Basic C library for MQTT packet serialization and deserialization
Dependents: MQTT MQTT MQTT MQTT ... more
Fork of MQTTPacket by
MQTTPacket.h
00001 /******************************************************************************* 00002 * Copyright (c) 2014 IBM Corp. 00003 * 00004 * All rights reserved. This program and the accompanying materials 00005 * are made available under the terms of the Eclipse Public License v1.0 00006 * and Eclipse Distribution License v1.0 which accompany this distribution. 00007 * 00008 * The Eclipse Public License is available at 00009 * http://www.eclipse.org/legal/epl-v10.html 00010 * and the Eclipse Distribution License is available at 00011 * http://www.eclipse.org/org/documents/edl-v10.php. 00012 * 00013 * Contributors: 00014 * Ian Craggs - initial API and implementation and/or initial documentation 00015 * Xiang Rong - 442039 Add makefile to Embedded C client 00016 *******************************************************************************/ 00017 00018 #ifndef MQTTPACKET_H_ 00019 #define MQTTPACKET_H_ 00020 00021 #if defined(__cplusplus) /* If this is a C++ compiler, use C linkage */ 00022 extern "C" { 00023 #endif 00024 00025 #if defined(WIN32_DLL) || defined(WIN64_DLL) 00026 #define DLLImport __declspec(dllimport) 00027 #define DLLExport __declspec(dllexport) 00028 #elif defined(LINUX_SO) 00029 #define DLLImport extern 00030 #define DLLExport __attribute__ ((visibility ("default"))) 00031 #else 00032 #define DLLImport 00033 #define DLLExport 00034 #endif 00035 00036 enum errors 00037 { 00038 MQTTPACKET_BUFFER_TOO_SHORT = -2, 00039 MQTTPACKET_READ_ERROR = -1, 00040 MQTTPACKET_READ_COMPLETE 00041 }; 00042 00043 enum msgTypes 00044 { 00045 CONNECT = 1, CONNACK, PUBLISH, PUBACK, PUBREC, PUBREL, 00046 PUBCOMP, SUBSCRIBE, SUBACK, UNSUBSCRIBE, UNSUBACK, 00047 PINGREQ, PINGRESP, DISCONNECT 00048 }; 00049 00050 /** 00051 * Bitfields for the MQTT header byte. 00052 */ 00053 typedef union 00054 { 00055 unsigned char byte; /**< the whole byte */ 00056 #if defined(REVERSED) 00057 struct 00058 { 00059 unsigned int type : 4; /**< message type nibble */ 00060 unsigned int dup : 1; /**< DUP flag bit */ 00061 unsigned int qos : 2; /**< QoS value, 0, 1 or 2 */ 00062 unsigned int retain : 1; /**< retained flag bit */ 00063 } bits; 00064 #else 00065 struct 00066 { 00067 unsigned int retain : 1; /**< retained flag bit */ 00068 unsigned int qos : 2; /**< QoS value, 0, 1 or 2 */ 00069 unsigned int dup : 1; /**< DUP flag bit */ 00070 unsigned int type : 4; /**< message type nibble */ 00071 } bits; 00072 #endif 00073 } MQTTHeader; 00074 00075 typedef struct 00076 { 00077 int len; 00078 char* data; 00079 } MQTTLenString; 00080 00081 typedef struct 00082 { 00083 char* cstring; 00084 MQTTLenString lenstring; 00085 } MQTTString; 00086 00087 #define MQTTString_initializer {NULL, {0, NULL}} 00088 00089 int MQTTstrlen(MQTTString mqttstring); 00090 00091 #include "MQTTConnect.h" 00092 #include "MQTTPublish.h" 00093 #include "MQTTSubscribe.h" 00094 #include "MQTTUnsubscribe.h" 00095 #include "MQTTFormat.h" 00096 00097 DLLExport int MQTTSerialize_ack(unsigned char* buf, int buflen, unsigned char type, unsigned char dup, unsigned short packetid); 00098 DLLExport int MQTTDeserialize_ack(unsigned char* packettype, unsigned char* dup, unsigned short* packetid, unsigned char* buf, int buflen); 00099 00100 int MQTTPacket_len(int rem_len); 00101 DLLExport int MQTTPacket_equals(MQTTString* a, char* b); 00102 00103 DLLExport int MQTTPacket_encode(unsigned char* buf, int length); 00104 int MQTTPacket_decode(int (*getcharfn)(unsigned char*, int), int* value); 00105 int MQTTPacket_decodeBuf(unsigned char* buf, int* value); 00106 00107 int readInt(unsigned char** pptr); 00108 char readChar(unsigned char** pptr); 00109 void writeChar(unsigned char** pptr, char c); 00110 void writeInt(unsigned char** pptr, int anInt); 00111 int readMQTTLenString(MQTTString* mqttstring, unsigned char** pptr, unsigned char* enddata); 00112 void writeCString(unsigned char** pptr, const char* string); 00113 void writeMQTTString(unsigned char** pptr, MQTTString mqttstring); 00114 00115 DLLExport int MQTTPacket_read(unsigned char* buf, int buflen, int (*getfn)(unsigned char*, int)); 00116 00117 typedef struct { 00118 int (*getfn)(void *, unsigned char*, int); /* must return -1 for error, 0 for call again, or the number of bytes read */ 00119 void *sck; /* pointer to whatever the system may use to identify the transport */ 00120 int multiplier; 00121 int rem_len; 00122 int len; 00123 char state; 00124 }MQTTTransport; 00125 00126 int MQTTPacket_readnb(unsigned char* buf, int buflen, MQTTTransport *trp); 00127 00128 #ifdef __cplusplus /* If this is a C++ compiler, use C linkage */ 00129 } 00130 #endif 00131 00132 00133 #endif /* MQTTPACKET_H_ */
Generated on Tue Jul 12 2022 17:50:32 by
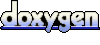