
STmicro HAL driver DMA.
Embed:
(wiki syntax)
Show/hide line numbers
mx.c
00001 /* 00002 * mx.c 00003 * 00004 * Created on: 2019/04/06 00005 * Author: aruaru 00006 */ 00007 #include <stdlib.h> 00008 #include "mx/main.h" 00009 #include "mx/usart.h" 00010 #include "add/mx.h" 00011 00012 #define TX_BUFFER_SIZE 4000 00013 #define RX_BUFFER_SIZE 40 00014 00015 extern DMA_HandleTypeDef hdma_usart2_rx; 00016 extern DMA_HandleTypeDef hdma_usart2_tx; 00017 00018 static char txbuffer[TX_BUFFER_SIZE]; 00019 static uint8_t rxbuf[RX_BUFFER_SIZE]; 00020 typedef struct{ 00021 uint8_t *b; 00022 size_t size; 00023 int pos; 00024 }RxBuffer_t; 00025 RxBuffer_t rxBuffer; 00026 00027 /*================================= 00028 * 以下はstm32l4xx_it.cから 00029 ==================================*/ 00030 00031 00032 /** 00033 * @brief This function handles DMA1 channel6 global interrupt. 00034 */ 00035 00036 /** 00037 * @brief This function handles DMA1 channel6 global interrupt. 00038 */ 00039 void DMA1_Channel6_IRQHandler(void) 00040 { 00041 /* USER CODE BEGIN DMA1_Channel6_IRQn 0 */ 00042 00043 /* USER CODE END DMA1_Channel6_IRQn 0 */ 00044 HAL_DMA_IRQHandler(&hdma_usart2_rx); 00045 /* USER CODE BEGIN DMA1_Channel6_IRQn 1 */ 00046 00047 /* USER CODE END DMA1_Channel6_IRQn 1 */ 00048 } 00049 /** 00050 * @brief This function handles DMA1 channel7 global interrupt. 00051 */ 00052 void DMA1_Channel7_IRQHandler(void) 00053 { 00054 00055 HAL_DMA_IRQHandler(&hdma_usart2_tx); 00056 00057 static int8_t endflag = 0; 00058 if(endflag != 0){//2回目のコール 00059 huart2.gState=HAL_UART_STATE_READY; 00060 endflag = 0; 00061 }else{ 00062 endflag++; 00063 } 00064 00065 } 00066 00067 00068 /* 00069 * dmaを利用するための関数 00070 */ 00071 00072 /* 00073 * ローカルのバッファーにコピー後に出力 00074 * 呼び出し元のバッファはなくなる可能性がある 00075 */ 00076 HAL_StatusTypeDef printUart(char* buf,int num){ 00077 HAL_StatusTypeDef status=HAL_ERROR; 00078 if(hdma_usart2_tx.Instance->CNDTR!=0){ 00079 return HAL_BUSY; 00080 } 00081 for(int i=0;i<num && i<4000 ;i++){ 00082 *(txbuffer+i)=*buf++; 00083 } 00084 00085 status = HAL_UART_Transmit_DMA(&huart2, (uint8_t*)txbuffer, num); 00086 00087 return status; 00088 } 00089 HAL_StatusTypeDef startRxUart(void){ 00090 00091 HAL_StatusTypeDef status; 00092 00093 rxBuffer.size=RX_BUFFER_SIZE; 00094 rxBuffer.b = (uint8_t*)malloc(sizeof(char)*rxBuffer.size); 00095 if(rxBuffer.b==0)return HAL_ERROR; 00096 rxBuffer.pos = 0; 00097 00098 status = HAL_UART_Receive_DMA(&huart2, rxBuffer.b, rxBuffer.size); 00099 return status; 00100 } 00101 int getChar(char* c){ 00102 if(readable()>0){ 00103 *c=*(rxBuffer.b+rxBuffer.pos++); 00104 rxBuffer.pos %= rxBuffer.size; 00105 return 1; 00106 }else{ 00107 return 0; 00108 } 00109 } 00110 int readable(void){ 00111 int cndtr,pos; 00112 cndtr = hdma_usart2_rx.Instance->CNDTR; 00113 pos = rxBuffer.size - cndtr; 00114 if( pos != rxBuffer.pos ){ 00115 return 1; 00116 }else{ 00117 return 0; 00118 } 00119 } 00120 int readCndtr(void){ 00121 return (int) hdma_usart2_rx.Instance->CNDTR; 00122 } 00123 int readPos(void){ 00124 return rxBuffer.pos; 00125 }
Generated on Fri Jul 15 2022 23:04:23 by
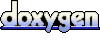