
Ultrasonic Audio File Player
Dependencies: mbed
Fork of TAU_ZOOLOG_Chirp_Generator by
main.cpp
00001 /* 00002 %%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%% 00003 % Generate WAVE Signal - 25/04/2018 % 00004 % Arkadi Rafalovich - % Arkadiraf@gmail.com % 00005 %%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%% 00006 % Updates: 00007 % Disable interrupt during chirp out, fixes systic interrupt delays at 1ms interval for 0.5 us 00008 Pinout: 00009 DAC -- PA_4 -- A2 00010 00011 I/O -- PA_5 -- D13 (Status LED, Condition) 00012 I/O -- PA_6 -- D12 (Toggle Pin, Loop Freq) 00013 Analog PA_0 -- A0 (Potentiometer) 00014 00015 */ 00016 #include "mbed.h" 00017 #include "signal.h" 00018 #define PULSE_RATE 10.0f // in HZ 00019 #define FREQ_POT_EN // Potentiometer to set PULSE Rate 00020 #define MIN_FREQ 0.1f //(HZ) 00021 #define SAMPLE_RATE_375_KHZ // basic sample rate is 1Mhz, this mode adds delay in between sample to meet the audio sample rate 00022 00023 float pulseRate=PULSE_RATE; 00024 // Serial over USB as input device 00025 Serial pc(SERIAL_TX, SERIAL_RX); 00026 00027 // mbed variables, Settings 00028 AnalogOut out(PA_4); 00029 AnalogIn potFreq(A0); 00030 // Potentiometer analog INPUT 00031 00032 // digital pins 00033 DigitalOut led(LED1); 00034 DigitalOut outPulse(PA_6); // Toggle pin, Loop Freq 00035 00036 // User Button as interrupt 00037 DigitalIn mybutton(USER_BUTTON); 00038 00039 //DAC declarations 00040 DAC_HandleTypeDef hdac1; 00041 00042 // Dac Register for direct method of setting DAC value`s 00043 __IO uint32_t Dac_Reg = 0; 00044 00045 // Variables 00046 bool toggle_state=0; 00047 00048 // nop operation 00049 inline void NOP() 00050 { 00051 __ASM volatile ("nop"); // one tick operation, Use to adjust frequency by slowing down the proccess 00052 } 00053 00054 /* DAC1 init function */ 00055 void DAC1_Init(void); 00056 00057 // Main procedure 00058 int main() 00059 { 00060 DAC1_Init(); 00061 00062 HAL_DAC_Start(&hdac1, DAC_CHANNEL_1); 00063 00064 // define Dac Register for direct method of setting DAC value`s 00065 Dac_Reg = (uint32_t) (hdac1.Instance); 00066 Dac_Reg += __HAL_DHR12R1_ALIGNEMENT(DAC_ALIGN_12B_R); 00067 00068 // set outputs 00069 outPulse.write(0); 00070 led.write(0); 00071 // Output value using DAC 00072 // HAL_DAC_SetValue(&hdac1, DAC_CHANNEL_1, DAC_ALIGN_12B_R, ADCValueOut); 00073 *(__IO uint32_t *) Dac_Reg = (uint16_t)(4095/2); 00074 00075 // Infinite loop 00076 while(true) { 00077 if (mybutton.read()==0) { // if button pressed, generate pulse out 00078 led.write(1); 00079 00080 ///////////////////////////////////////////////////////////////////////////////// 00081 __disable_irq(); // Disable Interrupts 00082 // generate chirp out 00083 for (int ii=0; ii<NUM_SAMPLES; ii++) { 00084 // toogle io for loop frequency 00085 toggle_state=!toggle_state; 00086 outPulse.write(toggle_state); 00087 // generate delay for 1MHz Sample rate 00088 for (int jj=0; jj<31; jj++) { 00089 NOP(); 00090 } 00091 // micro nops :) 00092 NOP(); 00093 NOP(); 00094 NOP(); 00095 NOP(); 00096 NOP(); 00097 NOP(); 00098 NOP(); 00099 00100 #ifdef SAMPLE_RATE_375_KHZ 00101 // generate delay 00102 for (int jj=0; jj<61; jj++) { 00103 NOP(); 00104 } 00105 // micro nops :) 00106 //NOP(); 00107 //NOP(); 00108 NOP(); 00109 NOP(); 00110 NOP(); 00111 #endif 00112 // Output value using DAC 00113 // HAL_DAC_SetValue(&hdac1, DAC_CHANNEL_1, DAC_ALIGN_12B_R, ADCValueOut); 00114 *(__IO uint32_t *) Dac_Reg = chirpData[ii]; 00115 } 00116 // Output value using DAC 00117 // HAL_DAC_SetValue(&hdac1, DAC_CHANNEL_1, DAC_ALIGN_12B_R, ADCValueOut); 00118 *(__IO uint32_t *) Dac_Reg = (uint16_t)(4095/2); 00119 __enable_irq(); // Enable Interrupts 00120 ////////////////////////////////////////////////////////////////////////////////// 00121 00122 // generate delay between pulses 00123 // delay post pulse // sets the pulse rate 00124 float waitTime = (1.0f/(2.0f*pulseRate) - (((float)NUM_SAMPLES)/1000000.0f)); 00125 if (waitTime > 0) { 00126 led.write(0); 00127 wait(waitTime); 00128 led.write(1); 00129 wait(1.0f/(2.0f*pulseRate)); 00130 } else { 00131 wait(0.5); 00132 printf("!!! Error Wait time is negative %f !!!\r\n", waitTime); 00133 wait(0.5); 00134 } 00135 } // end button press 00136 led.write(0); 00137 00138 // update freq based on potentiometer 00139 #ifdef FREQ_POT_EN 00140 pulseRate = potFreq * PULSE_RATE; 00141 if (pulseRate < MIN_FREQ) pulseRate = MIN_FREQ; 00142 //printf("Pulse Rate %f\r\n", pulseRate); 00143 #endif 00144 }// end while(True) 00145 } 00146 00147 00148 // init dac 00149 00150 /* DAC1 init function */ 00151 void DAC1_Init(void) 00152 { 00153 DAC_ChannelConfTypeDef sConfig; 00154 00155 // DAC Initialization 00156 hdac1.Instance = DAC; 00157 if(HAL_DAC_Init(&hdac1) != HAL_OK) { 00158 printf("!!! Error in DAC initialization !!!\r\n"); 00159 } 00160 00161 // DAC channel OUT1 config 00162 sConfig.DAC_Trigger = DAC_TRIGGER_NONE; 00163 sConfig.DAC_OutputBuffer = DAC_OUTPUTBUFFER_ENABLE; 00164 if (HAL_DAC_ConfigChannel(&hdac1, &sConfig, DAC_CHANNEL_1) != HAL_OK) { 00165 printf("!!! Error in DAC channel initialization !!!\r\n"); 00166 } 00167 }
Generated on Sun Jul 17 2022 11:34:30 by
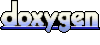