
Creating a project for TT_Mxx
Embed:
(wiki syntax)
Show/hide line numbers
HTU21D.h
00001 #ifndef HTU21D_H 00002 #define HTU21D_H 00003 00004 /** 00005 * Includes 00006 */ 00007 #include "mbed.h" 00008 00009 /** 00010 * Defines 00011 */ 00012 // Acquired from Datasheet. 00013 00014 #define HTU21D_I2C_ADDRESS 0x40 00015 #define TRIGGER_TEMP_MEASURE 0xE3 00016 #define TRIGGER_HUMD_MEASURE 0xE5 00017 00018 00019 //Commands. 00020 #define HTU21D_EEPROM_WRITE 0x80 00021 #define HTU21D_EEPROM_READ 0x81 00022 00023 00024 /** 00025 * Honeywell HTU21D digital humidity and temperature sensor. 00026 */ 00027 class HTU21D { 00028 00029 public: 00030 00031 HTU21D(); 00032 /** 00033 * Constructor. 00034 * 00035 * @param sda mbed pin to use for SDA line of I2C interface. 00036 * @param scl mbed pin to use for SCL line of I2C interface. 00037 */ 00038 HTU21D(PinName sda, PinName scl); 00039 00040 00041 /** 00042 * @brief Samples the temperature, input void, outputs an int in celcius. 00043 * @return [description] 00044 */ 00045 int sample_ctemp(void); 00046 00047 /** 00048 * @brief Samples the temperature, input void, outputs an int in fahrenheit. 00049 * @return [description] 00050 */ 00051 int sample_ftemp(void); 00052 00053 /** 00054 * @brief Samples the temperature, input void, outputs an int in kelvin. 00055 * @return [description] 00056 */ 00057 int sample_ktemp(void); 00058 00059 /** 00060 * @brief Samples the humidity, input void, outputs and int. 00061 * @return [description] 00062 */ 00063 int sample_humid(void); 00064 00065 00066 00067 private: 00068 00069 I2C* i2c_; 00070 00071 /** 00072 * Write to EEPROM or RAM on the device. 00073 * 00074 * @param EepromOrRam 0x80 -> Writing to EEPROM 00075 * @param address Address to write to. 00076 * @param data Data to write. 00077 */ 00078 void write(int EepromOrRam, int address, int data); 00079 00080 /** 00081 * Read EEPROM or RAM on the device. 00082 * 00083 * @param EepromOrRam 0x81 -> Reading from EEPROM 00084 * @param address Address to read from. 00085 * @return The contents of the memory address. 00086 */ 00087 int read(int EepromOrRam, int address); 00088 00089 }; 00090 00091 00092 #endif
Generated on Tue Jul 12 2022 22:50:09 by
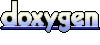