This example shows the use of the 'Pins' (edge connector) and the DAL to make a logic gate simulator from a micro:bit - many of these can be wired together to create logic networks. This is a one-way translation of the microbit-samples repository on GitHub. Please don't try to push changes here, instead push them to the source repo at https://github.com/lancaster-university/microbit-samples
Dependencies: microbit
main.cpp
00001 /* 00002 The MIT License (MIT) 00003 00004 Copyright (c) 2016 British Broadcasting Corporation. 00005 This software is provided by Lancaster University by arrangement with the BBC. 00006 00007 Permission is hereby granted, free of charge, to any person obtaining a 00008 copy of this software and associated documentation files (the "Software"), 00009 to deal in the Software without restriction, including without limitation 00010 the rights to use, copy, modify, merge, publish, distribute, sublicense, 00011 and/or sell copies of the Software, and to permit persons to whom the 00012 Software is furnished to do so, subject to the following conditions: 00013 00014 The above copyright notice and this permission notice shall be included in 00015 all copies or substantial portions of the Software. 00016 00017 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00018 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00019 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL 00020 THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00021 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING 00022 FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER 00023 DEALINGS IN THE SOFTWARE. 00024 */ 00025 00026 #include "MicroBit.h" 00027 00028 #define LOGIC_MODE_NOT 1 00029 #define LOGIC_MODE_AND 2 00030 #define LOGIC_MODE_OR 3 00031 #define LOGIC_MODE_OUTPUT 4 00032 00033 #define LOGIC_MODE_MIN 1 00034 #define LOGIC_MODE_MAX 4 00035 00036 #define TOOL_SELECT_DELAY 1000 00037 00038 MicroBit uBit; 00039 00040 MicroBitImage NOT("\ 00041 0 0 1 0 0\n\ 00042 0 0 1 1 0\n\ 00043 1 1 1 1 1\n\ 00044 0 0 1 1 0\n\ 00045 0 0 1 0 0\n"); 00046 00047 MicroBitImage AND("\ 00048 0 0 1 1 0\n\ 00049 1 1 1 1 1\n\ 00050 0 0 1 1 1\n\ 00051 1 1 1 1 1\n\ 00052 0 0 1 1 0\n"); 00053 00054 MicroBitImage OR("\ 00055 0 0 0 1 0\n\ 00056 1 1 1 1 1\n\ 00057 0 0 0 1 1\n\ 00058 1 1 1 1 1\n\ 00059 0 0 0 1 0\n"); 00060 00061 MicroBitImage OUTPUT_ON("\ 00062 0 1 1 1 0\n\ 00063 1 1 1 1 1\n\ 00064 1 1 1 1 1\n\ 00065 1 1 1 1 1\n\ 00066 0 1 1 1 0\n"); 00067 00068 MicroBitImage OUTPUT_OFF("\ 00069 0 1 1 1 0\n\ 00070 1 0 0 0 1\n\ 00071 1 0 0 0 1\n\ 00072 1 0 0 0 1\n\ 00073 0 1 1 1 0\n"); 00074 00075 int mode = LOGIC_MODE_NOT; 00076 00077 void onShake(MicroBitEvent) 00078 { 00079 // The micro:bit has been shaken, so move on to the next logic gate. 00080 mode++; 00081 00082 // Wrap back to the start if necessary. 00083 if (mode > LOGIC_MODE_MAX) 00084 mode = LOGIC_MODE_MIN; 00085 00086 // Update the display to 00087 switch (mode) 00088 { 00089 case LOGIC_MODE_NOT: 00090 uBit.display.print(NOT); 00091 break; 00092 00093 case LOGIC_MODE_AND: 00094 uBit.display.print(AND); 00095 break; 00096 00097 case LOGIC_MODE_OR: 00098 uBit.display.print(OR); 00099 break; 00100 00101 case LOGIC_MODE_OUTPUT: 00102 uBit.display.print(OUTPUT_OFF); 00103 break; 00104 } 00105 } 00106 00107 int main() 00108 { 00109 // Initialise the micro:bit runtime. 00110 uBit.init(); 00111 00112 // Register to receive events when the micro:bit is shaken. 00113 uBit.messageBus.listen(MICROBIT_ID_GESTURE, MICROBIT_ACCELEROMETER_EVT_SHAKE, onShake); 00114 00115 // 00116 // Create a simple logic gate simulator, using the P0, P1 and P2 pins. 00117 // The micro:bit can then be configured as an NOT / AND / OR gate, by shaking the device. 00118 // 00119 int output = 0; 00120 00121 // Our icons are drawn left to right, so rotate the display so the outputs point at the pins on the edge connector. :-) 00122 uBit.display.rotateTo(MICROBIT_DISPLAY_ROTATION_270); 00123 00124 while (1) 00125 { 00126 // Check inputs and update outputs accordingly. 00127 switch (mode) 00128 { 00129 int o1; 00130 int o2; 00131 00132 case LOGIC_MODE_NOT: 00133 output = uBit.buttonA.isPressed() ? 0 : !uBit.io.P0.getDigitalValue(); 00134 uBit.display.print(NOT); 00135 break; 00136 00137 case LOGIC_MODE_AND: 00138 o1 = uBit.buttonA.isPressed() || uBit.io.P0.getDigitalValue(); 00139 o2 = uBit.buttonB.isPressed() || uBit.io.P1.getDigitalValue(); 00140 output = o1 && o2; 00141 break; 00142 00143 case LOGIC_MODE_OR: 00144 output = uBit.buttonA.isPressed() || uBit.io.P0.getDigitalValue() || uBit.buttonB.isPressed() || uBit.io.P1.getDigitalValue(); 00145 break; 00146 00147 case LOGIC_MODE_OUTPUT: 00148 if (uBit.io.P0.getDigitalValue()) 00149 uBit.display.print(OUTPUT_ON); 00150 else 00151 uBit.display.print(OUTPUT_OFF); 00152 00153 output = 0; 00154 break; 00155 } 00156 00157 // Update output pin value 00158 uBit.io.P2.setDigitalValue(output); 00159 00160 // Perform a power efficient sleep for a little while. No need to run too quickly! 00161 uBit.sleep(1000); 00162 } 00163 } 00164
Generated on Sun Jul 17 2022 01:01:31 by
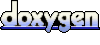