
Mail Queue Task
Dependencies: ELEC350-Practicals-FZ429
Fork of Task633-mbedos54 by
main.cpp
00001 #include "mbed.h" 00002 #include "sample_hardware.hpp" 00003 #include "string.h" 00004 #include <stdio.h> 00005 #include <ctype.h> 00006 00007 #define SWITCH1_RELEASE 1 00008 00009 void thread1(); 00010 void thread2(); 00011 void switchISR(); 00012 00013 00014 //Threads 00015 Thread samplingThread(osPriorityRealtime); 00016 Thread t1; 00017 00018 //Class type 00019 class message_t { 00020 public: 00021 float adcValue; 00022 int sw1State; 00023 int sw2State; 00024 00025 //Constructor 00026 message_t(float f, int s1, int s2) { 00027 adcValue = f; 00028 sw1State = s1; 00029 sw2State = s2; 00030 } 00031 }; 00032 00033 //Memory Pool - with capacity for 16 message_t types 00034 //MemoryPool<message_t, 16> mpool; 00035 00036 //Message queue - matched to the memory pool 00037 //Queue<message_t, 16> queue; 00038 00039 //Mail queue 00040 Mail<message_t, 16> mail_box; 00041 00042 00043 // Call this on precise intervals 00044 void adcISR() { 00045 //This is going to be brief 00046 samplingThread.signal_set(1); 00047 } 00048 void doSample() { 00049 00050 while (true) { 00051 //Waiting for ISR in WAITING state 00052 Thread::signal_wait(1); 00053 00054 //Read sample - make a copy 00055 float sample = adcIn; 00056 //Grab switch state 00057 uint32_t switch1State = SW1; 00058 uint32_t switch2State = SW2; 00059 00060 //Allocate a block from the memory pool 00061 message_t *message = mail_box.alloc(); 00062 if (message == NULL) { 00063 //Out of memory 00064 printf("Out of memory\n\r"); 00065 redLED = 1; 00066 return; 00067 } 00068 00069 //Fill in the data 00070 message->adcValue = sample; 00071 message->sw1State = switch1State; 00072 message->sw2State = switch2State; 00073 00074 //Write to queue 00075 osStatus stat = mail_box.put(message); //Note we are sending the "pointer" 00076 00077 //Check if succesful 00078 if (stat == osErrorResource) { 00079 redLED = 1; 00080 printf("queue->put() Error code: %4Xh, Resource not available\r\n", stat); 00081 mail_box.free(message); 00082 return; 00083 } 00084 00085 } //end whie 00086 } 00087 00088 //Normal priority thread (consumer) 00089 void thread1() 00090 { 00091 static int counter = 0; 00092 00093 while (true) { 00094 //Block on the queue 00095 osEvent evt = mail_box.get(); 00096 00097 //Check status 00098 if (evt.status == osEventMail) { 00099 message_t *pMessage = (message_t*)evt.value.p; //This is the pointer (address) 00100 //Make a copy 00101 message_t msg(pMessage->adcValue, pMessage->sw1State, pMessage->sw2State); 00102 //We are done with this, so give back the memory to the pool 00103 mail_box.free(pMessage); 00104 00105 //Echo to the terminal 00106 printf("ADC Value: %.2f\t", msg.adcValue); 00107 printf("SW1: %u\t", msg.sw1State); 00108 printf("SW2: %u\n\r", msg.sw2State); 00109 00110 //Toggle green 00111 if ( (msg.sw1State == 1) && (msg.sw2State == 1)) { 00112 counter++; 00113 if (counter == 10) { 00114 greenLED = !greenLED; 00115 counter = 0; 00116 } 00117 } else { 00118 counter = 0; 00119 } 00120 00121 } else { 00122 printf("ERROR: %x\n\r", evt.status); 00123 } 00124 00125 } //end while 00126 } 00127 00128 00129 // Main thread 00130 int main() { 00131 //Power on self-test 00132 post(); 00133 00134 //Start message 00135 printf("Welcome\n"); 00136 00137 //Hook up timer interrupt 00138 Ticker timer; 00139 timer.attach(&adcISR, 0.1); 00140 00141 //Threads 00142 samplingThread.start(doSample); //High Priority 00143 t1.start(thread1); 00144 00145 printf("Main Thread\n"); 00146 while (true) { 00147 Thread::wait(5000); 00148 puts("Main Thread Alive"); 00149 } 00150 } 00151 00152
Generated on Thu Jul 14 2022 12:17:40 by
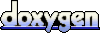