This library can be used in mbed driver or mbed OS2. So If you want to use WizFi310 on mbed OS5, You have to use another WizFi310 library(wizfi310-driver). That is git repository for wizfi310-driver. - https://github.com/ARMmbed/wizfi310-driver
Dependents: KT_IoTMakers_WizFi310_Example WizFi310_STATION_HelloWorld WizFi310_DNS_TCP_HelloWorld WizFi310_Ubidots ... more
WizFi310_hal.cpp
00001 /* 00002 * Copyright (C) 2013 gsfan, MIT License 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00005 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00006 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00007 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00008 * furnished to do so, subject to the following conditions: 00009 * 00010 * The above copyright notice and this permission notice shall be included in all copies or 00011 * substantial portions of the Software. 00012 * 00013 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00014 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00015 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00016 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00017 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00018 */ 00019 /* Copyright (C) 2014 Wiznet, MIT License 00020 * port to the Wiznet Module WizFi310 00021 */ 00022 00023 #include "WizFi310.h" 00024 00025 void WizFi310::setReset(bool flg) 00026 { 00027 if( flg ) 00028 { 00029 // low 00030 _reset.output(); 00031 _reset = 0; 00032 } 00033 else 00034 { 00035 // high z 00036 _reset.input(); 00037 _reset.mode(PullNone); 00038 } 00039 } 00040 00041 void WizFi310::isrUart() 00042 { 00043 char c; 00044 00045 c = getUart(); 00046 00047 recvData(c); 00048 } 00049 00050 int WizFi310::getUart() 00051 { 00052 return _wizfi.getc(); 00053 } 00054 00055 void WizFi310::putUart (char c) 00056 { 00057 _wizfi.putc(c); 00058 } 00059 00060 void WizFi310::setRts (bool flg) 00061 { 00062 if (flg) 00063 { 00064 if(_flow == 2) 00065 { 00066 if(_rts) 00067 { 00068 _rts->write(0); // low 00069 } 00070 } 00071 } 00072 else 00073 { 00074 if(_flow == 2) 00075 { 00076 if(_rts) 00077 { 00078 _rts->write(1); // high 00079 } 00080 } 00081 } 00082 } 00083 00084 int WizFi310::lockUart (int ms) 00085 { 00086 Timer t; 00087 00088 if(_state.mode != MODE_COMMAND) 00089 { 00090 t.start(); 00091 while(_state.mode != MODE_COMMAND) 00092 { 00093 if(t.read_ms() >= ms) 00094 { 00095 WIZ_WARN("lock timeout (%d)\r\n", _state.mode); 00096 return -1; 00097 } 00098 } 00099 } 00100 00101 #ifdef CFG_ENABLE_RTOS 00102 if (_mutexUart.lock(ms) != osOK) return -1; 00103 #endif 00104 00105 if(_flow == 2) 00106 { 00107 if(_cts && _cts->read()) 00108 { 00109 // CTS check 00110 t.start(); 00111 while (_cts->read()) 00112 { 00113 if(t.read_ms() >= ms) 00114 { 00115 WIZ_DBG("cts timeout\r\n"); 00116 return -1; 00117 } 00118 } 00119 } 00120 } 00121 00122 setRts(false); // blcok 00123 return 0; 00124 } 00125 00126 void WizFi310::unlockUart() 00127 { 00128 setRts(true); // release 00129 #ifdef CFG_ENABLE_RTOS 00130 _mutexUart.unlock(); 00131 #endif 00132 } 00133 00134 void WizFi310::initUart (PinName cts, PinName rts, PinName alarm, int baud) 00135 { 00136 _baud = baud; 00137 if (_baud) _wizfi.baud(_baud); 00138 00139 _wizfi.attach(this, &WizFi310::isrUart, Serial::RxIrq); 00140 00141 _cts = NULL; 00142 _rts = NULL; 00143 _flow = 0; 00144 00145 if(cts != NC) 00146 { 00147 _cts = new DigitalIn(cts); 00148 } 00149 if(rts != NC) 00150 { 00151 _rts = new DigitalOut(rts); 00152 _flow = 2; 00153 } 00154 }
Generated on Wed Jul 13 2022 18:48:26 by
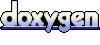