Coap Client and Server
Dependencies: DebugLib EthernetInterface cantcoap mbed-rtos
Fork of yeswecancoap by
client.h
00001 #pragma once 00002 00003 /* 00004 Waarom client per server 00005 Ten eerste moeten van een repons dan niet bepalen voor welke request hij juist was. 00006 Alles dat voor een bepaalde client / socket toekomt is voor die client. 00007 Dat betekent ook dat we niet eeuwig moeten wachten op een respons maar dat we async 00008 kunnen werken en werken met timeouts. We overlopen dan gewoon constant de clients die nog 00009 geen respons hebben gekregen. 00010 */ 00011 00012 #include <string> 00013 #include <vector> 00014 #include "cantcoap.h" 00015 #include "coap.h" 00016 #include "response.h" 00017 #include "request.h" 00018 00019 enum Method; 00020 00021 class Client { 00022 00023 private: 00024 // ResourceRequest holding info of previous request 00025 struct ResourceRequest{ 00026 uint32_t token_id; 00027 char* uri; 00028 void (*response_handler)(Request*, Response*); // Not sure if we need request and respons PDU's 00029 int method; 00030 CoapPDU * req_pdu; 00031 }; 00032 00033 private: 00034 UDPSocket udp_socket; 00035 Endpoint coap_server; // One client per endpoint 00036 uint32_t new_token_id; // Matches requests and responses 00037 uint16_t new_message_id; 00038 std::string identifier; // Identifier is some trivial string used for console output 00039 00040 // List of unhandled previous requests 00041 std::vector<ResourceRequest> requests; 00042 00043 public: 00044 Client(const char * server, const int port, std::string identifier="Client"); 00045 00046 public: 00047 void sendRequest(char* uri, void (*response_handler)(Request*, Response*), CoapPDU::Code method); 00048 void checkForResponse(void); 00049 00050 };
Generated on Wed Jul 13 2022 18:09:57 by
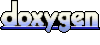