
isr example
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 00003 Ticker ticker; 00004 Thread thread; 00005 Queue<const char*, 5> trail; 00006 00007 // Since we're printing from multiple threads, we need a mutex 00008 Mutex print_lock; 00009 00010 enum ExecutionTypes { 00011 IDLE, 00012 USER, 00013 ISR 00014 }; 00015 00016 const char* ExecutionMessages[] = { 00017 "the idle thread", 00018 "a user thread", 00019 "interrupt context" 00020 }; 00021 00022 void handler() { 00023 // Check to see if we're in interrupt context 00024 if (core_util_is_isr_active()) { 00025 // Do not print since we're in interrupt context 00026 trail.put(&(ExecutionMessages[ISR])); 00027 } else { 00028 // Safe to print since we're in a user thread 00029 print_lock.lock(); 00030 printf("Starting user thread\r\n"); 00031 print_lock.unlock(); 00032 while(true) { 00033 trail.put(&(ExecutionMessages[USER])); 00034 wait(5); 00035 } 00036 } 00037 } 00038 00039 void custom_idle_function() { 00040 // Custom idle behavior would go here 00041 // We won't print here since the default idle thread's stack is too small 00042 trail.put(&(ExecutionMessages[IDLE])); 00043 00044 // Switch back to the default idle behavior 00045 Kernel::attach_idle_hook(NULL); 00046 } 00047 00048 int main() { 00049 printf("Starting execution example\r\n"); 00050 00051 // Attach the custom idle thread function 00052 Kernel::attach_idle_hook(custom_idle_function); 00053 00054 // Trigger the interrupt every 3 seconds 00055 ticker.attach(handler, 3); 00056 00057 // Start the user thread 00058 thread.start(handler); 00059 00060 // Get the past exectuion trail 00061 while (true) { 00062 osEvent evt = trail.get(); 00063 if (evt.status != osEventMessage) { 00064 print_lock.lock(); 00065 printf("Failed to retrieve the execution trail (returned %02x)\r\n", evt.status); 00066 print_lock.unlock(); 00067 } else { 00068 print_lock.lock(); 00069 printf("Execution was in %s\r\n", *(const char**)evt.value.v); 00070 print_lock.unlock(); 00071 } 00072 } 00073 }
Generated on Sat Jul 16 2022 23:34:11 by
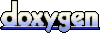