
WizFi310 DNS TCP Helloworld library 2.0 version
Dependencies: WizFi310Interface_Legacy mbed
main.cpp
00001 /* NetworkSocketAPI Example Program 00002 * Copyright (c) 2015 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "mbed.h" 00018 #include "WizFi310Interface.h" 00019 00020 00021 #if defined(TARGET_NUCLEO_F411RE) 00022 Serial pc(USBTX, USBRX); 00023 WizFi310Internet wifi(PA_11, PA_12, D6, D7, D3, NC, 115200); 00024 #endif 00025 00026 #if defined(TARGET_WIZwiki_W7500) 00027 Serial pc(USBTX, USBRX); 00028 WizFi310Interface wizfi310(D1, D0, D7, D6, D8, NC, 115200); 00029 #endif 00030 00031 #define SECURE WizFi310::SEC_AUTO 00032 #define SSID "wizms1" 00033 #define PASS "maker0701" 00034 00035 int main() 00036 { 00037 pc.baud(115200); 00038 printf("WizFi310 NetworkSocketAPI TCP Client Example\r\n"); 00039 wizfi310.init(); 00040 wizfi310.connect(SECURE, SSID, PASS); 00041 00042 const char *ip = wizfi310.getIPAddress(); 00043 const char *mac = wizfi310.getMACAddress(); 00044 printf("IP address is: %s\r\n", ip ? ip : "No IP"); 00045 printf("MAC address is: %s\r\n", mac ? mac : "No MAC"); 00046 00047 Endpoint addr; 00048 addr.set_address("mbed.org", 80); 00049 printf("mbed.org resolved to: %s\r\n", addr.get_address()); 00050 00051 TCPSocketConnection socket; 00052 socket.connect("4.ifcfg.me", 23); 00053 00054 char buffer[64]; 00055 int count = socket.receive(buffer, sizeof(buffer)); 00056 printf("public IP address is: %.15s\r\n", &buffer[15]); 00057 00058 socket.close(); 00059 wizfi310.disconnect(); 00060 00061 printf("Done\r\n"); 00062 00063 }
Generated on Sat Jul 16 2022 10:13:07 by
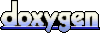