
Lab version
Dependencies: mbed
main.cpp
00001 #include "mbed.h" 00002 00003 00004 //Global objects 00005 DigitalOut myled(LED1); 00006 Serial pc(USBTX, USBRX); 00007 Ticker t; 00008 00009 //Integer state of the LED 00010 //static makes it visible only within main.cpp 00011 static int state = 0; 00012 00013 //Function prototype 00014 void doISR(); 00015 00016 int main() { 00017 00018 //Initialise 00019 pc.baud(115200); 00020 t.attach(&doISR, 2); 00021 myled = 0; 00022 00023 //Main loop 00024 while(1) { 00025 00026 //Go to sleep and wait for an ISR to wake 00027 sleep(); //At is says 00028 00029 //At this point, the ISR has run 00030 00031 //Update LED 00032 myled = state; 00033 00034 //Echo to terminal 00035 if (state == 0) { 00036 pc.printf("LED OFF\n"); 00037 } else { 00038 pc.printf("LED ON\n"); 00039 } 00040 } 00041 } 00042 00043 //Note - the ISR is short, and does NOT call functions 00044 //such as printf (it's actually unsafe to do so) 00045 //Always check a function is "reentrant" before calling it 00046 void doISR() { 00047 //Toggle 0 to 1, or 1 to 0 00048 state ^= 1; 00049 }
Generated on Fri Aug 26 2022 21:27:00 by
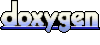