Complete sensor demo.
Dependencies: modem_ref_helper CRC X_NUCLEO_IKS01A1 DebouncedInterrupt
simul.cpp
00001 #include "mbed.h" 00002 #include "simul.h" 00003 #include "WizziDebug.h" 00004 00005 00006 unsigned int m_z=12434,m_w=33254; 00007 00008 unsigned int rnd() { 00009 m_z = 36969 * (m_z & 65535) + (m_z >>16); 00010 m_w = 18000 * (m_w & 65535) + (m_w >>16); 00011 return ((m_z <<16) + m_w); 00012 } 00013 00014 uint32_t g_simul_divider = 500; 00015 00016 void simul_update_param(uint32_t value) 00017 { 00018 g_simul_divider = value; 00019 } 00020 00021 bool simul_sensor_value(int32_t* buf, uint32_t nb_values, int32_t min, int32_t max) 00022 { 00023 for (uint8_t i = 0; i < nb_values; i++) 00024 { 00025 int32_t new_value; 00026 00027 uint32_t r = rnd()%g_simul_divider; 00028 00029 if (r) 00030 { 00031 if (r%2) 00032 { 00033 new_value = buf[i] + 1; 00034 } 00035 else 00036 { 00037 new_value = buf[i] - 1; 00038 } 00039 00040 if (new_value > max) 00041 { 00042 new_value = max; 00043 } 00044 00045 if (new_value < min) 00046 { 00047 new_value = min; 00048 } 00049 } 00050 else 00051 { 00052 new_value = (rnd()%(max - min)) + min; 00053 } 00054 00055 buf[i] = new_value; 00056 } 00057 00058 return false; 00059 } 00060
Generated on Tue Jul 12 2022 15:22:26 by
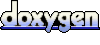