Llibrary for the WiGo MPL3115A2, I2C Precision Altimeter sensor. This is a temp fork
Dependents: sensor AerCloud_MutliTech_Socket_Modem_Example Freescale_Multi-Sensor_Shield 2lemetry_Sensor_Example ... more
Fork of MPL3115A2 by
MPL3115A2.h
00001 #ifndef MPL3115A2_H 00002 #define MPL3115A2_H 00003 00004 #include "mbed.h" 00005 00006 // Oversampling value and minimum time between sample 00007 #define OVERSAMPLE_RATIO_1 0 // 6 ms 00008 #define OVERSAMPLE_RATIO_2 1 // 10 ms 00009 #define OVERSAMPLE_RATIO_4 2 // 18 ms 00010 #define OVERSAMPLE_RATIO_8 3 // 34 ms 00011 #define OVERSAMPLE_RATIO_16 4 // 66 ms 00012 #define OVERSAMPLE_RATIO_32 5 // 130 ms 00013 #define OVERSAMPLE_RATIO_64 6 // 258 ms 00014 #define OVERSAMPLE_RATIO_128 7 // 512 ms 00015 00016 /* Mode */ 00017 #define ALTIMETER_MODE 1 00018 #define BAROMETRIC_MODE 2 00019 00020 /** 00021 * MPL3115A2 Altimeter example 00022 * 00023 * @code 00024 * #include "mbed.h" 00025 * #include "MPL3115A2.h" 00026 * 00027 * #define MPL3115A2_I2C_ADDRESS (0x60<<1) 00028 * MPL3115A2 wigo_sensor1(PTE0, PTE1, MPL3115A2_I2C_ADDRESS); 00029 * Serial pc(USBTX, USBRX); 00030 * 00031 * // pos [0] = altitude or pressure value 00032 * // pos [1] = temperature value 00033 * float sensor_data[2]; 00034 * 00035 * int main(void) { 00036 * 00037 * pc.baud( 230400); 00038 * pc.printf("MPL3115A2 Altimeter mode. [%d]\r\n", wigo_sensor1.getDeviceID()); 00039 * 00040 * wigo_sensor1.Oversample_Ratio( OVERSAMPLE_RATIO_32); 00041 * 00042 * while(1) { 00043 * // 00044 * if ( wigo_sensor1.isDataAvailable()) { 00045 * wigo_sensor1.getAllData( &sensor_data[0]); 00046 * pc.printf("\tAltitude: %f\tTemperature: %f\r\n", sensor_data[0], sensor_data[1]); 00047 * } 00048 * // 00049 * wait( 0.001); 00050 * } 00051 * 00052 * } 00053 * @endcode 00054 */ 00055 class MPL3115A2 00056 { 00057 public: 00058 /** 00059 * MPL3115A2 constructor 00060 * 00061 * @param sda SDA pin 00062 * @param sdl SCL pin 00063 * @param addr addr of the I2C peripheral 00064 * @param int1 InterruptIn 00065 * @param int2 InterruptIn 00066 * 00067 * Interrupt schema: 00068 * 00069 * * The Altitude Trigger use the IRQ1 - Altitude Trigger -> MPL3115A2_Int1.fall -> AltitudeTrg_IRQ -> MPL3115A2_usr1_fptr 00070 * 00071 * * The Data ready use the IRQ2 - Data Ready -> MPL3115A2_Int2.fall -> DataReady_IRQ -> MPL3115A2_usr2_fptr 00072 */ 00073 MPL3115A2(PinName sda, PinName scl, int addr, PinName int1, PinName int2); 00074 00075 /** 00076 * Get the value of the WHO_AM_I register 00077 * 00078 * @returns DEVICE_ID value == 0xC4 00079 */ 00080 uint8_t getDeviceID(); 00081 00082 /** 00083 * Return the STATUS register value 00084 * 00085 * @returns STATUS register value 00086 */ 00087 unsigned char getStatus( void); 00088 00089 /** 00090 * Get the altimeter value 00091 * 00092 * @returns altimeter value as float 00093 */ 00094 float getAltimeter( void); 00095 00096 /** 00097 * Get the altimeter value in raw mode 00098 * 00099 * @param dt pointer to unsigned char array 00100 * @returns 1 if data are available, 0 if not. 00101 */ 00102 unsigned int getAltimeterRaw( unsigned char *dt); 00103 00104 /** 00105 * Get the pressure value 00106 * 00107 * @returns pressure value as float 00108 */ 00109 float getPressure( void); 00110 00111 /** 00112 * Get the pressure value in raw mode 00113 * 00114 * @param dt pointer to unsigned char array 00115 * @returns 1 if data are available, 0 if not. 00116 */ 00117 unsigned int getPressureRaw( unsigned char *dt); 00118 00119 /** 00120 * Get the temperature value 00121 * 00122 * @returns temperature value as float 00123 */ 00124 float getTemperature( void); 00125 00126 /** 00127 * Get the temperature value in raw mode 00128 * 00129 * @param dt pointer to unsigned char array 00130 * @returns 1 if data are available, 0 if not. 00131 */ 00132 unsigned int getTemperatureRaw( unsigned char *dt); 00133 00134 /** 00135 * Set the Altimeter Mode 00136 * 00137 * @returns none 00138 */ 00139 void Altimeter_Mode( void); 00140 00141 /** 00142 * Set the Barometric Mode 00143 * 00144 * @returns none 00145 */ 00146 void Barometric_Mode( void); 00147 00148 /** 00149 * Get the altimeter or pressure and temperature values 00150 * 00151 * @param array of float f[2] 00152 * @returns 0 no data available, 1 for data available 00153 */ 00154 unsigned int getAllData( float *f); 00155 00156 /** 00157 * Get the altimeter or pressure and temperature values and the delta values 00158 * 00159 * @param array of float f[2], array of float d[2] 00160 * @returns 0 no data available, 1 for data available 00161 */ 00162 unsigned int getAllData( float *f, float *d); 00163 00164 /** 00165 * Get the altimeter or pressure and temperature captured maximum value 00166 * 00167 * @param array of float f[2] 00168 * @returns 0 no data available, 1 for data available 00169 */ 00170 void getAllMaximumData( float *f); 00171 00172 /** 00173 * Get the altimeter or pressure and temperature captured minimum value 00174 * 00175 * @param array of float f[2] 00176 * @returns 0 no data available, 1 for data available 00177 */ 00178 void getAllMinimumData( float *f); 00179 00180 /** 00181 * Get the altimeter or pressure, and temperature values in raw mode 00182 * 00183 * @param array of unsigned char[5] 00184 * @returns 1 if data are available, 0 if not. 00185 */ 00186 unsigned int getAllDataRaw( unsigned char *dt); 00187 00188 /** 00189 * Return if there are date available 00190 * 00191 * @return 0 for no data available, bit0 set for Temp data available, bit1 set for Press/Alti data available 00192 * bit2 set for both Temp and Press/Alti data available 00193 */ 00194 unsigned int isDataAvailable( void); 00195 00196 /** 00197 * Set the oversampling rate value 00198 * 00199 * @param oversampling values. See MPL3115A2.h 00200 * @return none 00201 */ 00202 void Oversample_Ratio( unsigned int ratio); 00203 00204 /** 00205 * Configure the sensor to streaming data using Interrupt 00206 * 00207 * @param user functin callback, oversampling values. See MPL3115A2.h 00208 * @return none 00209 */ 00210 void DataReady( void(*fptr)(void), unsigned char OS); 00211 00212 /** 00213 * Configure the sensor to generate an Interrupt crossing the center threshold 00214 * 00215 * @param user functin callback, level in meter 00216 * @return none 00217 */ 00218 void AltitudeTrigger( void(*fptr)(void), unsigned short level); 00219 00220 /** 00221 * Soft Reset 00222 * 00223 * @param none 00224 * @return none 00225 */ 00226 void Reset( void); 00227 00228 /** 00229 * Configure the Pressure offset. 00230 * Pressure user accessible offset trim value expressed as an 8-bit 2's complement number. 00231 * The user offset registers may be adjusted to enhance accuracy and optimize the system performance. 00232 * Range is from -512 to +508 Pa, 4 Pa per LSB. 00233 * In RAW output mode no scaling or offsets will be applied in the digital domain 00234 * 00235 * @param offset 00236 * @return none 00237 */ 00238 void SetPressureOffset( char offset); 00239 00240 /** 00241 * Configure the Temperature offset. 00242 * Temperature user accessible offset trim value expressed as an 8-bit 2's complement number. 00243 * The user offset registers may be adjusted to enhance accuracy and optimize the system performance. 00244 * Range is from -8 to +7.9375°C 0.0625°C per LSB. 00245 * In RAW output mode no scaling or offsets will be applied in the digital domain 00246 * 00247 * @param offset 00248 * @return none 00249 */ 00250 void SetTemperatureOffset( char offset); 00251 00252 /** 00253 * Configure the Altitude offset. 00254 * Altitude Data User Offset Register (OFF_H) is expressed as a 2’s complement number in meters. 00255 * The user offset register provides user adjustment to the vertical height of the Altitude output. 00256 * The range of values are from -128 to +127 meters. 00257 * In RAW output mode no scaling or offsets will be applied in the digital domain 00258 * 00259 * @param offset 00260 * @return none 00261 */ 00262 void SetAltitudeOffset( char offset); 00263 00264 private: 00265 I2C m_i2c; 00266 int m_addr; 00267 InterruptIn MPL3115A2_Int1; 00268 InterruptIn MPL3115A2_Int2; 00269 00270 unsigned char MPL3115A2_mode; 00271 unsigned char MPL3115A2_oversampling; 00272 void DataReady_IRQ( void); 00273 void AltitudeTrg_IRQ( void); 00274 00275 /** Set the device in active mode 00276 */ 00277 void Active( void); 00278 00279 /** Set the device in standby mode 00280 */ 00281 void Standby( void); 00282 00283 /** Get the altimiter value from the sensor. 00284 * 00285 * @param reg the register from which read the data. 00286 * Can be: REG_ALTIMETER_MSB for altimeter value 00287 * REG_ALTI_MIN_MSB for the minimum value captured 00288 * REG_ALTI_MAX_MSB for the maximum value captured 00289 */ 00290 float getAltimeter( unsigned char reg); 00291 00292 /** Get the pressure value from the sensor. 00293 * 00294 * @param reg the register from which read the data. 00295 * Can be: REG_PRESSURE_MSB for altimeter value 00296 * REG_PRES_MIN_MSB for the minimum value captured 00297 * REG_PRES_MAX_MSB for the maximum value captured 00298 */ 00299 float getPressure( unsigned char reg); 00300 00301 /** Get the altimiter value from the sensor. 00302 * 00303 * @param reg the register from which read the data. 00304 * Can be: REG_TEMP_MSB for altimeter value 00305 * REG_TEMP_MIN_MSB for the minimum value captured 00306 * REG_TEMP_MAX_MSB for the maximum value captured 00307 */ 00308 float getTemperature( unsigned char reg); 00309 00310 void readRegs(int addr, uint8_t * data, int len); 00311 void writeRegs(uint8_t * data, int len); 00312 00313 }; 00314 00315 #endif
Generated on Tue Jul 12 2022 18:14:51 by
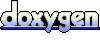