Complete sensor demo.
Dependencies: modem_ref_helper CRC X_NUCLEO_IKS01A1 DebouncedInterrupt
modem_callbacks.cpp
00001 #include "modem_d7a.h" 00002 #include "files.h" 00003 00004 #define SERIAL_MAX_PACKET_SIZE (255) 00005 00006 // ============================================================}}} 00007 00008 // Callbacks to MODEM's ALP requests 00009 // ============================================================{{{ 00010 void my_read(u8 action, u8 fid, u32 offset, u32 length, int id) 00011 { 00012 u8 data[SERIAL_MAX_PACKET_SIZE]; 00013 00014 ASSERT((ALP_ACTION_RSP_TAG_SIZE + ALP_ACTION_RSP_F_DATA_SIZE(offset, length)) <= SERIAL_MAX_PACKET_SIZE, 00015 "Read response too big for serial protocol (%d/%dmax)", length, ALP_ACTION_RSP_TAG_SIZE + ALP_ACTION_RSP_F_DATA_SIZE(offset,SERIAL_MAX_PACKET_SIZE)); 00016 00017 if (ram_fs_read(fid, data, offset, length)) 00018 { 00019 modem_ref_respond(action, ALP_ERR_FILE_NOT_FOUND, id); 00020 } 00021 else 00022 { 00023 modem_ref_respond_read(fid, data, offset, length, id); 00024 } 00025 } 00026 00027 00028 void my_write(u8 action, u8 fid, void *data, u32 offset, u32 length, int id) 00029 { 00030 alp_errors_t err; 00031 00032 if (ram_fs_write(fid, (uint8_t*)data, offset, length)) 00033 { 00034 err = ALP_ERR_FILE_NOT_FOUND; 00035 } 00036 else 00037 { 00038 err = ALP_ERR_NONE; 00039 00040 touch_t* touch = (touch_t*)MALLOC(sizeof(touch_t)); 00041 00042 touch->fid = fid; 00043 touch->offset = offset; 00044 touch->length = length; 00045 00046 g_file_modified.put(touch); 00047 } 00048 00049 modem_ref_respond(action, err, id); 00050 } 00051 00052 void my_read_fprop(u8 action, u8 fid, int id) 00053 { 00054 u8* hdr = (u8*)ram_fs_get_header(fid); 00055 00056 if (hdr != NULL) 00057 { 00058 modem_ref_respond_fprop(fid, (alp_file_header_t*)hdr, id); 00059 } 00060 else 00061 { 00062 modem_ref_respond(action, ALP_ERR_FILE_NOT_FOUND, id); 00063 } 00064 } 00065 00066 void my_flush(u8 action, u8 fid, int id) 00067 { 00068 // No flush in this file system 00069 modem_ref_respond(action, ALP_ERR_NONE, id); 00070 } 00071 00072 void my_delete(u8 action, u8 fid, int id) 00073 { 00074 modem_ref_respond(action, (ram_fs_delete(fid))? ALP_ERR_FILE_NOT_FOUND : ALP_ERR_NONE, id); 00075 } 00076 00077 void my_udata(alp_payload_t* alp) 00078 { 00079 alp_payload_print(alp); 00080 } 00081 00082 void my_lqual(u8 ifid, int per) 00083 { 00084 PRINT("Interface File [%3d] LQUAL : %d%% PER\r\n", ifid, per); 00085 } 00086 00087 void my_ldown(u8 ifid) 00088 { 00089 PRINT("Interface File [%3d] LDOWN\r\n", ifid); 00090 } 00091 00092 void my_reset(void) 00093 { 00094 PRINT("Restarting application...\r\n"); 00095 FLUSH(); 00096 NVIC_SystemReset(); 00097 } 00098 00099 void my_boot(u8 cause, u16 number) 00100 { 00101 PRINT("Modem BOOT[%c] #%d\r\n", cause, number); 00102 00103 // Modem re-booted, restart APP 00104 my_reset(); 00105 } 00106 00107 void my_busy(u8 busy) 00108 { 00109 if (busy) 00110 { 00111 PRINT("Modem Busy\r\n"); 00112 00113 /* Stop report, do not use modem */ 00114 /* Wait for modem reboot or modem not busy */ 00115 } 00116 else 00117 { 00118 PRINT("Modem not Busy\r\n"); 00119 00120 /* Resume reports */ 00121 } 00122 }
Generated on Tue Jul 12 2022 15:22:26 by
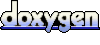