
embedded software for tortuga bike
Dependencies: BLE_API BikeControl DataLogging X_NUCLEO_IDB0XA1 _24LCXXX mbed
main.cpp
00001 #include "mbed.h" 00002 #include "BikeData.h" 00003 #include "BatteryState.h" 00004 #include "BikeControl.h" 00005 #include "ble/BLE.h" 00006 #include "ble/services/BikeService.h" 00007 #include "ble/services/BikeBatteryService.h" 00008 00009 #define trailerBatteryPin PB_0 00010 #define bikeBatteryPin PC_5 00011 #define auxiliaryBatteryPin PC_4 00012 00013 /*************************************************************************** 00014 VARIABLES 00015 ****************************************************************************/ 00016 00017 /* 00018 //LIGHT 00019 DigitalOut lightFront(PB_14); 00020 DigitalOut lightBack(PA_11); 00021 DigitalOut lightLeft(PC_6); 00022 DigitalOut lightRight(PC_8);*/ 00023 00024 00025 static BikeControl *bc; 00026 00027 00028 //3 battery states 00029 static BatteryState *trailerBattery; 00030 static BatteryState *bikeBattery; 00031 static BatteryState *auxiliaryBattery; 00032 00033 //BLE VARIABLES 00034 static char DEVICE_NAME[] = "NAME"; //Define default name of the BLE device 00035 static const uint16_t uuid16_list[] = {BikeService::BIKE_SERVICE_UUID, 00036 //GattService::UUID_DEVICE_INFORMATION_SERVICE, 00037 BatteryService::BATTERY_SERVICE_UUID, 00038 }; // List of the Service UUID's that are used. 00039 00040 //Pointers to diferent objects and BLE services. 00041 static BikeData *bd; 00042 static BikeService *bikeServicePtr; 00043 //static DeviceInformationService *DISptr; 00044 static BatteryService *batSerPtr; 00045 00046 //bool that is set to true each second. 00047 static bool update = false; 00048 static bool updateHalf = false; 00049 00050 /*************************************************************************** 00051 CONTROL FUNCTIONS 00052 ****************************************************************************/ 00053 /*void runTestLight() 00054 { 00055 printf("front\n"); 00056 lightFront =1; 00057 wait(1); 00058 lightFront = 0; 00059 printf("back\n"); 00060 lightBack = 1; 00061 wait(1); 00062 lightBack = 0; 00063 printf("left\n"); 00064 lightLeft = 1; 00065 wait(1); 00066 lightLeft = 0; 00067 printf("right\n"); 00068 lightRight = 1; 00069 wait(1); 00070 lightRight = 0; 00071 wait(1); 00072 }*/ 00073 00074 /*************************************************************************** 00075 BLE FUNCTIONS 00076 ****************************************************************************/ 00077 00078 void disconnectionCallback(const Gap::DisconnectionCallbackParams_t *params) 00079 { 00080 //restart advertising when disconnected 00081 BLE::Instance().gap().startAdvertising(); 00082 } 00083 00084 void periodicCallback(void) 00085 { 00086 //Set update high to send new data over BLE 00087 update = true; 00088 } 00089 00090 00091 //This function is called when the ble initialization process has failled 00092 void onBleInitError(BLE &ble, ble_error_t error) 00093 { 00094 /* Initialization error handling should go here */ 00095 printf("initialization error!\n"); 00096 } 00097 00098 00099 //Callback triggered when the ble initialization process has finished 00100 void bleInitComplete(BLE::InitializationCompleteCallbackContext *params) 00101 { 00102 BLE& ble = params->ble; 00103 ble_error_t error = params->error; 00104 00105 if (error != BLE_ERROR_NONE) { /* In case of error, forward the error handling to onBleInitError */ 00106 printf("BLE error\n"); 00107 onBleInitError(ble, error); 00108 return; 00109 } 00110 00111 /* Ensure that it is the default instance of BLE */ 00112 if(ble.getInstanceID() != BLE::DEFAULT_INSTANCE) { 00113 return; 00114 } 00115 00116 //set the disconnect function 00117 ble.gap().onDisconnection(disconnectionCallback); 00118 printf("BLE Disconnect callback set\n"); 00119 00120 /* Setup primary services */ 00121 //DISptr = new DeviceInformationService(ble, "The Opportunity Factory", "1.0", "PJTMN06", "1.0", "0.9", "0.9"); 00122 bikeServicePtr = new BikeService(ble,bd); 00123 batSerPtr = new BatteryService(ble, 00124 trailerBattery->getBatteryPercentage(), 00125 bikeBattery->getBatteryPercentage(), 00126 auxiliaryBattery->getBatteryPercentage()); 00127 00128 printf("Services set\n"); 00129 00130 /* setup advertising */ 00131 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::GENERIC_CYCLING);//set apperance 00132 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::BREDR_NOT_SUPPORTED | GapAdvertisingData::LE_GENERAL_DISCOVERABLE); //different BLE options set 00133 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LIST_16BIT_SERVICE_IDS, (uint8_t *)uuid16_list, sizeof(uuid16_list));//Adding the list of UUID's 00134 //ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LOCAL_NAME, (uint8_t *)DEVICE_NAME, sizeof(DEVICE_NAME));//passing the device name 00135 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LOCAL_NAME, (uint8_t *)DEVICE_NAME, bd->getBikeNameSize());//passing the device name 00136 ble.gap().setAdvertisingType(GapAdvertisingParams::ADV_CONNECTABLE_UNDIRECTED);//setting connection type 00137 ble.gap().setAdvertisingInterval(100); /* 1000ms. */ 00138 ble.gap().startAdvertising(); 00139 printf("advertising\n"); 00140 } 00141 00142 00143 int main() { 00144 00145 00146 printf("\n======================\n\n"); 00147 printf("SETUP \n"); 00148 00149 bd = new BikeData(PC_1); // creating BikeData object 00150 printf("BikeData object created\n"); 00151 00152 00153 trailerBattery = new BatteryState(trailerBatteryPin,BatteryState::Battery48V); 00154 bikeBattery = new BatteryState(bikeBatteryPin,BatteryState::Battery48V); 00155 auxiliaryBattery = new BatteryState(auxiliaryBatteryPin,BatteryState::Battery48V); 00156 00157 bc = new BikeControl(bd,trailerBattery, bikeBattery, auxiliaryBattery); 00158 00159 00160 printf("run TestLight \n"); 00161 //runTestLight(); 00162 bc->runTestLight(); 00163 00164 uint8_t size = bd->getBikeNameSize(); 00165 printf("name length: %i\n", size); 00166 if (size == 0){ 00167 printf("Setting Name First Time\n"); 00168 static char testName[] = "Tortuga"; 00169 bd -> setBikeName((char *)testName,sizeof(testName)); 00170 printf("SetFistTimeName\n"); 00171 //printf( "size: %i\n",bd -> getBikeNameSize()); 00172 //printf("first character: %c",bd->getBikeName()); 00173 } 00174 00175 bd->getBikeName(DEVICE_NAME); 00176 printf("got device name\n"); 00177 BLE &ble = BLE::Instance(); // creating BLE object 00178 printf("BLE object created\n"); 00179 BLE::Instance().init(bleInitComplete); // call init function 00180 00181 while (ble.hasInitialized() == false); // Wait until BLE is initialized 00182 printf("BLE object intitialized\n"); 00183 Ticker ticker; 00184 ticker.attach(periodicCallback, 1); //creating the ticker that wil update each second. 00185 printf("ticker 1 attached\n"); 00186 00187 printf("START \n"); 00188 00189 00190 bc->startControlLoop(); 00191 00192 while(true) 00193 { 00194 if (update){ 00195 printf("--------------\n"); 00196 float distance = (bd->getDataSet(BikeData::OVERALL))->getDistance(); 00197 printf("main distance: %f\n", distance); 00198 float speed = bd->getSpeed(); 00199 printf("main speed: %f\n", speed); 00200 00201 //printf("update bikeService\n"); 00202 bikeServicePtr->update(); //update the bikeserice 00203 //printf("update battery1: %i\n", trailerBattery->getBatteryPercentage()); 00204 //batSerPtr->updateBatteryLevel1((++batLevel)%=101); 00205 //printf("battery level: %i\n", batLevel); 00206 batSerPtr->updateBatteryLevel1(trailerBattery->getBatteryPercentage()); 00207 //printf("update battery2: %i\n", bikeBattery->getBatteryPercentage()); 00208 batSerPtr->updateBatteryLevel2(bikeBattery->getBatteryPercentage()); 00209 //printf("update battery3: %i\n", auxiliaryBattery->getBatteryPercentage()); 00210 batSerPtr->updateBatteryLevel3(auxiliaryBattery->getBatteryPercentage()); 00211 update = false; 00212 } 00213 ble.waitForEvent(); //wait for an event when idle. 00214 00215 bc->checkStatus(); 00216 } 00217 }
Generated on Sun Jul 17 2022 20:40:13 by
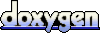