
Program to update the D7A modem's firmware.
Dependencies: modem_ref_helper DebouncedInterrupt
cup.cpp
00001 #include "cup.h" 00002 #include "bin.h" 00003 00004 00005 uint8_t const modem_data[CUP_DATA_SIZE] = CUP_DATA; 00006 uint8_t const bootloader_data[BOOTLOADER_DATA_SIZE] = BOOTLOADER_DATA; 00007 Semaphore modem_cup_ready(0); 00008 00009 cup_param_t const cup_modem = { 00010 .data = (uint8_t*)modem_data, 00011 .cfg_fid = CUP_CFG_FID, 00012 .code_fid = CUP_CODE_FID, 00013 .code_size = CUP_CODE_SIZE, 00014 .data_size = CUP_DATA_SIZE, 00015 .local_mtu = CUP_LOCAL_MTU, 00016 .nb_archives = CUP_NB_ARCHIVES, 00017 .signature = CUP_SIGNATURE, 00018 .mfg_id = CUP_MFG_ID, 00019 .dev_id = CUP_DEV_ID, 00020 .hw_id = CUP_HW_ID, 00021 .fw_major = CUP_FW_MAJOR, 00022 .fw_minor = CUP_FW_MINOR, 00023 .fw_patch = CUP_FW_PATCH, 00024 .fw_hash = CUP_FW_HASH, 00025 .target_fw_major = CUP_TARGET_FW_MAJOR, 00026 .target_fw_minor = CUP_TARGET_FW_MINOR, 00027 }; 00028 00029 cup_param_t const cup_bootloader = { 00030 .data = (uint8_t*)bootloader_data, 00031 .cfg_fid = BOOTLOADER_CFG_FID, 00032 .code_fid = BOOTLOADER_CODE_FID, 00033 .code_size = BOOTLOADER_CODE_SIZE, 00034 .data_size = BOOTLOADER_DATA_SIZE, 00035 .local_mtu = BOOTLOADER_LOCAL_MTU, 00036 .nb_archives = BOOTLOADER_NB_ARCHIVES, 00037 .signature = BOOTLOADER_SIGNATURE, 00038 .mfg_id = BOOTLOADER_MFG_ID, 00039 .dev_id = BOOTLOADER_DEV_ID, 00040 .hw_id = BOOTLOADER_HW_ID, 00041 .fw_major = BOOTLOADER_FW_MAJOR, 00042 .fw_minor = BOOTLOADER_FW_MINOR, 00043 .fw_patch = BOOTLOADER_FW_PATCH, 00044 .fw_hash = BOOTLOADER_FW_HASH, 00045 .target_fw_major = BOOTLOADER_TARGET_FW_MAJOR, 00046 .target_fw_minor = BOOTLOADER_TARGET_FW_MINOR, 00047 }; 00048 00049 void my_cup_callback(uint8_t terminal, int8_t err, uint8_t id) 00050 { 00051 (void)id; 00052 00053 if (terminal) 00054 { 00055 if (err) 00056 { 00057 PRINT("Done err %d\n", err); 00058 FLUSH(); 00059 while(1); 00060 } 00061 else 00062 { 00063 //PRINT("\nDone.\n"); 00064 } 00065 modem_cup_ready.release(); 00066 } 00067 else if (err) 00068 { 00069 PRINT("Got err %d\n", err); 00070 FLUSH(); 00071 while(1); 00072 } 00073 } 00074 00075 void cup_start_update(uint32_t offset, bool bootloader) 00076 { 00077 cup_cfg_t cfg = { 00078 .cmd = 0x10AD, 00079 .arch_nb = 20, 00080 }; 00081 00082 cup_param_t* cup; 00083 00084 uint32_t fof = 0; 00085 uint8_t percent = 0; 00086 uint8_t percent_old = 255; 00087 Timer tim; 00088 int32_t rem; 00089 float now = 0; 00090 00091 float speed_before = 0; 00092 float speed = 0; 00093 int speed_data = 0; 00094 00095 float time_before = 0; 00096 float time_left = 0; 00097 00098 float print_before = 0; 00099 00100 uint8_t id = modem_get_id(my_cup_callback); 00101 00102 if (bootloader) 00103 { 00104 PRINT("Uploading Bootloader\r\n"); 00105 cup = (cup_param_t*)&cup_bootloader; 00106 } 00107 else 00108 { 00109 PRINT("Uploading New version\r\n"); 00110 cup = (cup_param_t*)&cup_modem; 00111 } 00112 00113 rem = cup->data_size; 00114 00115 // Start CUP 00116 modem_write_file_root(cup->cfg_fid, (uint8_t*)&cfg, 0, 4, root_key, id); 00117 modem_cup_ready.acquire(); 00118 00119 // Upload file 00120 PRINT("Uploading %d bytes to CUP file. (offset %d)\r\n", cup->data_size, offset); 00121 00122 tim.start(); 00123 00124 while (rem > 0) 00125 { 00126 int32_t chunk = (rem > cup->local_mtu)? cup->local_mtu : rem; 00127 modem_write_file(cup->code_fid, &(cup->data[fof]), fof + offset, chunk, id); 00128 modem_cup_ready.acquire(); 00129 rem -= chunk; 00130 fof += chunk; 00131 00132 now = tim.read(); 00133 speed_data += chunk; 00134 00135 // Update speed 00136 if (now - speed_before > 1.0 || speed_before == 0) 00137 { 00138 speed = (speed_data/(now - speed_before))/1024.0; 00139 speed_before = now; 00140 speed_data = 0; 00141 } 00142 00143 // Update time left 00144 if (now - time_before > 0.2 || time_before == 0 || rem == 0) 00145 { 00146 time_before = now; 00147 time_left = (rem / speed) / 1024.0; 00148 } 00149 00150 // Print 00151 if (now - print_before > 0.1 || print_before == 0 || rem == 0) 00152 { 00153 percent = (100*fof)/cup->data_size; 00154 print_before = now; 00155 PRINT("\rUPLOADING CUP FILE %d/%d (%3d%%) %.2f kB/s %.0fs ", fof, cup->data_size, percent, speed, time_left); 00156 } 00157 } 00158 00159 PRINT("\n"); 00160 00161 float time_s = tim.read(); 00162 PRINT("CUP: %d bytes written in %.2f sec (%.2f kB/s)\r\n", cup->data_size, time_s, (cup->data_size/time_s)/1024.0); 00163 00164 // Force PFLASH-cache flushing 00165 modem_flush_file_root(cup->code_fid, root_key, id); 00166 modem_cup_ready.acquire(); 00167 00168 // Send Upgrade command 00169 cfg.cmd = 0xC0D5; 00170 cfg.arch_nb = cup->nb_archives; 00171 cfg.src_offset = offset; 00172 cfg.signature = cup->signature; 00173 00174 modem_write_file_root(cup->cfg_fid, (uint8_t*)&cfg, 0, 12, root_key, id); 00175 modem_cup_ready.acquire(); 00176 00177 PRINT("Waiting self reboot...\r\n"); 00178 } 00179
Generated on Fri Jul 15 2022 19:44:14 by
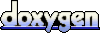