
UserAllocatedEvent class example to manually instantiate, configure and post events onto the static EventQueue.
main.cpp
00001 #include "mbed.h" 00002 00003 00004 // Creates static event queue 00005 static EventQueue queue(0); 00006 void handler(int count); 00007 // Creates an event for later bound 00008 auto event1 = make_user_allocated_event(handler, 1); 00009 auto event2 = make_user_allocated_event(handler, 2); 00010 // Creates an event bound to the specified event queue 00011 auto event3 = queue.make_user_allocated_event(handler, 3); 00012 00013 void handler(int count) { 00014 printf("UserAllocatedEvent = %d \n\r", count); 00015 return; 00016 } 00017 00018 void post_events(void) 00019 { 00020 // Events can be posted multiple times and enqueue gracefully until 00021 // the dispatch function is called. 00022 event1.call_on(&queue); 00023 event2.call_on(&queue); 00024 event3.call(); 00025 } 00026 00027 int main() 00028 { 00029 Thread event_thread; 00030 00031 // The event can be manually configured for special timing requirements 00032 // specified in milliseconds 00033 // Starting delay - 100 msec 00034 // Delay between each event - 200msec 00035 event1.delay(100); 00036 event1.period(200); 00037 event2.delay(100); 00038 event2.period(200); 00039 event3.delay(100); 00040 event3.period(200); 00041 00042 event_thread.start(callback(post_events)); 00043 00044 // Posted events are dispatched in the context of the queue's 00045 // dispatch function 00046 queue.dispatch(400); // Dispatch time - 400msec 00047 // 400 msec - Only 2 set of events will be dispatched as period is 200 msec 00048 00049 event_thread.join(); 00050 }
Generated on Fri Jul 15 2022 05:21:14 by
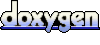