
WavSpiller having the songs on an SDCard
Dependencies: WavPlayer mbed C12832 SDFileSystem
Dependents: WavPlayerUSB WavPlayerUSB
main.cpp
00001 #include "mbed.h" 00002 #include "string.h" 00003 #include "stdio.h" 00004 #include "math.h" 00005 #include "C12832.h" 00006 #include "WavPlayer.h" 00007 #include "SDFileSystem.h" 00008 #include <string> 00009 using namespace std; 00010 00011 //Communication\interfaces 00012 Serial pc(USBTX,USBRX); //USB serial 00013 C12832 lcd(p5, p7, p6, p8, p11); //LCD 00014 SDFileSystem sd(p11, p12, p13, p15, "sd"); //SDCard 00015 DigitalIn sdDetect(p14); //CardDetect 00016 00017 //in- and outputs 00018 DigitalIn PauseBtn(p21); 00019 DigitalIn StopBtn(p22); 00020 00021 //joystick 00022 BusIn joy(p19,p17,p18,p20); 00023 DigitalIn joyBtn(p14); 00024 00025 //DAC output 00026 WavPlayer waver; //set up DAC to use wave player library 00027 00028 00029 //timer 00030 Timer dur; 00031 00032 //func 00033 void read_file_names(char *dir); //SDCard read file names 00034 void menu(); 00035 void PlaySong(int m); 00036 void PauseSong(); 00037 void StopSong(); 00038 00039 //variables 00040 int m = 1; //index of choosen songs 00041 int i = 0; //index of files from folder 00042 int Paused = 0; //Is the song paused? 00043 int Stopped = 0; //Is the song stopped? 00044 char* songs[]; 00045 00046 int main() 00047 { 00048 while(sdDetect == 0) { 00049 lcd.locate(0,0); 00050 lcd.printf("Insert SD Card!"); 00051 wait(0.5); 00052 } 00053 menu(); 00054 } 00055 00056 void menu() 00057 { 00058 lcd.cls(); 00059 sd.disk_initialize(); 00060 read_file_names("/sd/Music"); 00061 while(1) { 00062 lcd.locate(0,0); 00063 lcd.printf("Please select a song"); 00064 if(joy == 1) { 00065 m++; 00066 if(m == 5) { 00067 m = 0; 00068 } 00069 } else if(joy == 2) { 00070 m--; 00071 if(m == -1) { 00072 m = 4; 00073 } 00074 } 00075 lcd.locate(0,15); 00076 lcd.printf("%s", (songs[m])); 00077 if(joyBtn == 1) { 00078 PlaySong(m); 00079 } 00080 wait(0.1); 00081 } 00082 } 00083 00084 void read_file_names(char *dir) // function that reads in file names from sd cards 00085 { 00086 DIR *dp; 00087 struct dirent *dirp; 00088 dp = opendir(dir); 00089 //read all directory and file names in current directory into filename vector 00090 while((dirp = readdir(dp)) != NULL) { 00091 songs[i] = dirp->d_name; 00092 i++; 00093 } 00094 } 00095 00096 void PlaySong(int m) 00097 { 00098 string songname = songs[m]; 00099 string a = "/sd/Music/"; 00100 string fname = a + songname; //retrieves the file name 00101 FILE *wave_file; 00102 dur.start(); 00103 lcd.cls(); 00104 lcd.locate(0,0); 00105 lcd.printf("Now playing"); 00106 wave_file = fopen(fname.c_str(),"r"); //opens the music file 00107 waver.open(wave_file); 00108 waver.play(); //plays the music file 00109 lcd.locate(0,10); 00110 lcd.printf("%s", (songs[m])); 00111 while(Stopped == 0) { 00112 if(StopBtn == 1) { 00113 StopSong(); 00114 } 00115 if(PauseBtn == 1) { 00116 PauseSong(); 00117 } 00118 lcd.locate(0,20); 00119 lcd.printf("%2.f s", dur.read()); 00120 } 00121 fclose(wave_file); 00122 menu(); 00123 } 00124 00125 void PauseSong() 00126 { 00127 while(1) { 00128 if(Paused == 0) { 00129 string songname = songs[m]; 00130 unsigned index = songname.find(".wav"); 00131 songname = songname.substr(0,index); 00132 lcd.printf(songname.c_str()); 00133 dur.stop(); 00134 Paused = 1; 00135 } else if(StopBtn == 1) { 00136 StopSong(); 00137 } else { 00138 Paused = 0; 00139 dur.start(); 00140 } 00141 } 00142 } 00143 00144 void StopSong() 00145 { 00146 lcd.cls(); 00147 dur.reset(); 00148 Stopped = 1; 00149 } 00150 00151 00152 00153
Generated on Thu Aug 25 2022 06:29:07 by
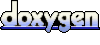