Creating a project for TT_Mxx
Embed:
(wiki syntax)
Show/hide line numbers
GraphicsDisplay.h
00001 /* mbed GraphicsDisplay Display Library Base Class 00002 * Copyright (c) 2007-2009 sford 00003 * Released under the MIT License: http://mbed.org/license/mit 00004 * 00005 * A library for providing a common base class for Graphics displays 00006 * To port a new display, derive from this class and implement 00007 * the constructor (setup the display), pixel (put a pixel 00008 * at a location), width and height functions. Everything else 00009 * (locate, printf, putc, cls, window, putp, fill, blit, blitbit) 00010 * will come for free. You can also provide a specialised implementation 00011 * of window and putp to speed up the results 00012 */ 00013 00014 #ifndef MBED_GRAPHICSDISPLAY_H 00015 #define MBED_GRAPHICSDISPLAY_H 00016 00017 #include "TextDisplay.h" 00018 00019 00020 #define RED 0xf800 00021 #define GREEN 0x07e0 00022 #define BLUE 0x001f 00023 #define BLACK 0x0000 00024 #define YELLOW 0xffe0 00025 #define WHITE 0xffff 00026 00027 #define FONT_CHAR_WIDTH 8 00028 #define FONT_CHAR_HEIGHT 16 00029 00030 class GraphicsDisplay : public TextDisplay { 00031 00032 public: 00033 00034 GraphicsDisplay(const char* name); 00035 00036 virtual void pixel(int x, int y, int colour) = 0; 00037 virtual int width() = 0; 00038 virtual int height() = 0; 00039 00040 virtual void window(unsigned int x,unsigned int y,unsigned int w,unsigned int h); 00041 virtual void putp(int colour); 00042 00043 virtual void cls(); 00044 virtual void fill(int x, int y, int w, int h, int colour); 00045 virtual void blit(int x, int y, int w, int h, const int *colour); 00046 virtual void blitbit(int x, int y, int w, int h, const char* colour); 00047 00048 virtual void character_(int x, int y, char value,int colour = WHITE); 00049 00050 virtual void character(int column, int row, int value); 00051 virtual int columns(); 00052 virtual int rows(); 00053 00054 virtual void sendData(uint16_t data) = 0; 00055 virtual void setAddress(uint16_t x0, uint16_t y0, uint16_t x1, uint16_t y1) = 0; 00056 00057 virtual void lcd_display_char(uint16_t hwXpos, //specify x position. 00058 uint16_t hwYpos, //specify y position. 00059 uint8_t chChr, //a char is display. 00060 uint8_t chSize, //specify the size of the char 00061 uint16_t hwColor); 00062 protected: 00063 00064 // pixel location 00065 short _x; 00066 short _y; 00067 00068 // window location 00069 short _x1; 00070 short _x2; 00071 short _y1; 00072 short _y2; 00073 00074 }; 00075 00076 #endif 00077
Generated on Tue Jul 12 2022 21:02:36 by
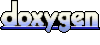