Broadcast read demo.
Dependencies: modem_ref_helper DebouncedInterrupt
main.cpp
00001 // @autor: jeremie@wizzilab.com 00002 // @date: 2017-05-02 00003 00004 #include "DebouncedInterrupt.h" 00005 #include "modem_d7a.h" 00006 #include "modem_callbacks.h" 00007 00008 static Semaphore button_user(0); 00009 00010 modem_ref_callbacks_t callbacks = { 00011 .read = my_read, 00012 .write = my_write, 00013 .read_fprop = my_read_fprop, 00014 .flush = my_flush, 00015 .remove = my_delete, 00016 .udata = my_udata, 00017 .reset = my_reset, 00018 .boot = my_boot, 00019 }; 00020 00021 alp_d7a_itf_t my_itf = { 00022 .type = ALP_ITF_TYPE_D7A, 00023 .cfg.to = D7A_CTF(0), 00024 .cfg.te = D7A_CTF(0), 00025 .cfg.qos.bf.resp = D7A_RESP_ALL, 00026 .cfg.qos.bf.retry = ALP_RPOL_ONESHOT, 00027 .cfg.addressee.ctrl.bf.nls = D7A_NLS_AES_CCM_64, 00028 .cfg.addressee.ctrl.bf.idf = D7A_ID_NBID, 00029 .cfg.addressee.xcl.bf = {.s = 2, .m = 0x1},// XXX D7A_XCL_GW, 00030 .cfg.addressee.id[0] = D7A_CTF_ENCODE(8), 00031 }; 00032 00033 // Interrupt Service Routine on button press. 00034 void button_push_isr( void ) 00035 { 00036 button_user.release(); 00037 } 00038 00039 void button_user_thread() 00040 { 00041 FPRINT("(id:0x%08x)\r\n", osThreadGetId()); 00042 00043 osEvent evt; 00044 fw_version_t fw_ver; 00045 uint8_t nb = 0; 00046 alp_payload_t* alp; 00047 alp_payload_t* alp_rsp; 00048 d7a_sp_res_t istat; 00049 int err; 00050 00051 uint8_t scan_xcl[] = { 0x01, 0x21 }; 00052 00053 modem_d7a_enable_itf(); 00054 00055 while (true) 00056 { 00057 // Wait for button press 00058 PRINT("Press button to scan...\r\n"); 00059 button_user.acquire(); 00060 00061 for (uint8_t i = 0; i < sizeof(scan_xcl); i++) 00062 { 00063 nb = 0; 00064 my_itf.cfg.addressee.xcl.byte = scan_xcl[i]; 00065 00066 PRINT("Scanning XCL 0x%02X...\n", my_itf.cfg.addressee.xcl.byte); 00067 00068 alp = NULL; 00069 alp = alp_payload_f_rd_data(alp, D7A_FID_FIRMWARE_VERSION, 12, sizeof(fw_version_t), false); 00070 00071 err = modem_remote_raw_alp((void*)&my_itf, alp, &alp_rsp); 00072 00073 if (err < ALP_ERR_NONE) 00074 { 00075 PRINT("Timeout.\n"); 00076 } 00077 else 00078 { 00079 err = alp_payload_get_err(alp_rsp); 00080 modem_print_error(my_itf.type, err); 00081 } 00082 00083 do { 00084 nb++; 00085 00086 if (alp_payload_extract_data(&alp_rsp, ALP_OPCODE_RSP_ISTATUS, &istat)) 00087 { 00088 PRINT("%2d: XCL:%02X ", nb, istat.addressee.xcl.byte); 00089 PRINT_DATA("UID:", "%02X", istat.addressee.id, 8, " "); 00090 PRINT("snr:%2d rxlev:%2d lb:%3d ", istat.snr, istat.rxlev, istat.lb); 00091 } 00092 else 00093 { 00094 break; 00095 } 00096 00097 if (alp_payload_extract_data(&alp_rsp, ALP_OPCODE_RSP_F_DATA, &fw_ver)) 00098 { 00099 PRINT("v%d.%d.%d\n", fw_ver.major, fw_ver.minor, fw_ver.patch); 00100 } 00101 else 00102 { 00103 break; 00104 } 00105 00106 FLUSH(); 00107 } while (1); 00108 00109 alp_payload_free(alp_rsp); 00110 } 00111 00112 PRINT("Done.\n"); 00113 } 00114 } 00115 00116 /*** Main function ------------------------------------------------------------- ***/ 00117 int main() 00118 { 00119 // Start & initialize 00120 #ifdef DEBUG_LED 00121 DBG_OPEN(DEBUG_LED); 00122 #else 00123 DBG_OPEN(NC); 00124 #endif 00125 PRINT("\n" 00126 "-----------------------------------------\n" 00127 "--------- Demo ActiveRFIDReader ---------\n" 00128 "-----------------------------------------\n"); 00129 00130 FPRINT("(id:0x%08x)\r\n", osThreadGetId()); 00131 00132 modem_open(&callbacks); 00133 00134 #ifdef DEBUG_BUTTON 00135 DebouncedInterrupt user_interrupt(DEBUG_BUTTON); 00136 user_interrupt.attach(button_push_isr, IRQ_FALL, 500, true); 00137 00138 Thread but_th(osPriorityNormal, 2048, NULL); 00139 osStatus status = but_th.start(button_user_thread); 00140 ASSERT(status == osOK, "Failed to start but thread (err: %d)\r\n", status); 00141 #else 00142 #error You need a button to use this APP as is 00143 #endif 00144 00145 #ifdef DEBUG_LED 00146 DigitalOut my_led(DEBUG_LED); 00147 #endif 00148 00149 // Set main task to lowest priority 00150 osThreadSetPriority(osThreadGetId(), osPriorityLow); 00151 while(true) 00152 { 00153 ThisThread::sleep_for(500); 00154 #ifdef DEBUG_LED 00155 my_led = !my_led; 00156 #endif 00157 } 00158 }
Generated on Wed Jul 13 2022 08:15:48 by
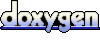