CMSIS DSP library
Dependents: KL25Z_FFT_Demo Hat_Board_v5_1 KL25Z_FFT_Demo_tony KL25Z_FFT_Demo_tony ... more
Fork of mbed-dsp by
arm_rfft_q31.c
00001 /* ---------------------------------------------------------------------- 00002 * Copyright (C) 2010-2013 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 17. January 2013 00005 * $Revision: V1.4.1 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_rfft_q31.c 00009 * 00010 * Description: RFFT & RIFFT Q31 process function 00011 * 00012 * 00013 * Target Processor: Cortex-M4/Cortex-M3/Cortex-M0 00014 * 00015 * Redistribution and use in source and binary forms, with or without 00016 * modification, are permitted provided that the following conditions 00017 * are met: 00018 * - Redistributions of source code must retain the above copyright 00019 * notice, this list of conditions and the following disclaimer. 00020 * - Redistributions in binary form must reproduce the above copyright 00021 * notice, this list of conditions and the following disclaimer in 00022 * the documentation and/or other materials provided with the 00023 * distribution. 00024 * - Neither the name of ARM LIMITED nor the names of its contributors 00025 * may be used to endorse or promote products derived from this 00026 * software without specific prior written permission. 00027 * 00028 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00029 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00030 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS 00031 * FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE 00032 * COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, 00033 * INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, 00034 * BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00035 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00036 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT 00037 * LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN 00038 * ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 00039 * POSSIBILITY OF SUCH DAMAGE. 00040 * -------------------------------------------------------------------- */ 00041 00042 #include "arm_math.h" 00043 00044 void arm_radix4_butterfly_inverse_q31( 00045 q31_t * pSrc, 00046 uint32_t fftLen, 00047 q31_t * pCoef, 00048 uint32_t twidCoefModifier); 00049 00050 void arm_radix4_butterfly_q31( 00051 q31_t * pSrc, 00052 uint32_t fftLen, 00053 q31_t * pCoef, 00054 uint32_t twidCoefModifier); 00055 00056 void arm_bitreversal_q31( 00057 q31_t * pSrc, 00058 uint32_t fftLen, 00059 uint16_t bitRevFactor, 00060 uint16_t * pBitRevTab); 00061 00062 /*-------------------------------------------------------------------- 00063 * Internal functions prototypes 00064 --------------------------------------------------------------------*/ 00065 00066 void arm_split_rfft_q31( 00067 q31_t * pSrc, 00068 uint32_t fftLen, 00069 q31_t * pATable, 00070 q31_t * pBTable, 00071 q31_t * pDst, 00072 uint32_t modifier); 00073 00074 void arm_split_rifft_q31( 00075 q31_t * pSrc, 00076 uint32_t fftLen, 00077 q31_t * pATable, 00078 q31_t * pBTable, 00079 q31_t * pDst, 00080 uint32_t modifier); 00081 00082 /** 00083 * @addtogroup RealFFT 00084 * @{ 00085 */ 00086 00087 /** 00088 * @brief Processing function for the Q31 RFFT/RIFFT. 00089 * @param[in] *S points to an instance of the Q31 RFFT/RIFFT structure. 00090 * @param[in] *pSrc points to the input buffer. 00091 * @param[out] *pDst points to the output buffer. 00092 * @return none. 00093 * 00094 * \par Input an output formats: 00095 * \par 00096 * Internally input is downscaled by 2 for every stage to avoid saturations inside CFFT/CIFFT process. 00097 * Hence the output format is different for different RFFT sizes. 00098 * The input and output formats for different RFFT sizes and number of bits to upscale are mentioned in the tables below for RFFT and RIFFT: 00099 * \par 00100 * \image html RFFTQ31.gif "Input and Output Formats for Q31 RFFT" 00101 * 00102 * \par 00103 * \image html RIFFTQ31.gif "Input and Output Formats for Q31 RIFFT" 00104 */ 00105 00106 void arm_rfft_q31( 00107 const arm_rfft_instance_q31 * S, 00108 q31_t * pSrc, 00109 q31_t * pDst) 00110 { 00111 const arm_cfft_radix4_instance_q31 *S_CFFT = S->pCfft; 00112 00113 /* Calculation of RIFFT of input */ 00114 if(S->ifftFlagR == 1u) 00115 { 00116 /* Real IFFT core process */ 00117 arm_split_rifft_q31(pSrc, S->fftLenBy2, S->pTwiddleAReal, 00118 S->pTwiddleBReal, pDst, S->twidCoefRModifier); 00119 00120 /* Complex readix-4 IFFT process */ 00121 arm_radix4_butterfly_inverse_q31(pDst, S_CFFT->fftLen, 00122 S_CFFT->pTwiddle, 00123 S_CFFT->twidCoefModifier); 00124 /* Bit reversal process */ 00125 if(S->bitReverseFlagR == 1u) 00126 { 00127 arm_bitreversal_q31(pDst, S_CFFT->fftLen, 00128 S_CFFT->bitRevFactor, S_CFFT->pBitRevTable); 00129 } 00130 } 00131 else 00132 { 00133 /* Calculation of RFFT of input */ 00134 00135 /* Complex readix-4 FFT process */ 00136 arm_radix4_butterfly_q31(pSrc, S_CFFT->fftLen, 00137 S_CFFT->pTwiddle, S_CFFT->twidCoefModifier); 00138 00139 /* Bit reversal process */ 00140 if(S->bitReverseFlagR == 1u) 00141 { 00142 arm_bitreversal_q31(pSrc, S_CFFT->fftLen, 00143 S_CFFT->bitRevFactor, S_CFFT->pBitRevTable); 00144 } 00145 00146 /* Real FFT core process */ 00147 arm_split_rfft_q31(pSrc, S->fftLenBy2, S->pTwiddleAReal, 00148 S->pTwiddleBReal, pDst, S->twidCoefRModifier); 00149 } 00150 00151 } 00152 00153 00154 /** 00155 * @} end of RealFFT group 00156 */ 00157 00158 /** 00159 * @brief Core Real FFT process 00160 * @param[in] *pSrc points to the input buffer. 00161 * @param[in] fftLen length of FFT. 00162 * @param[in] *pATable points to the twiddle Coef A buffer. 00163 * @param[in] *pBTable points to the twiddle Coef B buffer. 00164 * @param[out] *pDst points to the output buffer. 00165 * @param[in] modifier twiddle coefficient modifier that supports different size FFTs with the same twiddle factor table. 00166 * @return none. 00167 */ 00168 00169 void arm_split_rfft_q31( 00170 q31_t * pSrc, 00171 uint32_t fftLen, 00172 q31_t * pATable, 00173 q31_t * pBTable, 00174 q31_t * pDst, 00175 uint32_t modifier) 00176 { 00177 uint32_t i; /* Loop Counter */ 00178 q31_t outR, outI; /* Temporary variables for output */ 00179 q31_t *pCoefA, *pCoefB; /* Temporary pointers for twiddle factors */ 00180 q31_t CoefA1, CoefA2, CoefB1; /* Temporary variables for twiddle coefficients */ 00181 q31_t *pOut1 = &pDst[2], *pOut2 = &pDst[(4u * fftLen) - 1u]; 00182 q31_t *pIn1 = &pSrc[2], *pIn2 = &pSrc[(2u * fftLen) - 1u]; 00183 00184 /* Init coefficient pointers */ 00185 pCoefA = &pATable[modifier * 2u]; 00186 pCoefB = &pBTable[modifier * 2u]; 00187 00188 i = fftLen - 1u; 00189 00190 while(i > 0u) 00191 { 00192 /* 00193 outR = (pSrc[2 * i] * pATable[2 * i] - pSrc[2 * i + 1] * pATable[2 * i + 1] 00194 + pSrc[2 * n - 2 * i] * pBTable[2 * i] + 00195 pSrc[2 * n - 2 * i + 1] * pBTable[2 * i + 1]); 00196 */ 00197 00198 /* outI = (pIn[2 * i + 1] * pATable[2 * i] + pIn[2 * i] * pATable[2 * i + 1] + 00199 pIn[2 * n - 2 * i] * pBTable[2 * i + 1] - 00200 pIn[2 * n - 2 * i + 1] * pBTable[2 * i]); */ 00201 00202 CoefA1 = *pCoefA++; 00203 CoefA2 = *pCoefA; 00204 00205 /* outR = (pSrc[2 * i] * pATable[2 * i] */ 00206 outR = ((int32_t) (((q63_t) * pIn1 * CoefA1) >> 32)); 00207 00208 /* outI = pIn[2 * i] * pATable[2 * i + 1] */ 00209 outI = ((int32_t) (((q63_t) * pIn1++ * CoefA2) >> 32)); 00210 00211 /* - pSrc[2 * i + 1] * pATable[2 * i + 1] */ 00212 outR = 00213 (q31_t) ((((q63_t) outR << 32) + ((q63_t) * pIn1 * (-CoefA2))) >> 32); 00214 00215 /* (pIn[2 * i + 1] * pATable[2 * i] */ 00216 outI = 00217 (q31_t) ((((q63_t) outI << 32) + ((q63_t) * pIn1++ * (CoefA1))) >> 32); 00218 00219 /* pSrc[2 * n - 2 * i] * pBTable[2 * i] */ 00220 outR = 00221 (q31_t) ((((q63_t) outR << 32) + ((q63_t) * pIn2 * (-CoefA2))) >> 32); 00222 CoefB1 = *pCoefB; 00223 00224 /* pIn[2 * n - 2 * i] * pBTable[2 * i + 1] */ 00225 outI = 00226 (q31_t) ((((q63_t) outI << 32) + ((q63_t) * pIn2-- * (-CoefB1))) >> 32); 00227 00228 /* pSrc[2 * n - 2 * i + 1] * pBTable[2 * i + 1] */ 00229 outR = 00230 (q31_t) ((((q63_t) outR << 32) + ((q63_t) * pIn2 * (CoefB1))) >> 32); 00231 00232 /* pIn[2 * n - 2 * i + 1] * pBTable[2 * i] */ 00233 outI = 00234 (q31_t) ((((q63_t) outI << 32) + ((q63_t) * pIn2-- * (-CoefA2))) >> 32); 00235 00236 /* write output */ 00237 *pOut1++ = (outR << 1u); 00238 *pOut1++ = (outI << 1u); 00239 00240 /* write complex conjugate output */ 00241 *pOut2-- = -(outI << 1u); 00242 *pOut2-- = (outR << 1u); 00243 00244 /* update coefficient pointer */ 00245 pCoefB = pCoefB + (modifier * 2u); 00246 pCoefA = pCoefA + ((modifier * 2u) - 1u); 00247 00248 i--; 00249 00250 } 00251 00252 pDst[2u * fftLen] = pSrc[0] - pSrc[1]; 00253 pDst[(2u * fftLen) + 1u] = 0; 00254 00255 pDst[0] = pSrc[0] + pSrc[1]; 00256 pDst[1] = 0; 00257 00258 } 00259 00260 00261 /** 00262 * @brief Core Real IFFT process 00263 * @param[in] *pSrc points to the input buffer. 00264 * @param[in] fftLen length of FFT. 00265 * @param[in] *pATable points to the twiddle Coef A buffer. 00266 * @param[in] *pBTable points to the twiddle Coef B buffer. 00267 * @param[out] *pDst points to the output buffer. 00268 * @param[in] modifier twiddle coefficient modifier that supports different size FFTs with the same twiddle factor table. 00269 * @return none. 00270 */ 00271 00272 void arm_split_rifft_q31( 00273 q31_t * pSrc, 00274 uint32_t fftLen, 00275 q31_t * pATable, 00276 q31_t * pBTable, 00277 q31_t * pDst, 00278 uint32_t modifier) 00279 { 00280 q31_t outR, outI; /* Temporary variables for output */ 00281 q31_t *pCoefA, *pCoefB; /* Temporary pointers for twiddle factors */ 00282 q31_t CoefA1, CoefA2, CoefB1; /* Temporary variables for twiddle coefficients */ 00283 q31_t *pIn1 = &pSrc[0], *pIn2 = &pSrc[(2u * fftLen) + 1u]; 00284 00285 pCoefA = &pATable[0]; 00286 pCoefB = &pBTable[0]; 00287 00288 while(fftLen > 0u) 00289 { 00290 /* 00291 outR = (pIn[2 * i] * pATable[2 * i] + pIn[2 * i + 1] * pATable[2 * i + 1] + 00292 pIn[2 * n - 2 * i] * pBTable[2 * i] - 00293 pIn[2 * n - 2 * i + 1] * pBTable[2 * i + 1]); 00294 00295 outI = (pIn[2 * i + 1] * pATable[2 * i] - pIn[2 * i] * pATable[2 * i + 1] - 00296 pIn[2 * n - 2 * i] * pBTable[2 * i + 1] - 00297 pIn[2 * n - 2 * i + 1] * pBTable[2 * i]); 00298 00299 */ 00300 CoefA1 = *pCoefA++; 00301 CoefA2 = *pCoefA; 00302 00303 /* outR = (pIn[2 * i] * pATable[2 * i] */ 00304 outR = ((int32_t) (((q63_t) * pIn1 * CoefA1) >> 32)); 00305 00306 /* - pIn[2 * i] * pATable[2 * i + 1] */ 00307 outI = -((int32_t) (((q63_t) * pIn1++ * CoefA2) >> 32)); 00308 00309 /* pIn[2 * i + 1] * pATable[2 * i + 1] */ 00310 outR = 00311 (q31_t) ((((q63_t) outR << 32) + ((q63_t) * pIn1 * (CoefA2))) >> 32); 00312 00313 /* pIn[2 * i + 1] * pATable[2 * i] */ 00314 outI = 00315 (q31_t) ((((q63_t) outI << 32) + ((q63_t) * pIn1++ * (CoefA1))) >> 32); 00316 00317 /* pIn[2 * n - 2 * i] * pBTable[2 * i] */ 00318 outR = 00319 (q31_t) ((((q63_t) outR << 32) + ((q63_t) * pIn2 * (CoefA2))) >> 32); 00320 00321 CoefB1 = *pCoefB; 00322 00323 /* pIn[2 * n - 2 * i] * pBTable[2 * i + 1] */ 00324 outI = 00325 (q31_t) ((((q63_t) outI << 32) - ((q63_t) * pIn2-- * (CoefB1))) >> 32); 00326 00327 /* pIn[2 * n - 2 * i + 1] * pBTable[2 * i + 1] */ 00328 outR = 00329 (q31_t) ((((q63_t) outR << 32) + ((q63_t) * pIn2 * (CoefB1))) >> 32); 00330 00331 /* pIn[2 * n - 2 * i + 1] * pBTable[2 * i] */ 00332 outI = 00333 (q31_t) ((((q63_t) outI << 32) + ((q63_t) * pIn2-- * (CoefA2))) >> 32); 00334 00335 /* write output */ 00336 *pDst++ = (outR << 1u); 00337 *pDst++ = (outI << 1u); 00338 00339 /* update coefficient pointer */ 00340 pCoefB = pCoefB + (modifier * 2u); 00341 pCoefA = pCoefA + ((modifier * 2u) - 1u); 00342 00343 /* Decrement loop count */ 00344 fftLen--; 00345 00346 } 00347 00348 00349 }
Generated on Tue Jul 12 2022 12:36:57 by
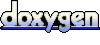