This is a test program for Camera Module(C328, LJ-DSC02)
Dependencies: CameraC328 SDFileSystem mbed-src
Fork of Camera_TestProgram_2015 by
main.cpp
00001 /** 00002 * Test program. 00003 * 00004 * Copyright (C) 2010 Shinichiro Nakamura (CuBeatSystems) 00005 * http://shinta.main.jp/ 00006 */ 00007 00008 /* 00009 * Include files. 00010 */ 00011 00012 #include "mbed.h" 00013 #include "CameraC328.h" 00014 #include "SDFileSystem.h" 00015 00016 /* 00017 * Definitions. 00018 */ 00019 #define USE_JPEG_HIGH_RESOLUTION 1 00020 #define USE_SD_CARD 1 00021 00022 /* 00023 * Variables. 00024 */ 00025 static const int CAPTURE_FRAMES = 1; 00026 static FILE *fp_jpeg; 00027 00028 /* 00029 * Modules. 00030 */ 00031 #if USE_SD_CARD 00032 SDFileSystem sd(PB_3, PB_2, PB_1, PB_0, "fs"); // the pinout on the mbed Cool Components workshop board 00033 #else 00034 LocalFileSystem fs("fs"); 00035 #endif 00036 CameraC328 camera(PA_13, PA_14, CameraC328::Baud115200); 00037 00038 /** 00039 * A callback function for jpeg images. 00040 * You can block this function until saving the image datas. 00041 * 00042 * @param buf A pointer to the image buffer. 00043 * @param siz A size of the image buffer. 00044 */ 00045 void jpeg_callback(char *buf, size_t siz) { 00046 for (int i = 0; i < (int)siz; i++) { 00047 fprintf(fp_jpeg, "%c", buf[i]); 00048 } 00049 } 00050 00051 /** 00052 * Synchronizing. 00053 */ 00054 void sync(void) { 00055 CameraC328::ErrorNumber err = CameraC328::NoError; 00056 00057 err = camera.sync(); 00058 if (CameraC328::NoError == err) { 00059 printf("[ OK ] : CameraC328::sync\n"); 00060 } else { 00061 printf("[FAIL] : CameraC328::sync (Error=%02X)\n", (int)err); 00062 } 00063 } 00064 00065 /** 00066 * A test function for jpeg snapshot picture. 00067 */ 00068 void test_jpeg_snapshot_picture(void) { 00069 CameraC328::ErrorNumber err = CameraC328::NoError; 00070 00071 #if USE_JPEG_HIGH_RESOLUTION 00072 err = camera.init(CameraC328::Jpeg, CameraC328::RawResolution80x60, CameraC328::JpegResolution640x480); 00073 #else 00074 err = camera.init(CameraC328::Jpeg, CameraC328::RawResolution80x60, CameraC328::JpegResolution320x240); 00075 #endif 00076 if (CameraC328::NoError == err) { 00077 printf("[ OK ] : CameraC328::init\n"); 00078 } else { 00079 printf("[FAIL] : CameraC328::init (Error=%02X)\n", (int)err); 00080 } 00081 00082 for (int i = 0; i < CAPTURE_FRAMES; i++) { 00083 char fname[64]; 00084 snprintf(fname, sizeof(fname), "/fs/jpss%04d.jpg", i); 00085 fp_jpeg = fopen(fname, "w"); 00086 00087 err = camera.getJpegSnapshotPicture(jpeg_callback); 00088 if (CameraC328::NoError == err) { 00089 printf("[ OK ] : CameraC328::getJpegSnapshotPicture\n"); 00090 } else { 00091 printf("[FAIL] : CameraC328::getJpegSnapshotPicture (Error=%02X)\n", (int)err); 00092 } 00093 00094 fclose(fp_jpeg); 00095 } 00096 } 00097 00098 /** 00099 * A entry point. 00100 */ 00101 int main() { 00102 printf("\n"); 00103 printf("==========\n"); 00104 printf("CameraC328\n"); 00105 printf("==========\n"); 00106 00107 sync(); 00108 00109 test_jpeg_snapshot_picture(); 00110 }
Generated on Sat Jul 16 2022 00:42:54 by
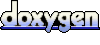