
working demo, with iPhone support
Dependencies: NeoPixels NeoStrip mbed
Fork of NeoPixels by
main.cpp
00001 #include "mbed.h" 00002 #include "NeoStrip.h" 00003 #include "text.h" 00004 #define N 128 00005 #define PATTERNS 4 00006 #include <string> 00007 #include "helper.h" 00008 00009 00010 NeoStrip strip(p18, N); 00011 00012 //callback function for incoming serial communication 00013 void callback(); 00014 //necessary functions for distanceDemo and bikeDemo 00015 void blinkOn(); 00016 void blinkOff(); 00017 void patternLeft(); 00018 void patternRight(); 00019 void patternStop(); 00020 void patternNone(); 00021 void distanceDemo(); 00022 void rainBow(); 00023 void emojiDemo(); 00024 //necessary functions for Animation Demo 00025 void fillBlue(); 00026 void progressBar(bool); 00027 void putChar(int row, int col, char ch, int color); 00028 void putString(std::string); 00029 void loopAscii(); 00030 void MarioBox(); 00031 void drawBox(int row);void emptyBox(int row); 00032 void putCharV(int row, int col, char ch, int color); 00033 int getIndex(int r, int c); 00034 00035 00036 00037 //hardware initilization 00038 Serial device(p13,p14); 00039 AnalogIn ir(p20); 00040 Serial pc(USBTX,USBRX); 00041 00042 //variable initilization 00043 Timer t; 00044 int chars[128]; 00045 std::string emoji[9] = {"*_*", "^_^", "$_$", ">_<", "=_=", "+_=", ":)", ":(", ":3"}; 00046 void (*patterns[])(void) = {&patternLeft, &patternRight, &patternStop, &patternNone}; 00047 int bikeDir; 00048 char ch = 'A'; 00049 00050 int main() 00051 { 00052 // std::string emoji[9] = {"*_*", "^_^", "$_$", ">_<", "=_=", "+_=", ":)", ":(", ":3"}; 00053 float bright = 0.2; // 20% is plenty for indoor use 00054 strip.setBrightness(bright); // set default brightness 00055 00056 //setup serial device 00057 device.baud(57600); 00058 device.attach(&callback); 00059 00060 //wait until phone is connected 00061 while(1){ 00062 if(device.readable()) break; 00063 } 00064 pc.printf("Device connected\n"); 00065 00066 //ready for commands, clear serael buffer 00067 while(device.readable()) device.getc(); 00068 pc.printf("Device ready for commands;\n"); 00069 //phone is disconnected, flash red light warning 00070 while(1){ 00071 if(t.read_ms()>3000){ 00072 pc.printf("time passed i %d\n",t.read_ms()); 00073 blinkOn(); 00074 //progressBar(true); 00075 wait_ms(200); 00076 blinkOff(); 00077 // wait_ms(200); 00078 } 00079 wait(5); 00080 } 00081 00082 00083 00084 } 00085 00086 00087 void callback(){ 00088 pc.printf("new commands arrive \n"); 00089 t.reset(); 00090 switch(device.getc()){ 00091 00092 case PhoneDemo: 00093 pc.printf("Lost Phone Demo\n"); 00094 while(device.readable()) device.getc(); 00095 //reply ack 00096 device.putc('P'); 00097 t.start(); 00098 break; 00099 00100 case MusicDemo: 00101 pc.printf("Music Demo\n"); 00102 rainBow(); 00103 //progressBar(true); 00104 //progressBar(false); 00105 break; 00106 00107 case DistanceDemo: 00108 pc.printf("Distance Demo\n"); 00109 device.putc('D'); 00110 distanceDemo(); 00111 break; 00112 00113 case AnimationOneDemo: 00114 MarioBox(); 00115 break; 00116 00117 case AnimationTwoDemo: 00118 pc.printf("Emoji Demo\n"); 00119 emojiDemo(); 00120 break; 00121 00122 case AnimationThreeDemo: 00123 pc.printf("ascii demo\n"); 00124 loopAscii(); 00125 break; 00126 00127 case LEFT: 00128 pc.printf("left arrow\n"); 00129 patternLeft(); 00130 break; 00131 00132 case RIGHT: 00133 pc.printf("right arrow\n"); 00134 00135 patternRight(); 00136 break; 00137 00138 case STOP: 00139 pc.printf("stop\n"); 00140 00141 patternStop(); 00142 break; 00143 00144 default: 00145 break; 00146 } 00147 00148 00149 } 00150 00151 00152 00153 00154 00155 00156 00157 void fillBlue(){ 00158 for(int i = 0; i < N; i++){ 00159 chars[i] = 0x122446; 00160 } 00161 } 00162 00163 void putChar(int row, int col, char ch, int color){ 00164 for(int r = 0; r < 8; r++){ 00165 for(int c = 0; c < 6; c++){ 00166 if(fontdata_6x8[ch * 48 + r * 6 +c]){ 00167 int idx = getIndex(row+r, col+c); 00168 if(idx != -1) 00169 chars[idx] = color; 00170 } 00171 } 00172 } 00173 } 00174 void putCharV(int row, int col, char ch, int color){ 00175 for(int r = 0; r < 8; r++){ 00176 for(int c = 0; c < 6; c++){ 00177 if(fontdata_6x8[ch * 48 + r * 6 +c]){ 00178 int idx = (row + r) *8 +( col +c); 00179 if(idx != -1) 00180 chars[idx] = color; 00181 } 00182 } 00183 } 00184 } 00185 00186 void MarioBox(){ 00187 int color = 0xffff00; 00188 // int red = 0xff0000; 00189 00190 drawBox(8); 00191 strip.setPixels(0, N, chars); 00192 strip.write(); 00193 wait(5); 00194 00195 for(int i = 1; i < 3; i++){ 00196 fillBlue(); 00197 drawBox(8-i); 00198 strip.setPixels(0, N, chars); 00199 strip.write(); 00200 wait(0.3); 00201 } 00202 for(int i = 0; i < 3; i++){ 00203 fillBlue(); 00204 drawBox(6 + i); 00205 strip.setPixels(0, N, chars); 00206 strip.write(); 00207 wait(0.3); 00208 } 00209 fillBlue(); 00210 emptyBox(8); 00211 putChar(0,0,'0',color); 00212 strip.setPixels(0, N, chars); 00213 strip.write(); 00214 wait(5); 00215 strip.clear(); 00216 strip.write(); 00217 00218 } 00219 void putString(std::string str){ 00220 int color = 0xffff00; 00221 for(int i = 0; i < str.length(); ++i){ 00222 putChar(0,5*i,str[i], color); 00223 strip.setPixels(0, N, chars); 00224 } 00225 strip.write(); 00226 wait_ms(100); 00227 } 00228 00229 void loopAscii(){ 00230 int color = 0xffff00; 00231 char ch = 'A'; 00232 int count = 50; 00233 00234 for(int i = 0; i < count; i+=2) { 00235 putChar(0,0,ch+i,color); 00236 putChar(0,7,ch+i+1,color); 00237 strip.setPixels(0, N, chars); 00238 strip.write(); 00239 wait_ms(200); 00240 } 00241 00242 } 00243 00244 void drawBox(int row){ 00245 for(int i = 0; i < 64; ++i) 00246 chars[row*8 + i] = 0x000000; 00247 putCharV(row,0,'?',0xffff00); 00248 } 00249 void emptyBox(int row){ 00250 for(int i = 0; i < 64; ++i) 00251 chars[row*8 + i] = 0x000000; 00252 } 00253 void progressBar(bool up){ 00254 int index; 00255 int init; 00256 int increment; 00257 int color; 00258 if(up == true){ 00259 init = 0; 00260 increment = 1; 00261 color = 0x122446; 00262 }else { 00263 init = 15; 00264 increment = -1; 00265 fillBlue(); 00266 color = 0x000000; 00267 } 00268 00269 for(int x = 0; x < 16 ; x++){ 00270 00271 for (int j = 0; j < 8; j++){ 00272 index = getIndex(j,init + increment * x); 00273 chars[index] = color; 00274 } 00275 strip.setPixels(0, N, chars); 00276 strip.write(); 00277 wait_ms(200); 00278 } 00279 } 00280 00281 00282 void rainBow(){ 00283 int index; 00284 unsigned int black = 0x000000; 00285 unsigned int color[7] = {0x5b00ff,0xff0d19, 0xf0ff03, 0x17ff29, 0x1fffee,0x0d65ff, 0xfb12ff}; 00286 int init = 0; 00287 int increment = 1; 00288 00289 for(int x = 0; x < 16 ; x++){ 00290 for (int j = 0; j < 8; j++){ 00291 index = getIndex(j,init + increment * x); 00292 chars[index] = color[index%7]; 00293 } 00294 strip.setPixels(0, N, chars); 00295 strip.write(); 00296 wait_ms(200); 00297 } 00298 00299 for(int x = 15; x >= 0 ; x--){ 00300 for (int j = 0; j < 8; j++){ 00301 index = getIndex(j,init + increment * x); 00302 chars[index] = black; 00303 } 00304 00305 strip.setPixels(0, N, chars); 00306 strip.write(); 00307 wait_ms(200); 00308 } 00309 00310 } 00311 00312 00313 00314 void patternLeft() 00315 { 00316 for(int j=0;j<3;j++){ 00317 for (int i = 0; i < N; i++) 00318 { 00319 if (maskLeft128[i] == 1) 00320 chars[i]=0x00FF00; 00321 else 00322 chars[i]=0x000000; 00323 } 00324 00325 strip.setPixels(0, N, chars); 00326 strip.write(); 00327 wait_ms(200); 00328 strip.clear(); 00329 strip.write(); 00330 wait_ms(200); 00331 00332 } 00333 } 00334 00335 void patternRight() 00336 { 00337 for(int j=0;j<3;j++){ 00338 for (int i = 0; i < N; i++) 00339 { 00340 if (maskRight128[i] == 1) 00341 chars[i]=0x00FF00; 00342 else 00343 chars[i]=0x000000; 00344 } 00345 strip.setPixels(0, N, chars); 00346 strip.write(); 00347 wait_ms(200); 00348 strip.clear(); 00349 strip.write(); 00350 wait_ms(200); 00351 00352 } 00353 00354 } 00355 00356 void patternStop() 00357 { 00358 for(int j=0;j<3;j++){ 00359 for (int i = 0; i < N; i++) 00360 { 00361 if (maskStop128[i] == 1) 00362 chars[i]=0xFF0000; 00363 else 00364 chars[i]=0x000000; 00365 } 00366 strip.setPixels(0, N, chars); 00367 strip.write(); 00368 wait_ms(200); 00369 strip.clear(); 00370 strip.write(); 00371 wait_ms(200); 00372 00373 } 00374 00375 } 00376 00377 void patternNone() 00378 { 00379 strip.clear(); 00380 } 00381 00382 00383 00384 void blinkOn() 00385 { 00386 strip.clear(); 00387 for (int i = 0; i < N; i++) 00388 { 00389 chars[i]=0xFF0000; 00390 } 00391 00392 strip.setPixels(0, N, chars); 00393 strip.write(); 00394 wait_ms(200); 00395 } 00396 00397 void blinkOff() 00398 { 00399 strip.clear(); 00400 for (int i = 0; i < N; i++) 00401 { 00402 chars[i]=0x000000; 00403 } 00404 strip.setPixels(0, N, chars); 00405 strip.write(); 00406 wait_ms(200); 00407 } 00408 00409 00410 00411 void distanceDemo(){ 00412 float val = 21/ir; 00413 pc.printf("dist avg=%f\n",val); 00414 00415 if(val<70) { 00416 blinkOn(); 00417 wait_ms(200); 00418 blinkOff(); 00419 } 00420 00421 } 00422 00423 void emojiDemo(){ 00424 for(int i=0;i<8;i++){ 00425 putString(emoji[i]); 00426 wait_ms(200); 00427 strip.write(); 00428 wait_ms(200); 00429 strip.clear(); 00430 strip.write(); 00431 wait_ms(200); 00432 } 00433 } 00434 00435 00436 int getIndex(int r, int c){ 00437 if(c < 8){ 00438 return (r * 8 + c); 00439 }else if(c > 15){ 00440 return -1; 00441 } 00442 else{ 00443 return (64 + r * 8 + (c-8)); 00444 } 00445 }
Generated on Wed Jul 13 2022 02:49:39 by
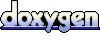