A simple CRC library used to verify configuration data
Fork of CRC by
crc.cpp
00001 /** crc.cpp 00002 * 00003 * @license 00004 * MIT License 00005 * 00006 * Copyright (c) 2016 Andrew D Lindsay/Thing Innovations https://thinginnovations.uk 00007 * 00008 * Permission is hereby granted, free of charge, to any person obtaining a copy 00009 * of this software and associated documentation files (the "Software"), to deal 00010 * in the Software without restriction, including without limitation the rights 00011 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00012 * copies of the Software, and to permit persons to whom the Software is 00013 * furnished to do so, subject to the following conditions: 00014 * 00015 * The above copyright notice and this permission notice shall be included in all 00016 * copies or substantial portions of the Software. 00017 * 00018 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00019 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00020 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00021 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00022 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00023 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE 00024 * SOFTWARE. 00025 * 00026 */ 00027 #define CRC32_POLYNOMIAL 0xEDB88320L 00028 00029 void CRC32Value(unsigned long &CRCval, unsigned char c) 00030 { 00031 ///////////////////////////////////////////////////////////////////////////////////// 00032 //CRC must be initialized as zero 00033 //c is a character from the sequence that is used to form the CRC 00034 //this code is a modification of the code from the Novatel OEM615 specification 00035 ///////////////////////////////////////////////////////////////////////////////////// 00036 unsigned long ulTemp1 = ( CRCval >> 8 ) & 0x00FFFFFFL; 00037 unsigned long ulCRC = ((int) CRCval ^ c ) & 0xff ; 00038 for (int j = 8 ; j > 0; j-- ) 00039 { 00040 if ( ulCRC & 1 ) 00041 { 00042 ulCRC = ( ulCRC >> 1 ) ^ CRC32_POLYNOMIAL; 00043 } 00044 else 00045 { 00046 ulCRC >>= 1; 00047 } 00048 } 00049 CRCval = ulTemp1 ^ ulCRC; 00050 } 00051 00052 /* -------------------------------------------------------------------------- 00053 Calculates the CRC-32 of a block of data all at once 00054 //the CRC is from the complete message (header plus data) 00055 //but excluding (of course) the CRC at the end 00056 -------------------------------------------------------------------------- */ 00057 unsigned long CalculateBlockCRC32( 00058 unsigned long ulCount, /* Number of bytes in the data block */ 00059 unsigned char *ucBuffer ) /* Data block */ 00060 { 00061 ////////////////////////////////////////////////////////////////////// 00062 //the below code tests the CRC32Value procedure used in a markov form 00063 ////////////////////////////////////////////////////////////////////// 00064 unsigned long CRC = 0; 00065 for (int i = 0; i<ulCount; i++) 00066 { 00067 CRC32Value( CRC, *ucBuffer++ ); 00068 } 00069 return CRC; 00070 } 00071 00072 /* 00073 unsigned long CalculateBlockCRC32( 00074 unsigned long ulCount, 00075 unsigned char *ucBuffer ) 00076 { 00077 //////////////////////////////////////////// 00078 //original code from the OEM615 manual 00079 //////////////////////////////////////////// 00080 unsigned long ulTemp1; 00081 unsigned long ulTemp2; 00082 unsigned long ulCRC = 0; 00083 while ( ulCount-- != 0 ) 00084 { 00085 ulTemp1 = ( ulCRC >> 8 ) & 0x00FFFFFFL; 00086 ulTemp2 = CRC32Value( ((int) ulCRC ^ *ucBuffer++ ) & 0xff ); 00087 ulCRC = ulTemp1 ^ ulTemp2; 00088 } 00089 return( ulCRC ); 00090 } 00091 */
Generated on Fri Jul 15 2022 06:11:46 by
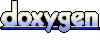