
Demo for showing Thunderboard Sense 2's sensor values on a 15-second interval
Dependencies: AMS_CCS811_gas_sensor BMP280 Si7021 Si7210 Si1133 ICM20648
Fork of TBSense2_Sensor_Demo by
main.cpp
00001 /***************************************************************************//** 00002 * @file main.cpp 00003 ******************************************************************************* 00004 * @section License 00005 * <b>(C) Copyright 2017 Silicon Labs, http://www.silabs.com</b> 00006 ******************************************************************************* 00007 * 00008 * SPDX-License-Identifier: Apache-2.0 00009 * 00010 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00011 * not use this file except in compliance with the License. 00012 * You may obtain a copy of the License at 00013 * 00014 * http://www.apache.org/licenses/LICENSE-2.0 00015 * 00016 * Unless required by applicable law or agreed to in writing, software 00017 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00018 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00019 * See the License for the specific language governing permissions and 00020 * limitations under the License. 00021 * 00022 ******************************************************************************/ 00023 00024 #include <string> 00025 #include "mbed.h" 00026 #include "BMP280/BMP280.h" 00027 #include "AMS_CCS811.h" 00028 #include "Si7021.h" 00029 #include "Si7210.h" 00030 #include "Si1133.h" 00031 #include "ICM20648.h" 00032 00033 /* Turn on power supply to ENV sensor suite */ 00034 DigitalOut env_en(PF9, 1); 00035 /* Turn on power to CCS811 sensor */ 00036 DigitalOut ccs_en(PF14, 1); 00037 /* Set CCS_WAKE pin to output */ 00038 DigitalOut ccs_wake(PF15, 1); 00039 /* Turn on power to hall effect sensor */ 00040 DigitalOut hall_en(PB10, 1); 00041 /* Turn on power to IMU */ 00042 DigitalOut imu_en(PF8, 1); 00043 00044 I2C ccs_i2c(PB6, PB7); 00045 I2C hall_i2c(PB8, PB9); 00046 I2C env_i2c(PC4, PC5); 00047 00048 int main() { 00049 uint32_t eco2, tvoc; 00050 float pressure, temperature2; 00051 int32_t temperature; 00052 uint32_t humidity; 00053 float light, uv; 00054 float acc_x, acc_y, acc_z, gyr_x, gyr_y, gyr_z, temperature3; 00055 00056 bool lightsensor_en = true; 00057 bool gassensor_en = true; 00058 bool hallsensor_en = true; 00059 bool rhtsensor_en = true; 00060 bool pressuresensor_en = true; 00061 bool imu_en = true; 00062 00063 ccs_en = 0; 00064 wait_ms(1000); 00065 ccs_en = 1; 00066 wait_ms(1000); 00067 00068 /* Initialize air quality sensor */ 00069 AMS_CCS811* gasSensor = new AMS_CCS811(&ccs_i2c, PF15); 00070 if(!gasSensor->init()) { 00071 printf("Failed CCS811 init\r\n"); 00072 gassensor_en = false; 00073 } else { 00074 if(!gasSensor->mode(AMS_CCS811::TEN_SECOND)) { 00075 printf("Failed to set CCS811 mode\r\n"); 00076 gassensor_en = false; 00077 } 00078 } 00079 00080 wait_ms(10); 00081 00082 /* Initialize barometer and RHT */ 00083 BMP280* pressureSensor = new BMP280(env_i2c); 00084 Si7021* rhtSensor = new Si7021(PC4, PC5); 00085 00086 printf("\r\n\r\nHello Thunderboard Sense 2...\r\n"); 00087 00088 /* Check if hall sensor is alive */ 00089 silabs::Si7210* hallSensor = new silabs::Si7210(&hall_i2c); 00090 uint8_t id; 00091 hallSensor->wakeup(); 00092 hallSensor->readRegister(SI72XX_HREVID, &id); 00093 printf("Hall ID: %d\r\n", id); 00094 00095 /* Initialize light sensor */ 00096 Si1133* lightSensor = new Si1133(PC4, PC5); 00097 00098 if(!lightSensor->open()) { 00099 printf("Something is wrong with Si1133, disabling...\n"); 00100 lightsensor_en = false; 00101 } 00102 00103 /* Initialize the IMU*/ 00104 ICM20648* imu = new ICM20648(PC0, PC1, PC2, PC3, PF12); 00105 00106 if(!imu->open()) { 00107 printf("Something is wrong with ICM20648, disabling...\n"); 00108 imu_en = false; 00109 } 00110 00111 do { 00112 printf("----------------------------------------------\r\n"); 00113 /* Measure temperature and humidity */ 00114 if(rhtsensor_en) { 00115 rhtSensor->measure(); 00116 rhtSensor->measure(); 00117 temperature = rhtSensor->get_temperature(); 00118 humidity = rhtSensor->get_humidity(); 00119 00120 printf("Si7021:\r\n"); 00121 printf("T: %ld.%03ld degC\r\n", temperature/1000, abs(temperature%1000)); 00122 printf("h: %ld.%03ld %%\r\n", humidity/1000, humidity%1000); 00123 } 00124 00125 /* Measure barometric pressure */ 00126 if(pressuresensor_en) { 00127 temperature2 = pressureSensor->getTemperature(); 00128 pressure = pressureSensor->getPressure(); 00129 00130 printf("BMP280:\r\n"); 00131 printf("P: %.2f bar\r\n", pressure); 00132 printf("T: %.2f degC\r\n", temperature2); 00133 } 00134 00135 /* Measure air quality */ 00136 if(gassensor_en) { 00137 gasSensor->has_new_data(); 00138 eco2 = gasSensor->co2_read(); 00139 tvoc = gasSensor->tvoc_read(); 00140 00141 printf("CCS811:\r\n"); 00142 printf("CO2: %ld ppm\r\n", eco2); 00143 printf("VoC: %ld ppb\r\n", tvoc); 00144 } 00145 00146 /* measure HALL */ 00147 if(hallsensor_en) { 00148 hallSensor->measureOnce(); 00149 00150 printf("Si7210:\r\n"); 00151 printf("T: %d.%02d degC\r\n", hallSensor->getTemperature()/1000, abs(hallSensor->getTemperature()%1000)); 00152 printf("F: %i.%03d mT\r\n", (int)(hallSensor->getFieldStrength()/1000), abs(hallSensor->getFieldStrength() % 1000)); 00153 } 00154 00155 /* measure light */ 00156 if(lightsensor_en) { 00157 lightSensor->get_light_and_uv(&light, &uv); 00158 00159 printf("BMP280:\r\n"); 00160 printf("L: %.2f lux\r\n", light); 00161 printf("U: %.2f\r\n", uv); 00162 } 00163 00164 /* measure imu */ 00165 if(imu_en) { 00166 imu->get_temperature(&temperature3); 00167 imu->get_accelerometer(&acc_x, &acc_y, &acc_z); 00168 imu->get_gyroscope(&gyr_x, &gyr_y, &gyr_z); 00169 00170 printf("ICM20648:\r\n"); 00171 printf("T: %.2f degC\r\n", temperature3); 00172 printf("Acc: %.2f %.2f %.2f\r\n", acc_x, acc_y, acc_z); 00173 printf("Gyro: %.2f %.2f %.2f\r\n", gyr_x, gyr_y, gyr_z); 00174 } 00175 00176 wait_ms(15000); 00177 } while(1); 00178 }
Generated on Sat Jul 16 2022 18:45:28 by
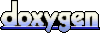