
LED can be controlled by remote TCP client. This code was tested with WIZwiki-W7500 platform board.
Dependencies: WIZnetInterface mbed
Fork of TCP_LED_Control-WIZwiki-W7500 by
main.cpp
00001 #include "mbed.h" 00002 #include "EthernetInterface.h" 00003 00004 #define MAC "\x00\x08\xDC\x55\x51\x52" 00005 #define IP "192.168.0.20" 00006 #define SUBNET "255.255.255.0" 00007 #define GATEWAY "192.168.0.1" 00008 00009 #define ECHO_SERVER_PORT 5000 00010 00011 //#define USE_DHCP 00012 00013 #define FLAG_LED_BLINK 3 00014 #define FLAG_LED_ON 2 00015 #define FLAG_LED_OFF 1 00016 #define FLAG_INVALID_CMD 0 00017 00018 #define LED_ON 0 00019 #define LED_OFF 1 00020 00021 void led_switch(void); 00022 00023 Ticker time_up; 00024 00025 /* LED Pin Configuration */ 00026 DigitalOut myled(LED1, LED_OFF); 00027 00028 /* UART Pin Configuration */ 00029 Serial pc(USBTX, USBRX); 00030 00031 // LED control if received message matches pre-defined commands 00032 char cmd_ledon[] = {'L', 'E', 'D', '_', 'O', 'N', '\0'}; 00033 char cmd_ledoff[] = {'L', 'E', 'D', '_', 'O', 'F', 'F', '\0'}; 00034 char cmd_ledblink[] = {'L', 'E', 'D', '_', 'B', 'L', 'I', 'N', 'K', '\0'}; 00035 00036 int flag; // LED status flag 00037 00038 void led_switch(void) 00039 { 00040 if(flag == FLAG_LED_BLINK) { 00041 myled=!myled; 00042 } 00043 } 00044 00045 int main (void) 00046 { 00047 /* Serial baudrate configuration */ 00048 pc.baud(115200); 00049 00050 // mbed Ticker 00051 time_up.attach(&led_switch, 0.2); 00052 00053 pc.printf("Wait a second...\r\n\r\n"); 00054 00055 // Ethernet 00056 EthernetInterface eth; 00057 #ifdef USE_DHCP 00058 eth.init(MAC); // Use DHCP 00059 #else 00060 eth.init((uint8_t*)MAC, IP, SUBNET, GATEWAY); // Use Static IP address 00061 #endif 00062 eth.connect(); 00063 00064 pc.printf("Server IP Address is %s : %d\r\n", eth.getIPAddress(), ECHO_SERVER_PORT); 00065 00066 pc.printf("Command #1: %s\r\n", cmd_ledon); 00067 pc.printf("Command #2: %s\r\n", cmd_ledoff); 00068 pc.printf("Command #3: %s\r\n", cmd_ledblink); 00069 pc.printf("\r\n"); 00070 00071 TCPSocketServer server; 00072 server.bind(ECHO_SERVER_PORT); 00073 server.listen(); 00074 00075 while (true) 00076 { 00077 pc.printf("Wait for new connection...\r\n"); 00078 TCPSocketConnection client; 00079 server.accept(client); 00080 client.set_blocking(false, 15000); // Timeout after (1.5)s 00081 00082 pc.printf("Connection from: %s\r\n", client.get_address()); 00083 printf("\r\n"); 00084 00085 char buffer[256]; 00086 while (true) { 00087 int n = client.receive(buffer, sizeof(buffer)); 00088 if (n <= 0) break; 00089 00090 // Print received message to terminal 00091 buffer[n] = '\0'; 00092 pc.printf("Received message from Client :'%s'\r\n",buffer); 00093 00094 // Compares the received string to predefined commands 00095 if(strncmp(buffer, cmd_ledon, sizeof(buffer)) == 0) { 00096 flag = FLAG_LED_ON; 00097 } else if(strncmp(buffer, cmd_ledoff, sizeof(buffer)) == 0) { 00098 flag = FLAG_LED_OFF; 00099 } else if(strncmp(buffer, cmd_ledblink, sizeof(buffer)) == 0) { 00100 flag = FLAG_LED_BLINK; 00101 } else { 00102 flag = FLAG_INVALID_CMD; 00103 } 00104 00105 // LED On/Off or Invalid command 00106 switch(flag) 00107 { 00108 case FLAG_LED_ON: 00109 myled = LED_ON; // LED ON in WIZwiki-W7500 00110 pc.printf("WIZwiki: LED is turned on!\r\n"); 00111 break; 00112 00113 case FLAG_LED_OFF: 00114 myled = LED_OFF; // LED OFF in WIZwiki-W7500 00115 pc.printf("WIZwiki: LED is turned off!\r\n"); 00116 break; 00117 00118 case FLAG_LED_BLINK: 00119 pc.printf("WIZwiki: LED starts blinking!\r\n"); 00120 break; 00121 00122 case FLAG_INVALID_CMD: 00123 default: 00124 pc.printf("WIZwiki: Invalid command\r\n"); 00125 break; 00126 } 00127 00128 // Reverse the message 00129 char temp; 00130 for(int f = 0, l = n-1; f<l; f++,l--){ 00131 temp = buffer[f]; 00132 buffer[f] = buffer[l]; 00133 buffer[l] = temp; 00134 } 00135 00136 // Print reversed message to terminal 00137 pc.printf("Sending reversed message to Client: '%s'\r\n", buffer); 00138 pc.printf("\r\n"); 00139 00140 // Echo received message back to client 00141 client.send_all(buffer, n); 00142 if (n <= 0) break; 00143 } 00144 00145 client.close(); 00146 } 00147 }
Generated on Thu Jul 14 2022 01:56:10 by
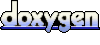