
回転数計のクラスです。
Dependencies: mbed
Fork of test_cadenceClass by
Cadence.h
00001 //;2648 ;00 00000 0;168 ;001; 10043 c1;31 10;00 00;00 00;10 63;08 39;I; -012; 0002; 0104;\r\n 00002 //;109 ;00 00000 0;123 ;011; 10008 e4;27 95;00 00;00 00;13 99;08 51;G; -068; -096; -192; 00003 #ifndef CADENCE_H 00004 #define CADENCE_H 00005 00006 #include "mbed.h" 00007 #include <string> 00008 DigitalOut led3(LED3); 00009 class Cadence : public RawSerial{ 00010 private: 00011 static const int DATAS_NUM = 75; 00012 00013 protected: 00014 00015 public: 00016 string strC,strV; 00017 char data[DATAS_NUM]; 00018 string strData; 00019 int data_count, data_num; 00020 double cadence, voltage; 00021 Cadence(PinName tx, PinName rx, const char* name = NULL) : RawSerial(tx, rx){ 00022 for(int i=0;i<DATAS_NUM;i++) data[i]= NULL; 00023 data_num=0; 00024 data_count=0; 00025 baud(115200); 00026 cadence=0; 00027 voltage=0; 00028 } 00029 int checkInt(const char c[]){ 00030 for(int i = 0; i<strlen(c); i++){ 00031 if( c[0] == '-' ) continue; 00032 if( c[i] - '0' > 9 || c[i] - '0' <0 ) return -1; 00033 }return 1; 00034 } 00035 void readData(){ 00036 if(readable()){ 00037 data_count = 0; 00038 for (int i = 0; i<DATAS_NUM; i++) data[i] = NULL; 00039 do{ 00040 if(readable()) { 00041 data[data_count] = getc(); 00042 if(data[data_count] != '\n') data_count++; 00043 led3 = !led3; 00044 } 00045 }while(data[data_count] != '\n' && data_count<DATAS_NUM); 00046 strData = data; 00047 if( int strlength = strData.length() > DATAS_NUM-4 ){ 00048 // for(int i = 0; i<4; i++){ 00049 // strC += data[data_count-6+i]; 00050 // strV += data[data_count-43+i]; 00051 // } 00052 // if( checkInt(strC) ) sscanf(strC,"%lf",&cadence); 00053 // if( checkInt(strV) ) sscanf(strV,"%lf",&voltage); 00054 00055 switch (strData.length()){ 00056 case DATAS_NUM-3 : 00057 strData.erase(0,2); 00058 break; 00059 case DATAS_NUM-2 : 00060 strData.erase(0,3); 00061 break; 00062 case DATAS_NUM-1 : 00063 strData.erase(0,4); 00064 break; 00065 case DATAS_NUM : 00066 strData.erase(0,5); 00067 } 00068 strV = strData.substr(27,4); 00069 strC = strData.substr(64,4); 00070 if( checkInt( strV.c_str() ) ) sscanf(strV.c_str(),"%lf",&voltage); 00071 if( checkInt( strC.c_str() ) ) sscanf(strC.c_str(),"%lf",&cadence); 00072 00073 cadence /= 6.0; 00074 voltage *= 0.001; 00075 } 00076 } 00077 } 00078 }; 00079 #endif
Generated on Mon Jul 18 2022 09:50:56 by
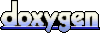