LoRaWAN demo.
Dependencies: modem_ref_helper DebouncedInterrupt
d7a_callbacks.cpp
00001 #include "modem_d7a.h" 00002 #include "ram_fs.h" 00003 00004 00005 // ============================================================}}} 00006 00007 // Callbacks to MODEM's ALP requests 00008 // ============================================================{{{ 00009 void my_read(u8 action, uint8_t fid, uint32_t offset, uint32_t length, int id) 00010 { 00011 uint8_t data[256]; 00012 #if 0 00013 //if (!ram_fs_read(fid, (uint8_t*)data, offset, length)) 00014 { 00015 modem_ref_respond_read(fid, data, offset, length, id); 00016 } 00017 else 00018 #endif 00019 { 00020 modem_ref_respond(action, ALP_ERR_FILE_NOT_FOUND, id); 00021 } 00022 } 00023 00024 void my_write(u8 action, uint8_t fid, void *data, uint32_t offset, uint32_t length, int id) 00025 { 00026 if (!ram_fs_write(fid, (uint8_t*)data, offset, length)) 00027 { 00028 extern Queue<uint8_t, 8> g_file_modified; 00029 00030 modem_ref_respond(action, ALP_ERR_NONE, id); 00031 g_file_modified.put((uint8_t*)(uint32_t)fid); 00032 } 00033 else 00034 { 00035 modem_ref_respond(action, ALP_ERR_FILE_NOT_FOUND, id); 00036 } 00037 } 00038 00039 void my_read_fprop(u8 action, uint8_t fid, int id) 00040 { 00041 uint8_t* hdr = (uint8_t*)ram_fs_get_header(fid); 00042 00043 if (hdr != NULL) 00044 { 00045 modem_ref_respond_fprop(fid, (alp_file_header_t*)hdr, id); 00046 } 00047 else 00048 { 00049 modem_ref_respond(action, ALP_ERR_FILE_NOT_FOUND, id); 00050 } 00051 } 00052 00053 void my_flush(u8 action, uint8_t fid, int id) 00054 { 00055 // No flush in this file system 00056 modem_ref_respond(action, ALP_ERR_NONE, id); 00057 } 00058 00059 void my_delete(u8 action, uint8_t fid, int id) 00060 { 00061 modem_ref_respond(action, (ram_fs_delete(fid))? ALP_ERR_FILE_NOT_FOUND : ALP_ERR_NONE, id); 00062 } 00063 00064 void my_udata(alp_payload_t* alp) 00065 { 00066 alp_payload_print(alp); 00067 } 00068 00069 void my_lqual(uint8_t ifid, int per) 00070 { 00071 PRINT("Interface File [%3d] LQUAL : %d%% PER\r\n", ifid, per); 00072 } 00073 00074 void my_ldown(uint8_t ifid) 00075 { 00076 PRINT("Interface File [%3d] LDOWN\r\n", ifid); 00077 } 00078 00079 void my_reset(void) 00080 { 00081 PRINT("Restarting application...\r\n"); 00082 FLUSH(); 00083 NVIC_SystemReset(); 00084 } 00085 00086 void my_boot(uint8_t cause, u16 number) 00087 { 00088 PRINT("Modem BOOT[%c] #%d\r\n", cause, number); 00089 00090 // Modem re-booted, restart APP 00091 my_reset(); 00092 } 00093 00094 void my_busy(uint8_t busy) 00095 { 00096 if (busy) 00097 { 00098 PRINT("Modem Busy\r\n"); 00099 00100 /* Stop report, do not use modem */ 00101 /* Wait for modem reboot or modem not busy */ 00102 } 00103 else 00104 { 00105 PRINT("Modem not Busy\r\n"); 00106 00107 /* Resume reports */ 00108 } 00109 } 00110 00111 void my_itf_busy(uint8_t ifid, uint32_t seconds) 00112 { 00113 extern Queue<uint32_t, 8> g_urc; 00114 00115 //PRINT("ITF[%d] Busy for %d seconds.\r\n", ifid, seconds); 00116 g_urc.put((uint32_t*)seconds); 00117 }
Generated on Wed Jul 13 2022 08:15:36 by
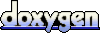