
Create a project for TT_Mxx.
Embed:
(wiki syntax)
Show/hide line numbers
MotionSensor.h
00001 /* MotionSensor Base Class 00002 * Copyright (c) 2014-2015 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef MOTIONSENSOR_H 00018 #define MOTIONSENSOR_H 00019 00020 #include "stdint.h" 00021 00022 /** motion_data_counts_t struct 00023 */ 00024 typedef struct { 00025 int16_t x ; /*!< x-axis counts */ 00026 int16_t y ; /*!< y-axis counts */ 00027 int16_t z ; /*!< z-axis counts */ 00028 } motion_data_counts_t; 00029 00030 /** motion_data_units_t struct 00031 */ 00032 typedef struct { 00033 float x ; /*!< x-axis counts */ 00034 float y ; /*!< y-axis counts */ 00035 float z ; /*!< z-axis counts */ 00036 } motion_data_units_t; 00037 00038 typedef motion_data_units_t MotionSensorDataUnits; 00039 typedef motion_data_counts_t MotionSensorDataCounts; 00040 /** Motion Sensor Base Class 00041 Useful for accessing data in a common way 00042 */ 00043 class MotionSensor 00044 { 00045 //public: 00046 00047 // /** Enable the sensor for operation 00048 // */ 00049 // virtual void enable(void) const = 0; 00050 00051 // /** disable the sensors operation 00052 // */ 00053 // virtual void disable(void) const = 0; 00054 00055 // /** Set the sensor sample rate 00056 // @param frequency The desires sample frequency 00057 // @return The amount of error in Hz between desired and actual frequency 00058 // */ 00059 // virtual uint32_t sampleRate(uint32_t frequency) const = 0; 00060 00061 // /** Tells of new data is ready 00062 // @return The amount of data samples ready to be read from a device 00063 // */ 00064 // virtual uint32_t dataReady(void) const = 0; 00065 00066 // /** Get the x data in counts 00067 // @param x A referene to the variable to put the data in, 0 denotes not used 00068 // @return The x data in counts 00069 // */ 00070 // virtual int16_t getX(int16_t &x) const = 0; 00071 00072 // /** Get the y data in counts 00073 // @param y A referene to the variable to put the data in, 0 denotes not used 00074 // @return The y data in counts 00075 // */ 00076 // virtual int16_t getY(int16_t &y) const = 0; 00077 00078 // * Get the z data in counts 00079 // @param z A referene to the variable to put the data in, 0 denotes not used 00080 // @return The z data in counts 00081 00082 // virtual int16_t getZ(int16_t &z) const = 0; 00083 00084 // /** Get the x data in units 00085 // @param x A referene to the variable to put the data in, 0 denotes not used 00086 // @return The x data in units 00087 // */ 00088 // virtual float getX(float &x) const = 0; 00089 00090 // /** Get the y data in units 00091 // @param y A referene to the variable to put the data in, 0 denotes not used 00092 // @return The y data in units 00093 // */ 00094 // virtual float getY(float &y) const = 0; 00095 00096 // /** Get the z data in units 00097 // @param z A referene to the variable to put the data in, 0 denotes not used 00098 // @return The z data in units 00099 // */ 00100 // virtual float getZ(float &z) const = 0; 00101 00102 // /** Get the x,y,z data in counts 00103 // @param xyz A referene to the variable to put the data in, 0 denotes not used 00104 // */ 00105 // virtual void getAxis(motion_data_counts_t &xyz) const = 0; 00106 00107 // /** Get the x,y,z data in units 00108 // @param xyz A referene to the variable to put the data in, 0 denotes not used 00109 // */ 00110 // virtual void getAxis(motion_data_units_t &xyz) const = 0; 00111 }; 00112 00113 #endif 00114
Generated on Wed Jul 13 2022 20:20:49 by
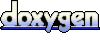