
add iphone Support.
Fork of Led_demo by
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "NeoStrip.h" 00003 #include "text.h" 00004 #define N 128 00005 #define PATTERNS 4 00006 #include <string> 00007 #include "helper.h" 00008 //callback function for incoming serial communication 00009 void callback(); 00010 //necessary functions for distanceDemo and bikeDemo 00011 void blinkOn(); 00012 void blinkOff(); 00013 void patternLeft(); 00014 void patternRight(); 00015 void patternStop(); 00016 void patternNone(); 00017 void distanceDemo(); 00018 00019 //necessary functions for Animation Demo 00020 int hueToRGB(float h); 00021 void rainBow(); 00022 void fillBlue(); 00023 void progressBar(bool); 00024 void putChar(int row, int col, char ch, int color); 00025 void putString(std::string); 00026 void loopAscii(int count); 00027 void MarioBox(); 00028 void drawBox(int row);void emptyBox(int row); 00029 void putCharV(int row, int col, char ch, int color); 00030 00031 00032 00033 00034 //hardware initilization 00035 NeoStrip strip(p18, N); 00036 Serial device(p13,p14); 00037 AnalogIn ir(p20); 00038 00039 00040 //variable initilization 00041 Timer t; 00042 int chars[128]; 00043 char ch = 'A'; 00044 std::string emoji[9] = {"*_*", "^_^", "$_$", ">_<", "=_=", "+_=", ":)", ":(", ":3"}; 00045 void (*patterns[])(void) = {&patternLeft, &patternRight, &patternStop, &patternNone}; 00046 int bikeDir; 00047 00048 int main() 00049 { 00050 float bright = 0.2; // 20% is plenty for indoor use 00051 strip.setBrightness(bright); // set default brightness 00052 //setup serial device 00053 device.baud(57600); 00054 device.attach(&callback); 00055 00056 //wait until phone is connected 00057 progressBar(false); 00058 while(1){ 00059 if(device.readable()) break; 00060 } 00061 //progress Bar light up on T-shirt to demonstrate connection is established. 00062 00063 progressBar(true); 00064 //ready for commands, clear serael buffer 00065 while(device.readable()) device.getc(); 00066 00067 //phone is disconnected, flash red light warning 00068 while(1){ 00069 if(t.read_ms()>3000){ 00070 blinkOn(); 00071 wait_ms(200); 00072 blinkOff(); 00073 wait_ms(200); 00074 } 00075 00076 } 00077 00078 00079 00080 } 00081 00082 00083 void callback(){ 00084 t.reset(); 00085 t.stop(); 00086 switch(device.getc()){ 00087 00088 case PhoneDemo: 00089 //discard second byte 00090 device.getc(); 00091 //reply ack 00092 device.putc('P'); 00093 t.start(); 00094 break; 00095 00096 case MusicDemo: 00097 device.getc(); 00098 rainBow(); 00099 break; 00100 00101 case DistanceDemo: 00102 device.getc(); 00103 device.putc('D'); 00104 break; 00105 00106 case AnimationOneDemo: 00107 device.getc(); 00108 MarioBox(); 00109 break; 00110 00111 case AnimationTwoDemo: 00112 device.getc(); 00113 for(int i=0;i<8;i++){ 00114 putString(emoji[i]); 00115 } 00116 00117 00118 break; 00119 00120 case AnimationThreeDemo: 00121 device.getc(); 00122 for(int i=0;i<126;i++) loopAscii(i); 00123 break; 00124 00125 case BikeDemo: 00126 bikeDir=device.getc(); 00127 if (bikeDir==LEFT) patternLeft(); 00128 else if(bikeDir==RIGHT) patternRight(); 00129 else if(bikeDir==STOP)patternStop(); 00130 else patternNone(); 00131 break; 00132 00133 default: 00134 break; 00135 } 00136 00137 00138 } 00139 00140 00141 // display a shifting rainbow, all colors have maximum 00142 // saturation and value, with evenly spaced hue 00143 void rainBow() 00144 { 00145 static float dh = 360.0 / N; 00146 static float x = 0; 00147 00148 //rainbow three times 00149 for(int j=0;j<3;j++){ 00150 00151 for (int i = 0; i < N; i++) 00152 strip.setPixel(i, hueToRGB((dh * i) - x)); 00153 00154 x += 1; 00155 if (x > 360) 00156 x = 0; 00157 00158 } 00159 00160 00161 } 00162 00163 00164 00165 // Converts HSV to RGB with the given hue, assuming 00166 // maximum saturation and value 00167 int hueToRGB(float h) 00168 { 00169 // lots of floating point magic from the internet and scratching my head 00170 float r, g, b; 00171 if (h > 360) 00172 h -= 360; 00173 if (h < 0) 00174 h += 360; 00175 int i = (int)(h / 60.0); 00176 float f = (h / 60.0) - i; 00177 float q = 1 - f; 00178 00179 switch (i % 6) 00180 { 00181 case 0: r = 1; g = f; b = 0; break; 00182 case 1: r = q; g = 1; b = 0; break; 00183 case 2: r = 0; g = 1; b = f; break; 00184 case 3: r = 0; g = q; b = 1; break; 00185 case 4: r = f; g = 0; b = 1; break; 00186 case 5: r = 1; g = 0; b = q; break; 00187 default: r = 0; g = 0; b = 0; break; 00188 } 00189 00190 // scale to integers and return the packed value 00191 uint8_t R = (uint8_t)(r * 255); 00192 uint8_t G = (uint8_t)(g * 255); 00193 uint8_t B = (uint8_t)(b * 255); 00194 00195 return (R << 16) | (G << 8) | B; 00196 } 00197 00198 void fillBlue(){ 00199 for(int i = 0; i < N; i++){ 00200 chars[i] = 0x122446; 00201 } 00202 } 00203 00204 00205 00206 00207 void putChar(int row, int col, char ch, int color){ 00208 for(int r = 0; r < 8; r++){ 00209 for(int c = 0; c < 6; c++){ 00210 if(fontdata_6x8[ch * 48 + r * 6 +c]){ 00211 int idx = getIndex(row+r, col+c); 00212 if(idx != -1) 00213 chars[idx] = color; 00214 } 00215 } 00216 } 00217 } 00218 void putCharV(int row, int col, char ch, int color){ 00219 for(int r = 0; r < 8; r++){ 00220 for(int c = 0; c < 6; c++){ 00221 if(fontdata_6x8[ch * 48 + r * 6 +c]){ 00222 int idx = (row + r) *8 +( col +c); 00223 if(idx != -1) 00224 chars[idx] = color; 00225 } 00226 } 00227 } 00228 } 00229 00230 void MarioBox(){ 00231 int color = 0xffff00; 00232 // int red = 0xff0000; 00233 00234 drawBox(8); 00235 strip.setPixels(0, N, chars); 00236 strip.write(); 00237 wait(5); 00238 00239 for(int i = 1; i < 3; i++){ 00240 fillBlue(); 00241 drawBox(8-i); 00242 strip.setPixels(0, N, chars); 00243 strip.write(); 00244 wait(0.3); 00245 } 00246 for(int i = 0; i < 3; i++){ 00247 fillBlue(); 00248 drawBox(6 + i); 00249 strip.setPixels(0, N, chars); 00250 strip.write(); 00251 wait(0.3); 00252 } 00253 fillBlue(); 00254 emptyBox(8); 00255 putChar(0,0,'0',color); 00256 strip.setPixels(0, N, chars); 00257 strip.write(); 00258 wait(30); 00259 } 00260 void putString(std::string str){ 00261 int color = 0xffff00; 00262 for(int i = 0; i < str.length(); ++i){ 00263 putChar(0,5*i,str[i], color); 00264 } 00265 strip.setPixels(0, N, chars); 00266 strip.write(); 00267 00268 } 00269 00270 void loopAscii(int count){ 00271 int color = 0xffff00; 00272 putChar(0,0,ch+count,color); 00273 putChar(0,6,ch+count+1,color); 00274 strip.setPixels(0, N, chars); 00275 strip.write(); 00276 } 00277 00278 void drawBox(int row){ 00279 for(int i = 0; i < 64; ++i) 00280 chars[row*8 + i] = 0x000000; 00281 putCharV(row,0,'?',0xffff00); 00282 } 00283 void emptyBox(int row){ 00284 for(int i = 0; i < 64; ++i) 00285 chars[row*8 + i] = 0x000000; 00286 } 00287 void progressBar(bool up){ 00288 int index; 00289 int init; 00290 int increment; 00291 int color; 00292 if(up == true){ 00293 init = 0; 00294 increment = 1; 00295 color = 0x122446; 00296 }else { 00297 init = 15; 00298 increment = -1; 00299 fillBlue(); 00300 color = 0x000000; 00301 } 00302 00303 for(int x = 0; x < 16 ; x++){ 00304 00305 for (int j = 0; j < 8; j++){ 00306 index = getIndex(j,init + increment * x); 00307 chars[index] = color; 00308 } 00309 strip.setPixels(0, N, chars); 00310 strip.write(); 00311 wait(1); 00312 } 00313 } 00314 00315 00316 00317 void patternLeft() 00318 { 00319 for (int i = 0; i < 59; i++) 00320 { 00321 if (maskLeft[i] == 1) 00322 strip.setPixel(i, 0, 0xff, 0); 00323 else 00324 strip.setPixel(i, 0); 00325 } 00326 } 00327 00328 void patternRight() 00329 { 00330 for (int i = 0; i < 59; i++) 00331 { 00332 if (maskRight[i] == 1) 00333 strip.setPixel(i, 0, 0xff, 0); 00334 else 00335 strip.setPixel(i, 0); 00336 } 00337 } 00338 00339 void patternStop() 00340 { 00341 for (int i = 0; i < 59; i++) 00342 { 00343 if (maskStop[i] == 1) 00344 strip.setPixel(i, 0xff, 0, 0); 00345 else 00346 strip.setPixel(i, 0); 00347 } 00348 } 00349 00350 void patternNone() 00351 { 00352 for (int i = 0; i < 59; i++) 00353 { 00354 strip.setPixel(i, 0); 00355 } 00356 } 00357 00358 00359 00360 void blinkOn() 00361 { 00362 for (int i = 0; i < N; i++) 00363 { 00364 if (i % 2) 00365 strip.setPixel(i, 0xff, 0, 0); 00366 else 00367 strip.setPixel(i, 0, 0, 0); 00368 } 00369 wait_ms(50); 00370 strip.write(); 00371 } 00372 00373 void blinkOff() 00374 { 00375 for (int i = 0; i < N; i++) 00376 { 00377 strip.setPixel(i, 0, 0, 0); 00378 } 00379 wait_ms(60); 00380 strip.write(); 00381 } 00382 00383 00384 00385 void distanceDemo(){ 00386 float temp[10]; 00387 for (int i = 0; i < 10; i++) 00388 { 00389 float val; 00390 val = 21/ir; 00391 temp[i] = val; 00392 float sum = 0; 00393 for (int j = 0; j < 10; j++) 00394 { 00395 sum += temp[j]; 00396 } 00397 float avg = sum/10; 00398 if (avg < 70) 00399 { 00400 blinkOn(); 00401 wait_ms(100); 00402 blinkOff(); 00403 wait_ms(100); 00404 blinkOn(); 00405 wait_ms(100); 00406 blinkOff(); 00407 wait_ms(100); 00408 blinkOn(); 00409 wait_ms(100); 00410 blinkOff(); 00411 wait_ms(100); 00412 00413 } 00414 } 00415 00416 } 00417 00418
Generated on Mon Jul 25 2022 18:36:17 by
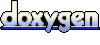