CMSIS DSP library
Dependents: KL25Z_FFT_Demo Hat_Board_v5_1 KL25Z_FFT_Demo_tony KL25Z_FFT_Demo_tony ... more
Fork of mbed-dsp by
arm_mat_trans_q15.c
00001 /* ---------------------------------------------------------------------- 00002 * Copyright (C) 2010-2013 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 17. January 2013 00005 * $Revision: V1.4.1 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_mat_trans_q15.c 00009 * 00010 * Description: Q15 matrix transpose. 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3/Cortex-M0 00013 * 00014 * Redistribution and use in source and binary forms, with or without 00015 * modification, are permitted provided that the following conditions 00016 * are met: 00017 * - Redistributions of source code must retain the above copyright 00018 * notice, this list of conditions and the following disclaimer. 00019 * - Redistributions in binary form must reproduce the above copyright 00020 * notice, this list of conditions and the following disclaimer in 00021 * the documentation and/or other materials provided with the 00022 * distribution. 00023 * - Neither the name of ARM LIMITED nor the names of its contributors 00024 * may be used to endorse or promote products derived from this 00025 * software without specific prior written permission. 00026 * 00027 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00028 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00029 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS 00030 * FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE 00031 * COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, 00032 * INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, 00033 * BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00034 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00035 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT 00036 * LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN 00037 * ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 00038 * POSSIBILITY OF SUCH DAMAGE. 00039 * -------------------------------------------------------------------- */ 00040 00041 #include "arm_math.h" 00042 00043 /** 00044 * @ingroup groupMatrix 00045 */ 00046 00047 /** 00048 * @addtogroup MatrixTrans 00049 * @{ 00050 */ 00051 00052 /* 00053 * @brief Q15 matrix transpose. 00054 * @param[in] *pSrc points to the input matrix 00055 * @param[out] *pDst points to the output matrix 00056 * @return The function returns either <code>ARM_MATH_SIZE_MISMATCH</code> 00057 * or <code>ARM_MATH_SUCCESS</code> based on the outcome of size checking. 00058 */ 00059 00060 arm_status arm_mat_trans_q15( 00061 const arm_matrix_instance_q15 * pSrc, 00062 arm_matrix_instance_q15 * pDst) 00063 { 00064 q15_t *pSrcA = pSrc->pData; /* input data matrix pointer */ 00065 q15_t *pOut = pDst->pData; /* output data matrix pointer */ 00066 uint16_t nRows = pSrc->numRows; /* number of nRows */ 00067 uint16_t nColumns = pSrc->numCols; /* number of nColumns */ 00068 uint16_t col, row = nRows, i = 0u; /* row and column loop counters */ 00069 arm_status status; /* status of matrix transpose */ 00070 00071 #ifndef ARM_MATH_CM0_FAMILY 00072 00073 /* Run the below code for Cortex-M4 and Cortex-M3 */ 00074 #ifndef UNALIGNED_SUPPORT_DISABLE 00075 00076 q31_t in; /* variable to hold temporary output */ 00077 00078 #else 00079 00080 q15_t in; 00081 00082 #endif /* #ifndef UNALIGNED_SUPPORT_DISABLE */ 00083 00084 #ifdef ARM_MATH_MATRIX_CHECK 00085 00086 00087 /* Check for matrix mismatch condition */ 00088 if((pSrc->numRows != pDst->numCols) || (pSrc->numCols != pDst->numRows)) 00089 { 00090 /* Set status as ARM_MATH_SIZE_MISMATCH */ 00091 status = ARM_MATH_SIZE_MISMATCH; 00092 } 00093 else 00094 #endif /* #ifdef ARM_MATH_MATRIX_CHECK */ 00095 00096 { 00097 /* Matrix transpose by exchanging the rows with columns */ 00098 /* row loop */ 00099 do 00100 { 00101 00102 /* Apply loop unrolling and exchange the columns with row elements */ 00103 col = nColumns >> 2u; 00104 00105 /* The pointer pOut is set to starting address of the column being processed */ 00106 pOut = pDst->pData + i; 00107 00108 /* First part of the processing with loop unrolling. Compute 4 outputs at a time. 00109 ** a second loop below computes the remaining 1 to 3 samples. */ 00110 while(col > 0u) 00111 { 00112 #ifndef UNALIGNED_SUPPORT_DISABLE 00113 00114 /* Read two elements from the row */ 00115 in = *__SIMD32(pSrcA)++; 00116 00117 /* Unpack and store one element in the destination */ 00118 #ifndef ARM_MATH_BIG_ENDIAN 00119 00120 *pOut = (q15_t) in; 00121 00122 #else 00123 00124 *pOut = (q15_t) ((in & (q31_t) 0xffff0000) >> 16); 00125 00126 #endif /* #ifndef ARM_MATH_BIG_ENDIAN */ 00127 00128 /* Update the pointer pOut to point to the next row of the transposed matrix */ 00129 pOut += nRows; 00130 00131 /* Unpack and store the second element in the destination */ 00132 00133 #ifndef ARM_MATH_BIG_ENDIAN 00134 00135 *pOut = (q15_t) ((in & (q31_t) 0xffff0000) >> 16); 00136 00137 #else 00138 00139 *pOut = (q15_t) in; 00140 00141 #endif /* #ifndef ARM_MATH_BIG_ENDIAN */ 00142 00143 /* Update the pointer pOut to point to the next row of the transposed matrix */ 00144 pOut += nRows; 00145 00146 /* Read two elements from the row */ 00147 #ifndef ARM_MATH_BIG_ENDIAN 00148 00149 in = *__SIMD32(pSrcA)++; 00150 00151 #else 00152 00153 in = *__SIMD32(pSrcA)++; 00154 00155 #endif /* #ifndef ARM_MATH_BIG_ENDIAN */ 00156 00157 /* Unpack and store one element in the destination */ 00158 #ifndef ARM_MATH_BIG_ENDIAN 00159 00160 *pOut = (q15_t) in; 00161 00162 #else 00163 00164 *pOut = (q15_t) ((in & (q31_t) 0xffff0000) >> 16); 00165 00166 #endif /* #ifndef ARM_MATH_BIG_ENDIAN */ 00167 00168 /* Update the pointer pOut to point to the next row of the transposed matrix */ 00169 pOut += nRows; 00170 00171 /* Unpack and store the second element in the destination */ 00172 #ifndef ARM_MATH_BIG_ENDIAN 00173 00174 *pOut = (q15_t) ((in & (q31_t) 0xffff0000) >> 16); 00175 00176 #else 00177 00178 *pOut = (q15_t) in; 00179 00180 #endif /* #ifndef ARM_MATH_BIG_ENDIAN */ 00181 00182 #else 00183 /* Read one element from the row */ 00184 in = *pSrcA++; 00185 00186 /* Store one element in the destination */ 00187 *pOut = in; 00188 00189 /* Update the pointer px to point to the next row of the transposed matrix */ 00190 pOut += nRows; 00191 00192 /* Read one element from the row */ 00193 in = *pSrcA++; 00194 00195 /* Store one element in the destination */ 00196 *pOut = in; 00197 00198 /* Update the pointer px to point to the next row of the transposed matrix */ 00199 pOut += nRows; 00200 00201 /* Read one element from the row */ 00202 in = *pSrcA++; 00203 00204 /* Store one element in the destination */ 00205 *pOut = in; 00206 00207 /* Update the pointer px to point to the next row of the transposed matrix */ 00208 pOut += nRows; 00209 00210 /* Read one element from the row */ 00211 in = *pSrcA++; 00212 00213 /* Store one element in the destination */ 00214 *pOut = in; 00215 00216 #endif /* #ifndef UNALIGNED_SUPPORT_DISABLE */ 00217 00218 /* Update the pointer pOut to point to the next row of the transposed matrix */ 00219 pOut += nRows; 00220 00221 /* Decrement the column loop counter */ 00222 col--; 00223 } 00224 00225 /* Perform matrix transpose for last 3 samples here. */ 00226 col = nColumns % 0x4u; 00227 00228 #else 00229 00230 /* Run the below code for Cortex-M0 */ 00231 00232 #ifdef ARM_MATH_MATRIX_CHECK 00233 00234 /* Check for matrix mismatch condition */ 00235 if((pSrc->numRows != pDst->numCols) || (pSrc->numCols != pDst->numRows)) 00236 { 00237 /* Set status as ARM_MATH_SIZE_MISMATCH */ 00238 status = ARM_MATH_SIZE_MISMATCH; 00239 } 00240 else 00241 #endif /* #ifdef ARM_MATH_MATRIX_CHECK */ 00242 00243 { 00244 /* Matrix transpose by exchanging the rows with columns */ 00245 /* row loop */ 00246 do 00247 { 00248 /* The pointer pOut is set to starting address of the column being processed */ 00249 pOut = pDst->pData + i; 00250 00251 /* Initialize column loop counter */ 00252 col = nColumns; 00253 00254 #endif /* #ifndef ARM_MATH_CM0_FAMILY */ 00255 00256 while(col > 0u) 00257 { 00258 /* Read and store the input element in the destination */ 00259 *pOut = *pSrcA++; 00260 00261 /* Update the pointer pOut to point to the next row of the transposed matrix */ 00262 pOut += nRows; 00263 00264 /* Decrement the column loop counter */ 00265 col--; 00266 } 00267 00268 i++; 00269 00270 /* Decrement the row loop counter */ 00271 row--; 00272 00273 } while(row > 0u); 00274 00275 /* set status as ARM_MATH_SUCCESS */ 00276 status = ARM_MATH_SUCCESS; 00277 } 00278 /* Return to application */ 00279 return (status); 00280 } 00281 00282 /** 00283 * @} end of MatrixTrans group 00284 */
Generated on Tue Jul 12 2022 12:36:56 by
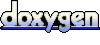