
V.06 11/3
Dependencies: FT6206 SDFileSystem SPI_TFT_ILI9341 TFT_fonts
Fork of ATT_AWS_IoT_demo by
aws_iot_shadow_json_data.h
00001 /* 00002 * Copyright 2010-2015 Amazon.com, Inc. or its affiliates. All Rights Reserved. 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"). 00005 * You may not use this file except in compliance with the License. 00006 * A copy of the License is located at 00007 * 00008 * http://aws.amazon.com/apache2.0 00009 * 00010 * or in the "license" file accompanying this file. This file is distributed 00011 * on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either 00012 * express or implied. See the License for the specific language governing 00013 * permissions and limitations under the License. 00014 */ 00015 00016 #ifndef SRC_SHADOW_AWS_IOT_SHADOW_JSON_DATA_H_ 00017 #define SRC_SHADOW_AWS_IOT_SHADOW_JSON_DATA_H_ 00018 00019 /** 00020 * @file aws_iot_shadow_json_data.h 00021 * @brief This file is the interface for all the Shadow related JSON functions. 00022 */ 00023 00024 #include <stddef.h> 00025 00026 /** 00027 * @brief This is a static JSON object that could be used in code 00028 * 00029 */ 00030 typedef struct jsonStruct jsonStruct_t; 00031 /** 00032 * @brief Every JSON name value can have a callback. The callback should follow this signature 00033 */ 00034 typedef void (*jsonStructCallback_t)(const char *pJsonValueBuffer, uint32_t valueLength, jsonStruct_t *pJsonStruct_t); 00035 00036 /** 00037 * @brief All the JSON object types enum 00038 * 00039 * JSON number types need to be split into proper integer / floating point data types and sizes on embedded platforms. 00040 */ 00041 typedef enum { 00042 SHADOW_JSON_INT32, 00043 SHADOW_JSON_INT16, 00044 SHADOW_JSON_INT8, 00045 SHADOW_JSON_UINT32, 00046 SHADOW_JSON_UINT16, 00047 SHADOW_JSON_UINT8, 00048 SHADOW_JSON_FLOAT, 00049 SHADOW_JSON_DOUBLE, 00050 SHADOW_JSON_BOOL, 00051 SHADOW_JSON_STRING, 00052 SHADOW_JSON_OBJECT 00053 } JsonPrimitiveType; 00054 00055 /** 00056 * @brief This is the struct form of a JSON Key value pair 00057 */ 00058 struct jsonStruct { 00059 const char *pKey; ///< JSON key 00060 void *pData; ///< pointer to the data (JSON value) 00061 JsonPrimitiveType type; ///< type of JSON 00062 jsonStructCallback_t cb; ///< callback to be executed on receiving the Key value pair 00063 }; 00064 00065 /** 00066 * @brief Initialize the JSON document with Shadow expected name/value 00067 * 00068 * This Function will fill the JSON Buffer with a null terminated string. Internally it uses snprintf 00069 * This function should always be used First, followed by iot_shadow_add_reported and/or iot_shadow_add_desired. 00070 * Always finish the call sequence with iot_finalize_json_document 00071 * 00072 * @note Ensure the size of the Buffer is enough to hold the entire JSON Document. 00073 * 00074 * 00075 * @param pJsonDocument The JSON Document filled in this char buffer 00076 * @param maxSizeOfJsonDocument maximum size of the pJsonDocument that can be used to fill the JSON document 00077 * @return An IoT Error Type defining if the buffer was null or the entire string was not filled up 00078 */ 00079 IoT_Error_t aws_iot_shadow_init_json_document(char *pJsonDocument, size_t maxSizeOfJsonDocument); 00080 00081 /** 00082 * @brief Add the reported section of the JSON document of jsonStruct_t 00083 * 00084 * This is a variadic function and please be careful with the usage. count is the number of jsonStruct_t types that you would like to add in the reported section 00085 * This function will add "reported":{<all the values that needs to be added>} 00086 * 00087 * @note Ensure the size of the Buffer is enough to hold the reported section + the init section. Always use the same JSON document buffer used in the iot_shadow_init_json_document function. This function will accommodate the size of previous null terminated string, so pass teh max size of the buffer 00088 * 00089 * 00090 * @param pJsonDocument The JSON Document filled in this char buffer 00091 * @param maxSizeOfJsonDocument maximum size of the pJsonDocument that can be used to fill the JSON document 00092 * @param count total number of arguments(jsonStruct_t object) passed in the arguments 00093 * @return An IoT Error Type defining if the buffer was null or the entire string was not filled up 00094 */ 00095 IoT_Error_t aws_iot_shadow_add_reported(char *pJsonDocument, size_t maxSizeOfJsonDocument, uint8_t count, ...); 00096 00097 /** 00098 * @brief Add the desired section of the JSON document of jsonStruct_t 00099 * 00100 * This is a variadic function and please be careful with the usage. count is the number of jsonStruct_t types that you would like to add in the reported section 00101 * This function will add "desired":{<all the values that needs to be added>} 00102 * 00103 * @note Ensure the size of the Buffer is enough to hold the reported section + the init section. Always use the same JSON document buffer used in the iot_shadow_init_json_document function. This function will accommodate the size of previous null terminated string, so pass the max size of the buffer 00104 * 00105 * 00106 * @param pJsonDocument The JSON Document filled in this char buffer 00107 * @param maxSizeOfJsonDocument maximum size of the pJsonDocument that can be used to fill the JSON document 00108 * @param count total number of arguments(jsonStruct_t object) passed in the arguments 00109 * @return An IoT Error Type defining if the buffer was null or the entire string was not filled up 00110 */ 00111 IoT_Error_t aws_iot_shadow_add_desired(char *pJsonDocument, size_t maxSizeOfJsonDocument, uint8_t count, ...); 00112 00113 /** 00114 * @brief Finalize the JSON document with Shadow expected client Token. 00115 * 00116 * This function will automatically increment the client token every time this function is called. 00117 * 00118 * @note Ensure the size of the Buffer is enough to hold the entire JSON Document. If the finalized section is not invoked then the JSON doucment will not be valid 00119 * 00120 * 00121 * @param pJsonDocument The JSON Document filled in this char buffer 00122 * @param maxSizeOfJsonDocument maximum size of the pJsonDocument that can be used to fill the JSON document 00123 * @return An IoT Error Type defining if the buffer was null or the entire string was not filled up 00124 */ 00125 IoT_Error_t aws_iot_finalize_json_document(char *pJsonDocument, size_t maxSizeOfJsonDocument); 00126 00127 /** 00128 * @brief Fill the given buffer with client token for tracking the Repsonse. 00129 * 00130 * This function will add the AWS_IOT_MQTT_CLIENT_ID with a sequence number. Every time this function is used the sequence number gets incremented 00131 * 00132 * 00133 * @param pBufferToBeUpdatedWithClientToken buffer to be updated with the client token string 00134 * @param maxSizeOfJsonDocument maximum size of the pBufferToBeUpdatedWithClientToken that can be used 00135 * @return An IoT Error Type defining if the buffer was null or the entire string was not filled up 00136 */ 00137 00138 IoT_Error_t aws_iot_fill_with_client_token(char *pBufferToBeUpdatedWithClientToken, size_t maxSizeOfJsonDocument); 00139 00140 #endif /* SRC_SHADOW_AWS_IOT_SHADOW_JSON_DATA_H_ */ 00141
Generated on Tue Jul 12 2022 14:16:19 by
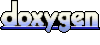