
Demonstration of possible usage of the NFC EEPROM class.
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2018-2018 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "events/EventQueue.h" 00018 00019 #include "nfc/ndef/MessageBuilder.h" 00020 #include "nfc/ndef/common/URI.h" 00021 #include "nfc/ndef/common/util.h" 00022 00023 #include "NFCEEPROM.h" 00024 00025 #include "EEPROMDriver.h" 00026 00027 using events::EventQueue; 00028 00029 using mbed::nfc::NFCEEPROM; 00030 using mbed::nfc::NFCEEPROMDriver; 00031 using mbed::Span; 00032 00033 using mbed::nfc::ndef::MessageBuilder; 00034 using mbed::nfc::ndef::common::URI; 00035 using mbed::nfc::ndef::common::span_from_cstr; 00036 00037 /* URL that will be written into the tag */ 00038 const char url_string[] = "mbed.com"; 00039 00040 class EEPROMExample : mbed::nfc::NFCEEPROM::Delegate 00041 { 00042 public: 00043 EEPROMExample(events::EventQueue& queue, NFCEEPROMDriver& eeprom_driver) : 00044 _ndef_buffer(), 00045 _eeprom(&eeprom_driver, &queue, _ndef_buffer), 00046 _queue(queue) 00047 { } 00048 00049 void run() 00050 { 00051 if (_eeprom.initialize() != NFC_OK) { 00052 printf("failed to initialise\r\n"); 00053 _queue.break_dispatch(); 00054 } 00055 00056 _eeprom.set_delegate(this); 00057 00058 _queue.call(&_eeprom, &NFCEEPROM::write_ndef_message); 00059 } 00060 00061 private: 00062 virtual void on_ndef_message_written(nfc_err_t result) { 00063 if (result == NFC_OK) { 00064 printf("message written successfully\r\n"); 00065 } else { 00066 printf("failed to write (error: %d)\r\n", result); 00067 } 00068 00069 _queue.call(&_eeprom, &NFCEEPROM::read_ndef_message); 00070 } 00071 00072 virtual void on_ndef_message_read(nfc_err_t result) { 00073 if (result == NFC_OK) { 00074 printf("message read successfully\r\n"); 00075 } else { 00076 printf("failed to read (error: %d)\r\n", result); 00077 } 00078 } 00079 00080 virtual void parse_ndef_message(const Span<const uint8_t> &buffer) { 00081 printf("Received an ndef message of size %d\r\n", buffer.size()); 00082 } 00083 00084 virtual size_t build_ndef_message(const Span<uint8_t> &buffer) { 00085 printf("Building an ndef message\r\n"); 00086 00087 /* create a message containing the URL */ 00088 00089 MessageBuilder builder(buffer); 00090 00091 /* URI expected a non-null terminated string so we use a helper function that casts 00092 * the pointer into a Span of size smaller by one */ 00093 URI uri(URI::HTTPS_WWW, span_from_cstr(url_string)); 00094 00095 uri.append_as_record(builder, true); 00096 00097 return builder.get_message().size(); 00098 } 00099 00100 private: 00101 uint8_t _ndef_buffer[1024]; 00102 NFCEEPROM _eeprom; 00103 EventQueue& _queue; 00104 }; 00105 00106 int main() 00107 { 00108 EventQueue queue; 00109 NFCEEPROMDriver& eeprom_driver = get_eeprom_driver(queue); 00110 00111 EEPROMExample example(queue, eeprom_driver); 00112 00113 example.run(); 00114 queue.dispatch_forever(); 00115 00116 return 0; 00117 } 00118
Generated on Thu Jul 14 2022 02:40:13 by
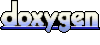