
pasword
Dependencies: Keypadlatest TextLCD
main.cpp
00001 00002 #include "mbed.h" 00003 #include "Keypad.h" 00004 #include "TextLCD.h" 00005 #include "string.h" 00006 TextLCD display(D8, D9, D4, D5, D6, D7); // rs, e, d4-d7 00007 00008 char kpdLayout[4][4] = {{'1' ,'2' ,'3' ,'A'}, //row0 00009 {'4' ,'5' ,'6' ,'B'}, //row1 00010 {'7' ,'8' ,'9' ,'C'}, //row2 00011 {'*' ,'0' ,'#' ,'D'}}; //row3 00012 00013 Keypad kpad(PB_14, PB_15, PB_1, PB_2, PB_11, PB_12, PA_11, PA_12); 00014 char password[20]; 00015 const char code1[]="12345678"; 00016 int main() { 00017 char key; 00018 int released = 1,count=0; 00019 display.locate(0,0); 00020 display.printf("insert password"); 00021 while(1){ 00022 key = kpad.ReadKey(); //read the current key pressed 00023 if(key == '\0') 00024 released = 1; //set the flag when all keys are released 00025 if((key != '\0') && (released == 1)) 00026 { //if a key is pressed AND previous key was released 00027 if(key!='#') 00028 { password[count]=key; // add pasword 00029 password[count+1]='\0'; // put end of password 00030 count++; //counter 00031 if(count>8) //max count 00032 count=0; 00033 display.cls(); 00034 display.locate(0,0); 00035 display.printf("insert password"); 00036 display.locate(2,1); 00037 display.printf("pass:%s",password); 00038 } 00039 else if(key=='#') 00040 { bool accept=1; 00041 for(int i=0;password[i]!='\0';i++) 00042 { if(password[i]!=code1[i]) 00043 { accept=0; 00044 break; 00045 } 00046 } 00047 display.cls(); 00048 if(accept==0) 00049 { display.locate(0,0); 00050 display.printf("wrong password "); 00051 } 00052 else 00053 { display.locate(0,0); 00054 display.printf("accept password"); 00055 } 00056 count=0; // reset password counter 00057 } 00058 00059 00060 00061 released = 0; //clear the flag to indicate that key is still pressed 00062 } 00063 } 00064 }
Generated on Tue Jul 12 2022 20:10:38 by
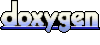