
TaDA
Fork of luce_rossa_piezo by
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "note.h" 00003 #include "Servo.h" 00004 00005 DigitalIn bottone(p14); 00006 DigitalOut ledR(p25); 00007 DigitalOut ledG(p24); 00008 DigitalOut ledB(p26); 00009 PwmOut piezo(p22); 00010 Servo myservo1(p23); 00011 Servo myservo2(p21); 00012 int c=0; 00013 00014 void suono(int frequenza, int beat); 00015 00016 int main() 00017 { 00018 while(1) { 00019 00020 c=0; 00021 if(bottone==1) { 00022 ledG=1; 00023 ledR=0; 00024 suono(NOTE_B4,1); 00025 wait(1); 00026 00027 do { 00028 wait(0.001); 00029 c++; 00030 if(c++>=10000) { 00031 ledG=0; 00032 ledR=1; 00033 suono(NOTE_C5,1); 00034 } 00035 } while(!bottone); 00036 //*************************MOVIMENTO SERVI************************ 00037 int a=0; 00038 int b=0; 00039 if(a<2) a++; 00040 else if(a>=2) { 00041 for(float i=0; i<30; i++) { 00042 myservo1=i; 00043 wait(0.1); 00044 a=0; 00045 b++; 00046 } 00047 } 00048 if(b==5){ 00049 for(float i=180; i<0; i++) 00050 myservo1=i; 00051 wait(0.1); 00052 b=0; 00053 } 00054 int f=0; 00055 00056 if(f==0){ 00057 for(float i=0; i<30; i++) 00058 myservo2=i; 00059 wait(0.1); 00060 f++; 00061 } 00062 else if(f==1) 00063 for(float i=0; i<60; i++) 00064 myservo2=i; 00065 wait(0.1); 00066 f++; 00067 if(f==3){ 00068 for(float i=60; i<0; i--) 00069 myservo2=i; 00070 wait(0.1); 00071 f=0; 00072 } 00073 00074 //**************************************************************** 00075 00076 ledR=0; 00077 ledB=1; 00078 wait(1); 00079 ledB=0; 00080 suono(NOTE_F1,1); 00081 } 00082 00083 wait_ms(1); 00084 00085 } 00086 } 00087 00088 void suono(int frequenza, int beat) 00089 { 00090 piezo.period(1.0 / frequenza); 00091 piezo.write(0.5); 00092 wait(1.0 / beat); 00093 piezo.write(0); 00094 wait(0.05); 00095 }
Generated on Sun Jul 31 2022 07:51:08 by
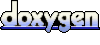