Time Stamp using fingerprint with WIZwiki-W7500.
Dependencies: GT511C3 NTPClient SDFileSystem WIZnetInterface mbed-src
Fork of GT511C3_HelloWorld_WIZwiki-W7500 by
main.cpp
00001 #include "mbed.h" 00002 #include "GT511C3.hpp" 00003 #include "SDFileSystem.h" 00004 #include "EthernetInterface.h" 00005 #include "NTPClient.h" 00006 00007 #define MAX_ID_NUM 20 00008 00009 #ifdef TARGET_WIZWIKI_W7500 00010 GT511C3 finger(PA_13,PA_14); 00011 DigitalIn del_ID(D7); 00012 DigitalIn enroll_ID(D8); 00013 InterruptIn in(D9); 00014 SDFileSystem sd(PB_3, PB_2, PB_1, PB_0, "sd"); // the pinout on the mbed 00015 uint8_t mac_addr[6] = {0x00, 0x08, 0xDC, 0x11, 0x22, 0xab}; 00016 #endif 00017 00018 Serial pc(USBTX,USBRX); 00019 EthernetInterface eth; 00020 NTPClient ntpClient; 00021 00022 FILE *fp; 00023 00024 00025 int progress(int status,char *msg) 00026 { 00027 pc.printf("%s",msg); 00028 return 0; 00029 } 00030 00031 void close_file(void) 00032 { 00033 fclose(fp); 00034 } 00035 00036 int main() 00037 { 00038 int i; 00039 int sts = 0; 00040 int ID = 0; 00041 int cnt = 0; 00042 int EnrollID; 00043 00044 char domainName[3][80] = {"kr.pool.ntp.org", "time.bora.net", "time.nuri.net"};//SET TO DOMAIN NAME OF SERVER GETTING TIME FROM 00045 char buffer[80]; //BUFFER TO HOLD FORMATTED TIME DATA 00046 time_t sysTime; 00047 00048 in.rise(&close_file); 00049 00050 pc.baud(115200); 00051 00052 printf("File open...\r\n"); 00053 00054 if((fp = fopen("/sd/time.txt", "w")) == NULL) 00055 { 00056 printf("Cannot open file!!\r\n"); 00057 return 0; 00058 } 00059 00060 printf("GT511C3 open....\r\n"); 00061 00062 while(1) 00063 { 00064 sts = finger.Open(); 00065 if(sts == -1) 00066 { 00067 printf("GT511C3 Open failed!!\r\n"); 00068 cnt++; 00069 while(cnt > 5); 00070 } 00071 else 00072 { 00073 break; 00074 } 00075 00076 wait(0.2); 00077 } 00078 00079 if(del_ID == 1) 00080 { 00081 finger.DeleteID_All(); 00082 printf("All ID are deleted!!\r\n"); 00083 } 00084 00085 cnt = 0; 00086 if(enroll_ID == 1) 00087 { 00088 while(1) 00089 { 00090 if(finger.CheckEnrolled(cnt) == -1) 00091 { 00092 EnrollID = cnt; 00093 printf("ID(%d) is empty\r\n", EnrollID); 00094 break; 00095 } 00096 cnt++; 00097 if(cnt > MAX_ID_NUM) 00098 { 00099 printf("\r\nERROR : ID number is fulled!!Delete ID first!!\r\n\r\n"); 00100 while(1); 00101 } 00102 } 00103 finger.Enroll(EnrollID,progress); 00104 } 00105 00106 eth.init(mac_addr); //Use DHCP 00107 printf("Check Ethernet Link\r\n"); 00108 while(1) //Wait link up 00109 { 00110 if(eth.link() == true) 00111 break; 00112 } 00113 printf("Link up\r\n"); 00114 printf("Getting IP address by DHCP...\r\n"); 00115 eth.connect(); 00116 printf("Server IP Address is %s\r\n", eth.getIPAddress()); 00117 00118 printf("Getting time information by using NTP...\r\n"); 00119 00120 cnt = 0; 00121 while(1) 00122 { 00123 if(ntpClient.setTime(domainName[cnt],123,0x00005000) != NTP_OK) 00124 { 00125 printf("Cannot get time information by NTP\r\n"); 00126 cnt++; 00127 } 00128 else 00129 break; 00130 00131 if(cnt > 3) 00132 { 00133 printf("All NTP servers are not resposed!!\r\n"); 00134 return 1; 00135 } 00136 } 00137 00138 printf("Completed Get and Set Time\r\n\r\n"); 00139 eth.disconnect(); 00140 00141 //Start to capture fingerprint 00142 finger.CmosLed(1); 00143 00144 while(1) 00145 { 00146 printf("Press finger for Identify\r\n"); 00147 finger.WaitPress(1); 00148 if(finger.Capture(1) != 0) 00149 continue; 00150 00151 ID = finger.Identify(); 00152 00153 if(ID == -1) 00154 printf("\r\nERROR : There is no ID!!Enroll first!!\r\n\r\n"); 00155 else 00156 { 00157 printf("ID = %d\r\n",ID); 00158 sysTime = time(NULL)+(3600*9); //TIME with offset for eastern time KR 00159 //FORMAT TIME FOR DISPLAY AND STORE FORMATTED RESULT IN BUFFER 00160 strftime(buffer,80,"%Y/%m/%d %p %I:%M:%S \r\n",localtime(&sysTime)); 00161 fprintf(fp, "ID : %d\r\nTime : %s\r\n", ID, buffer); 00162 printf("Date and Time\r\n%s\r\n", buffer); 00163 } 00164 00165 printf("Remove finger\r\n"); 00166 finger.WaitPress(0); 00167 } 00168 } 00169
Generated on Tue Jul 12 2022 17:56:29 by
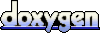