Update FTPClient
Dependents: Digital_Photo_Frame_with_FTP_SD_WIZwiki-W7500 FTP_Streaming_Music_Player_WIZwiki-W7500 GIF2015 MP3Decoding_VS1002_WIZwiki-W7500
Fork of FTPClient by
FTPClient.h
00001 #ifndef FTP_CLIENT_H 00002 #define FTP_CLIENT_H 00003 #include "mbed.h" 00004 #include "SDFileSystem.h" 00005 #define MAX_SS 512 00006 /** FTPClient class. 00007 * Used file transfer with FTPServer like ALFTP(http://software.altools.co.kr/ko-kr/closed.html) 00008 * This test was completed in ALFTP 00009 */ 00010 class FTPClient{ 00011 public: 00012 /** Create FTPClient instance */ 00013 FTPClient(PinName mosi, PinName miso, PinName sclk, PinName ssel, const char* root); 00014 FTPClient(const char* root); 00015 ~FTPClient(); 00016 00017 /** Connect to FTPServer 00018 * 00019 * @param FTPServer IP, FTPServer PORT, FTPServer login ID, FTPServer login PASS 00020 * @returns 00021 * 1 on success, 00022 * 0 on open error 00023 */ 00024 bool open(char* ip, int port, char* id, char* pass); 00025 00026 /** Get file from FTPServer 00027 * 00028 * @param filename 00029 * @returns 00030 * 1 on success, 00031 * 0 on getfile error 00032 */ 00033 bool getfile(char* filename); 00034 00035 /** Put file to FTPServer 00036 * 00037 * @param FTPServer file name 00038 * @returns 00039 * 1 on success, 00040 * 0 on putfile error 00041 */ 00042 bool putfile(char* filename); 00043 00044 /** View FTPServer directory 00045 * 00046 * @param 00047 * @returns 00048 * 1 on success, 00049 * 0 on dir error 00050 */ 00051 bool dir(char* liststr); 00052 00053 /** View FTPServer directory 00054 * 00055 * @param 00056 * @returns 00057 * 1 on success, 00058 * 0 on ls error 00059 */ 00060 bool ls(char* liststr); 00061 00062 /** Delete FTPServer file 00063 * 00064 * @param FTPServer file name 00065 * @returns 00066 * 1 on success, 00067 * 0 on delete error 00068 */ 00069 bool fdelete(char* filename); 00070 00071 /** Make FTPServer directory 00072 * 00073 * @param FTPServer directory name 00074 * @returns 00075 * 1 on success, 00076 * 0 on mkdir error 00077 */ 00078 bool mkdir(char* dirname); 00079 00080 /** Change current FTPServer directory 00081 * 00082 * @param FTPServer directory name 00083 * @returns 00084 * 1 on success, 00085 * 0 on mkdir error 00086 */ 00087 bool cd(char* dirname); 00088 00089 /** Disconnect from FTPServer 00090 * 00091 * @param 00092 * @returns 00093 * 1 on success, 00094 * 0 on Disconnect error 00095 */ 00096 bool quit(); 00097 00098 private: 00099 TCPSocketConnection FTPClientControlSock; 00100 TCPSocketConnection FTPClientDataSock; 00101 00102 bool blogin; 00103 00104 char ftpServer_data_ip_addr_str[20]; 00105 int remote_port; 00106 00107 char ftpbuf[MAX_SS]; 00108 00109 00110 SDFileSystem* _SDFileSystem; 00111 00112 int pportc(char * arg); 00113 00114 char root[20]; 00115 }; 00116 #endif
Generated on Thu Jul 14 2022 12:00:17 by
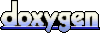