
Kviz izveden pomocu mikrokontrolera NUCLEO-F103RB, 16x2 LCD-a i 3 tipkala.
Dependencies: mbed Pitanja TextLCD
main.cpp
00001 #include "mbed.h" 00002 #include "pitanja.h" 00003 #include "TextLCD.h" 00004 00005 DigitalOut myled(LED1); 00006 TextLCD lcd(PA_0,PA_1,PA_4,PB_0,PC_1,PC_0); // Rs, E, D4-D7 00007 InterruptIn button(USER_BUTTON); 00008 DigitalIn buttonC(PA_9); 00009 DigitalIn buttonB(PC_7); 00010 DigitalIn buttonA(PB_6); 00011 Timer timer; 00012 int i = 1; 00013 int brojTocnih = 0; 00014 00015 void start(); 00016 void zapocniKviz(); 00017 void postaviPitanje(string pitanjeA,string pitanjeB, string odgA, string odgB, string odgC, string tocanOdg); 00018 00019 int main() 00020 { 00021 std::vector<Pitanje> pitanja = Pitanje::init(); 00022 myled = 0; 00023 string pitanjeA; 00024 string pitanjeB; 00025 string odgA; 00026 string odgB; 00027 string odgC; 00028 string tocanOdg; 00029 00030 zapocniKviz(); 00031 int j = 1; 00032 while(j) { 00033 Pitanje* iter = pitanja.begin(); 00034 int i = 1; 00035 for ( ; iter != pitanja.end(); iter++) { 00036 pitanjeA = (*iter).getPitanjeA(); 00037 pitanjeB = (*iter).getPitanjeB(); 00038 odgA = (*iter).getOdgA(); 00039 odgB = (*iter).getOdgB(); 00040 odgC = (*iter).getOdgC(); 00041 lcd.cls(); 00042 tocanOdg = (*iter).getTocanOdg(); 00043 lcd.printf("%d. pitanje:",i); 00044 wait(1.5); 00045 lcd.cls(); 00046 i++; 00047 00048 postaviPitanje(pitanjeA, pitanjeB, odgA, odgB, odgC, tocanOdg); 00049 00050 if(i == 10) { 00051 j = 0; 00052 } 00053 } 00054 } 00055 lcd.cls(); 00056 lcd.printf("Kviz zavrsen!"); 00057 lcd.locate(3,1); 00058 lcd.printf("Tocno %d/10", brojTocnih); 00059 wait(3); 00060 } 00061 00062 void start() 00063 { 00064 i = 0; 00065 } 00066 00067 void zapocniKviz() 00068 { 00069 lcd.locate(2,0); 00070 lcd.printf("Zapocni kviz"); 00071 lcd.locate(5,1); 00072 lcd.printf("Sretno!"); 00073 while(i) { 00074 button.rise(&start); 00075 } 00076 lcd.cls(); 00077 } 00078 00079 void postaviPitanje(string pitanjeA,string pitanjeB, string odgA, string odgB, string odgC, string tocanOdg) 00080 { 00081 lcd.cls(); 00082 lcd.locate(0,0); 00083 lcd.printf("%s",pitanjeA.c_str()); 00084 lcd.locate(0,1); 00085 lcd.printf("%s",pitanjeB.c_str()); 00086 wait(3); 00087 lcd.cls(); 00088 00089 lcd.printf("A)%s",odgA.c_str()); 00090 wait(2); 00091 lcd.cls(); 00092 00093 lcd.printf("B)%s",odgB.c_str()); 00094 wait(2); 00095 lcd.cls(); 00096 00097 lcd.printf("C)%s",odgC.c_str()); 00098 wait(2); 00099 lcd.cls(); 00100 00101 timer.reset(); 00102 timer.start(); 00103 lcd.printf("Vas odgovor je?"); 00104 while(timer.read_ms() < 3500) { 00105 if(buttonA || buttonB || buttonC) { 00106 if (buttonA &&(tocanOdg == odgA)) { 00107 lcd.cls(); 00108 lcd.locate(5,0); 00109 lcd.printf("Tocno"); 00110 wait(1); 00111 brojTocnih++; 00112 break; 00113 } 00114 if (buttonB &&(tocanOdg == odgB)) { 00115 lcd.cls(); 00116 lcd.locate(5,0); 00117 lcd.printf("Tocno"); 00118 wait(1); 00119 brojTocnih++; 00120 break; 00121 } 00122 if (buttonC &&(tocanOdg == odgC)) { 00123 lcd.cls(); 00124 lcd.locate(5,0); 00125 lcd.printf("Tocno"); 00126 wait(1); 00127 brojTocnih++; 00128 break; 00129 } else { 00130 lcd.cls(); 00131 lcd.locate(5,0); 00132 lcd.printf("Krivo"); 00133 wait(1); 00134 break; 00135 } 00136 } 00137 if (timer.read_ms() >2500) { 00138 lcd.cls(); 00139 lcd.printf("Isteklo vrijeme"); 00140 wait(1); 00141 break; 00142 } 00143 } 00144 }
Generated on Thu Jul 14 2022 14:38:47 by
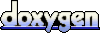