
Task 1.3.3 Solution
Dependencies: mbed
main.cpp
00001 //This is known as a “header file” 00002 //In short, this copies and pastes the text file 00003 //mbed.h into this code 00004 #include "mbed.h" 00005 00006 //Create a DigitalOut “object” called myled 00007 //Pass constant D7 as a “parameter” 00008 DigitalOut redLED(D7); 00009 DigitalOut yellowLED(D6); 00010 DigitalOut greenLED(D5); 00011 00012 //The main function - all executable C / C++ 00013 //applications have a main function. This is 00014 //out entry point in the software 00015 int main() { 00016 00017 redLED = 0; 00018 yellowLED = 0; 00019 greenLED = 0; 00020 00021 // ALL the code is contained in a 00022 // “while loop" 00023 00024 // THIS IS NOT AN IDEAL SOLUTION. HOWEVER IT IS SIMPLE 00025 00026 00027 while(1) 00028 { 00029 //The code between the { curly braces } 00030 //is the code that is repeated 00031 00032 //STATE 1 (R) 00033 redLED = 1; 00034 yellowLED = 0; 00035 greenLED = 0; 00036 wait(1.0); 00037 00038 //STATE 2 (RA) 00039 yellowLED = 1; 00040 wait(1.0); 00041 00042 //STATE 3 (G) 00043 redLED = 0; 00044 yellowLED = 0; 00045 greenLED = 1; 00046 wait(1.0); 00047 00048 //STATE 4 (A) 00049 yellowLED = 1; 00050 greenLED = 0; 00051 wait(0.25); 00052 00053 //STATE 5 00054 yellowLED = 0; 00055 wait(0.25); 00056 00057 //STATE 6 00058 yellowLED = 1; 00059 wait(0.25); 00060 00061 //STATE 7 00062 yellowLED = 0; 00063 wait(0.25); 00064 00065 00066 } 00067 }
Generated on Tue Jul 19 2022 21:28:16 by
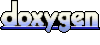