This is a driver for the segment LCD found on the Silicon Labs EF32 Giant, Leopard and Wonder Gecko platforms. NOTE: This driver will not work with other platforms, because it contains EFM32-specific code.
Dependents: EFM32 RDA5807M RDS Radio EMF32-Segment-Touch-Demo EMF32_ShowKey blinky_EFM32_Giant ... more
segmentlcd.h
00001 /**************************************************************************//** 00002 * @file 00003 * @brief EFM32 Segment LCD Display driver, header file 00004 * @version 3.20.9 00005 ****************************************************************************** 00006 * @section License 00007 * <b>(C) Copyright 2014 Silicon Labs, http://www.silabs.com</b> 00008 ******************************************************************************* 00009 * 00010 * This file is licensensed under the Silabs License Agreement. See the file 00011 * "Silabs_License_Agreement.txt" for details. Before using this software for 00012 * any purpose, you must agree to the terms of that agreement. 00013 * 00014 ******************************************************************************/ 00015 00016 00017 #ifndef __SEGMENTLCD_H 00018 #define __SEGMENTLCD_H 00019 00020 #include <stdint.h> 00021 #include <stdbool.h> 00022 00023 #if defined( TARGET_EFM32GG_STK3700 ) 00024 #include "segmentlcdconfig_stk_gg.h" 00025 #elif defined( TARGET_EFM32_G8XX_STK ) 00026 #include "segmentlcdconfig_stk_g.h" 00027 #elif defined( TARGET_EFM32LG_STK3600 ) 00028 #include "segmentlcdconfig_stk_lg.h" 00029 #elif defined( TARGET_EFM32TG_STK3300 ) 00030 #include "segmentlcdconfig_stk_tg.h" 00031 #elif defined( TARGET_EFM32WG_STK3800 ) 00032 #include "segmentlcdconfig_stk_wg.h" 00033 #elif defined( TARGET_EFM32ZG_STK3200 ) 00034 #error "No segment LCD available on the Zero Gecko STK." 00035 #elif defined( TARGET_EFM32HG_STK3400 ) 00036 #error "No segment LCD available on the Happy Gecko STK." 00037 #else 00038 #error "No EFM32 target STK defined." 00039 #endif 00040 00041 /***************************************************************************//** 00042 * @addtogroup Drivers 00043 * @{ 00044 ******************************************************************************/ 00045 00046 /***************************************************************************//** 00047 * @addtogroup SegmentLcd 00048 * @{ 00049 ******************************************************************************/ 00050 00051 #ifdef __cplusplus 00052 extern "C" { 00053 #endif 00054 00055 /* Regular functions */ 00056 void SegmentLCD_AllOff(void); 00057 void SegmentLCD_AllOn(void); 00058 void SegmentLCD_AlphaNumberOff(void); 00059 void SegmentLCD_ARing(int anum, int on); 00060 void SegmentLCD_Battery(int batteryLevel); 00061 void SegmentLCD_Disable(void); 00062 void SegmentLCD_EnergyMode(int em, int on); 00063 void SegmentLCD_Init(bool useBoost); 00064 void SegmentLCD_LowerHex( uint32_t num ); 00065 void SegmentLCD_LowerNumber( int num ); 00066 void SegmentLCD_Number(int value); 00067 void SegmentLCD_NumberOff(void); 00068 void SegmentLCD_Symbol(lcdSymbol s, int on); 00069 void SegmentLCD_UnsignedHex(uint16_t value); 00070 void SegmentLCD_Write(char *string); 00071 00072 #ifdef __cplusplus 00073 } 00074 #endif 00075 00076 /** @} (end group SegmentLcd) */ 00077 /** @} (end group Drivers) */ 00078 00079 #endif
Generated on Thu Jul 14 2022 05:15:53 by
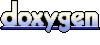