
Updated for the FZ429ZI
Fork of Task421 by
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 00003 #define kRED (1 << 2) //4 00004 #define kYELLOW (1 << 1) //2 00005 #define kGREEN (1 << 0) //1 00006 #define kALL (kRED | kYELLOW | kGREEN) 00007 00008 //Global objects 00009 BusOut binaryOutput(D5, D6, D7); 00010 DigitalIn SW1(D3); 00011 DigitalIn SW2(D4); 00012 00013 AnalogIn POT_ADC_In(A0); 00014 AnalogIn LDD_ADC_In(A1); 00015 float fPOT, fLDR = 0.0; 00016 00017 //Main function 00018 int main() { 00019 00020 while(1) { 00021 00022 //Read ADC 00023 fPOT = POT_ADC_In; 00024 fLDR = LDD_ADC_In; 00025 00026 //Write to terminal 00027 printf("POT = %6.4f\tLDR = %6.4f\n", fPOT, fLDR); 00028 00029 if (fLDR > fPOT) { 00030 binaryOutput = 0; //Binary 000 00031 } else { 00032 binaryOutput = kALL; //Binary 111 00033 } 00034 00035 //Wait 00036 wait(0.1); 00037 00038 } //end while(1) 00039 } //end main
Generated on Tue Aug 2 2022 17:55:37 by
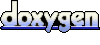