
.
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2019 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #include "mbed.h" 00017 #include <stdio.h> 00018 #include <errno.h> 00019 00020 #include "BlockDevice.h" 00021 00022 // Maximum number of elements in buffer 00023 #define BUFFER_MAX_LEN 10 00024 00025 // This will take the system's default block device 00026 BlockDevice *bd = BlockDevice::get_default_instance(); 00027 00028 // Instead of the default block device, you can define your own block device. 00029 // For example: HeapBlockDevice with size of 2048 bytes, read size 1, write size 1 and erase size 512. 00030 // #include "HeapBlockDevice.h" 00031 // BlockDevice *bd = new HeapBlockDevice(2048, 1, 1, 512); 00032 00033 00034 // This example uses LittleFileSystem as the default file system 00035 #include "LittleFileSystem.h" 00036 LittleFileSystem fs("fs"); 00037 00038 // Uncomment the following two lines and comment the previous two to use FAT file system. 00039 // #include "FATFileSystem.h" 00040 // FATFileSystem fs("fs"); 00041 00042 00043 // Set up the button to trigger an erase 00044 InterruptIn irq(BUTTON1); 00045 void erase() { 00046 printf("Initializing the block device... "); 00047 fflush(stdout); 00048 int err = bd->init(); 00049 printf("%s\n", (err ? "Fail :(" : "OK")); 00050 if (err) { 00051 error("error: %s (%d)\n", strerror(-err), err); 00052 } 00053 00054 printf("Erasing the block device... "); 00055 fflush(stdout); 00056 err = bd->erase(0, bd->size()); 00057 printf("%s\n", (err ? "Fail :(" : "OK")); 00058 if (err) { 00059 error("error: %s (%d)\n", strerror(-err), err); 00060 } 00061 00062 printf("Deinitializing the block device... "); 00063 fflush(stdout); 00064 err = bd->deinit(); 00065 printf("%s\n", (err ? "Fail :(" : "OK")); 00066 if (err) { 00067 error("error: %s (%d)\n", strerror(-err), err); 00068 } 00069 } 00070 00071 00072 // Entry point for the example 00073 int main() { 00074 printf("--- Mbed OS filesystem example ---\n"); 00075 00076 // Setup the erase event on button press, use the event queue 00077 // to avoid running in interrupt context 00078 irq.fall(mbed_event_queue()->event(erase)); 00079 00080 // Try to mount the filesystem 00081 printf("Mounting the filesystem... "); 00082 fflush(stdout); 00083 int err = fs.mount(bd); 00084 printf("%s\n", (err ? "Fail :(" : "OK")); 00085 if (err) { 00086 // Reformat if we can't mount the filesystem 00087 // this should only happen on the first boot 00088 printf("No filesystem found, formatting... "); 00089 fflush(stdout); 00090 err = fs.reformat(bd); 00091 printf("%s\n", (err ? "Fail :(" : "OK")); 00092 if (err) { 00093 error("error: %s (%d)\n", strerror(-err), err); 00094 } 00095 } 00096 00097 // Open the numbers file 00098 printf("Opening \"/fs/numbers.txt\"... "); 00099 fflush(stdout); 00100 FILE *f = fopen("/fs/numbers.txt", "r+"); 00101 printf("%s\n", (!f ? "Fail :(" : "OK")); 00102 if (!f) { 00103 // Create the numbers file if it doesn't exist 00104 printf("No file found, creating a new file... "); 00105 fflush(stdout); 00106 f = fopen("/fs/numbers.txt", "w+"); 00107 printf("%s\n", (!f ? "Fail :(" : "OK")); 00108 if (!f) { 00109 error("error: %s (%d)\n", strerror(errno), -errno); 00110 } 00111 00112 for (int i = 0; i < 10; i++) { 00113 printf("\rWriting numbers (%d/%d)... ", i, 10); 00114 fflush(stdout); 00115 err = fprintf(f, " %d\n", i); 00116 if (err < 0) { 00117 printf("Fail :(\n"); 00118 error("error: %s (%d)\n", strerror(errno), -errno); 00119 } 00120 } 00121 printf("\rWriting numbers (%d/%d)... OK\n", 10, 10); 00122 00123 printf("Seeking file... "); 00124 fflush(stdout); 00125 err = fseek(f, 0, SEEK_SET); 00126 printf("%s\n", (err < 0 ? "Fail :(" : "OK")); 00127 if (err < 0) { 00128 error("error: %s (%d)\n", strerror(errno), -errno); 00129 } 00130 } 00131 00132 // Go through and increment the numbers 00133 for (int i = 0; i < 10; i++) { 00134 printf("\rIncrementing numbers (%d/%d)... ", i, 10); 00135 fflush(stdout); 00136 00137 // Get current stream position 00138 long pos = ftell(f); 00139 00140 // Parse out the number and increment 00141 char buf[BUFFER_MAX_LEN]; 00142 if (!fgets(buf, BUFFER_MAX_LEN, f)) { 00143 error("error: %s (%d)\n", strerror(errno), -errno); 00144 } 00145 char *endptr; 00146 int32_t number = strtol(buf, &endptr, 10); 00147 if ( 00148 (errno == ERANGE) || // The number is too small/large 00149 (endptr == buf) || // No character was read 00150 (*endptr && *endptr != '\n') // The whole input was not converted 00151 ) { 00152 continue; 00153 } 00154 number += 1; 00155 00156 // Seek to beginning of number 00157 fseek(f, pos, SEEK_SET); 00158 00159 // Store number 00160 fprintf(f, " %d\n", number); 00161 00162 // Flush between write and read on same file 00163 fflush(f); 00164 } 00165 printf("\rIncrementing numbers (%d/%d)... OK\n", 10, 10); 00166 00167 // Close the file which also flushes any cached writes 00168 printf("Closing \"/fs/numbers.txt\"... "); 00169 fflush(stdout); 00170 err = fclose(f); 00171 printf("%s\n", (err < 0 ? "Fail :(" : "OK")); 00172 if (err < 0) { 00173 error("error: %s (%d)\n", strerror(errno), -errno); 00174 } 00175 00176 // Display the root directory 00177 printf("Opening the root directory... "); 00178 fflush(stdout); 00179 DIR *d = opendir("/fs/"); 00180 printf("%s\n", (!d ? "Fail :(" : "OK")); 00181 if (!d) { 00182 error("error: %s (%d)\n", strerror(errno), -errno); 00183 } 00184 00185 printf("root directory:\n"); 00186 while (true) { 00187 struct dirent *e = readdir(d); 00188 if (!e) { 00189 break; 00190 } 00191 00192 printf(" %s\n", e->d_name); 00193 } 00194 00195 printf("Closing the root directory... "); 00196 fflush(stdout); 00197 err = closedir(d); 00198 printf("%s\n", (err < 0 ? "Fail :(" : "OK")); 00199 if (err < 0) { 00200 error("error: %s (%d)\n", strerror(errno), -errno); 00201 } 00202 00203 // Display the numbers file 00204 printf("Opening \"/fs/numbers.txt\"... "); 00205 fflush(stdout); 00206 f = fopen("/fs/numbers.txt", "r"); 00207 printf("%s\n", (!f ? "Fail :(" : "OK")); 00208 if (!f) { 00209 error("error: %s (%d)\n", strerror(errno), -errno); 00210 } 00211 00212 printf("numbers:\n"); 00213 while (!feof(f)) { 00214 int c = fgetc(f); 00215 printf("%c", c); 00216 } 00217 00218 printf("\rClosing \"/fs/numbers.txt\"... "); 00219 fflush(stdout); 00220 err = fclose(f); 00221 printf("%s\n", (err < 0 ? "Fail :(" : "OK")); 00222 if (err < 0) { 00223 error("error: %s (%d)\n", strerror(errno), -errno); 00224 } 00225 00226 // Tidy up 00227 printf("Unmounting... "); 00228 fflush(stdout); 00229 err = fs.unmount(); 00230 printf("%s\n", (err < 0 ? "Fail :(" : "OK")); 00231 if (err < 0) { 00232 error("error: %s (%d)\n", strerror(-err), err); 00233 } 00234 00235 printf("Mbed OS filesystem example done!\n"); 00236 } 00237
Generated on Thu Jul 14 2022 23:05:20 by
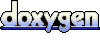