep power-down mode) 00027 #define LPC1768_PCON_PM1 0x2 00028 // bit 2: Brown-out reduced power mode 00029 #define LPC1768_PCON_BODRPM 0x4 00030 // bit 3: Brown-out global disable 00031 #define LPC1768_PCON_BOGD 0x8 00032 // bit 4: Brown-out reset disable 00033 #define LPC1768_PCON_BORD 0x10 00034 // bit 5-7 : Reserved 00035 // bit 8: Sleep Mode Entry Flag 00036 #define LPC1768_PCON_SMFLAG 0x100 00037 // bit 9: Deep Sleep Entry Flag 00038 #define LPC1768_PCON_DSFLAG 0x200 00039 // bit 10: Power Down Entry Flag 00040 #define LPC1768_PCON_PDFLAG 0x400 00041 // bit 11: Deep Power Down Entry Flag 00042 #define LPC1768_PCON_DPDFLAG 0x800 00043 // bit 12-31: Reserved 00044 00045 //"Sleep Mode" (WFI). 00046 inline void Sleep(void) 00047 { 00048 __WFI(); 00049 } 00050 00051 //"Deep Sleep" Mode 00052 inline void DeepSleep(void) 00053 { 00054 SCB->SCR |= LPC1768_SCR_SLEEPDEEP; 00055 __WFI(); 00056 } 00057 00058 //"Power-Down" Mode 00059 inline void PowerDown(void) 00060 { 00061 SCB->SCR |= LPC1768_SCR_SLEEPDEEP; 00062 LPC_SC->PCON &= ~LPC1768_PCON_PM1; 00063 LPC_SC->PCON |= LPC1768_PCON_PM0; 00064 __WFI(); 00065 //reset back to normal 00066 LPC_SC->PCON &= ~(LPC1768_PCON_PM1 | LPC1768_PCON_PM0); 00067 } 00068 00069 //"Deep Power-Down" Mode 00070 inline void DeepPowerDown(void) 00071 { 00072 SCB->SCR |= LPC1768_SCR_SLEEPDEEP; 00073 LPC_SC->PCON |= LPC1768_PCON_PM1 | LPC1768_PCON_PM0; 00074 __WFI(); 00075 //reset back to normal 00076 LPC_SC->PCON &= ~(LPC1768_PCON_PM1 | LPC1768_PCON_PM0); 00077 } 00078 00079 //shut down BOD during power-down/deep sleep 00080 inline void BrownOut_ReducedPowerMode_Enable(void) 00081 { 00082 LPC_SC->PCON |= LPC1768_PCON_BODRPM; 00083 } 00084 00085 //turn on BOD during power-down/deep sleep 00086 inline void BrownOut_ReducedPowerMode_Disable(void) 00087 { 00088 LPC_SC->PCON &= ~LPC1768_PCON_BODRPM; 00089 } 00090 00091 //turn off brown out circutry 00092 inline void BrownOut_Global_Disable(void) 00093 { 00094 LPC_SC->PCON |= LPC1768_PCON_BOGD; 00095 } 00096 00097 //turn on brown out circutry 00098 inline void BrownOut_Global_Enable(void) 00099 { 00100 LPC_SC->PCON &= !LPC1768_PCON_BOGD; 00101 } 00102 00103 //turn off brown out reset circutry 00104 inline void BrownOut_Reset_Disable(void) 00105 { 00106 LPC_SC->PCON |= LPC1768_PCON_BORD; 00107 } 00108 00109 //turn on brown outreset circutry 00110 inline void BrownOut_Reset_Enable(void) 00111 { 00112 LPC_SC->PCON &= ~LPC1768_PCON_BORD; 00113 } 00114 //Peripheral Control Register 00115 // bit 0: Reserved 00116 // bit 1: PCTIM0: Timer/Counter 0 power/clock enable 00117 #define LPC1768_PCONP_PCTIM0 0x2 00118 // bit 2: PCTIM1: Timer/Counter 1 power/clock enable 00119 #define LPC1768_PCONP_PCTIM1 0x4 00120 // bit 3: PCUART0: UART 0 power/clock enable 00121 #define LPC1768_PCONP_PCUART0 0x8 00122 // bit 4: PCUART1: UART 1 power/clock enable 00123 #define LPC1768_PCONP_PCUART1 0x10 00124 // bit 5: Reserved 00125 // bit 6: PCPWM1: PWM 1 power/clock enable 00126 #define LPC1768_PCONP_PCPWM1 0x40 00127 // bit 7: PCI2C0: I2C interface 0 power/clock enable 00128 #define LPC1768_PCONP_PCI2C0 0x80 00129 // bit 8: PCSPI: SPI interface power/clock enable 00130 #define LPC1768_PCONP_PCSPI 0x100 00131 // bit 9: PCRTC: RTC power/clock enable 00132 #define LPC1768_PCONP_PCRTC 0x200 00133 // bit 10: PCSSP1: SSP interface 1 power/clock enable 00134 #define LPC1768_PCONP_PCSSP1 0x400 00135 // bit 11: Reserved 00136 // bit 12: PCADC: A/D converter power/clock enable 00137 #define LPC1768_PCONP_PCADC 0x1000 00138 // bit 13: PCCAN1: CAN controller 1 power/clock enable 00139 #define LPC1768_PCONP_PCCAN1 0x2000 00140 // bit 14: PCCAN2: CAN controller 2 power/clock enable 00141 #define LPC1768_PCONP_PCCAN2 0x4000 00142 // bit 15: PCGPIO: GPIOs power/clock enable 00143 #define LPC1768_PCONP_PCGPIO 0x8000 00144 // bit 16: PCRIT: Repetitive interrupt timer power/clock enable 00145 #define LPC1768_PCONP_PCRIT 0x10000 00146 // bit 17: PCMCPWM: Motor control PWM power/clock enable 00147 #define LPC1768_PCONP_PCMCPWM 0x20000 00148 // bit 18: PCQEI: Quadrature encoder interface power/clock enable 00149 #define LPC1768_PCONP_PCQEI 0x40000 00150 // bit 19: PCI2C1: I2C interface 1 power/clock enable 00151 #define LPC1768_PCONP_PCI2C1 0x80000 00152 // bit 20: Reserved 00153 // bit 21: PCSSP0: SSP interface 0 power/clock enable 00154 #define LPC1768_PCONP_PCSSP0 0x200000 00155 // bit 22: PCTIM2: Timer 2 power/clock enable 00156 #define LPC1768_PCONP_PCTIM2 0x400000 00157 // bit 23: PCTIM3: Timer 3 power/clock enable 00158 #define LPC1768_PCONP_PCQTIM3 0x800000 00159 // bit 24: PCUART2: UART 2 power/clock enable 00160 #define LPC1768_PCONP_PCUART2 0x1000000 00161 // bit 25: PCUART3: UART 3 power/clock enable 00162 #define LPC1768_PCONP_PCUART3 0x2000000 00163 // bit 26: PCI2C2: I2C interface 2 power/clock enable 00164 #define LPC1768_PCONP_PCI2C2 0x4000000 00165 // bit 27: PCI2S: I2S interface power/clock enable 00166 #define LPC1768_PCONP_PCI2S 0x8000000 00167 // bit 28: Reserved 00168 // bit 29: PCGPDMA: GP DMA function power/clock enable 00169 #define LPC1768_PCONP_PCGPDMA 0x20000000 00170 // bit 30: PCENET: Ethernet block power/clock enable 00171 #define LPC1768_PCONP_PCENET 0x40000000 00172 // bit 31: PCUSB: USB interface power/clock enable 00173 #define LPC1768_PCONP_PCUSB 0x80000000 00174 00175 //Powers Up specified Peripheral(s) 00176 inline unsigned int Peripheral_PowerUp(unsigned int bitMask) 00177 { 00178 return LPC_SC->PCONP |= bitMask; 00179 } 00180 00181 //Powers Down specified Peripheral(s) 00182 inline unsigned int Peripheral_PowerDown(unsigned int bitMask) 00183 { 00184 return LPC_SC->PCONP &= ~bitMask; 00185 } 00186 00187 //returns if the peripheral is on or off 00188 inline bool Peripheral_GetStatus(unsigned int peripheral) 00189 { 00190 return (LPC_SC->PCONP & peripheral) ? true : false; 00191 } 00192 00193 #endif
Generated on Tue Jul 19 2022 11:25:02 by
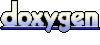