
pulls data from SD and parses 1 line
Dependencies: 4DGL-uLCD-SE SDFileSystem mbed
main.cpp
00001 //SD Import from scratch 00002 00003 #include "mbed.h" 00004 #include "SDFileSystem.h" 00005 #include "uLCD_4DGL.h" 00006 uLCD_4DGL uLCD(p28,p27,p29); 00007 SDFileSystem sd(p5, p6, p7, p8, "sd"); // the pinout on the mbed Cool Components workshop board 00008 00009 const int MAX_CHARS_PER_LINE = 17; 00010 const int MAX_TOKENS_PER_LINE = 4; 00011 const char* const DELIMITER = " "; 00012 00013 int main() { 00014 // file reading object 00015 FILE *fp = fopen("/sd/mydir/sdtest.txt", "r"); 00016 char mytext[15]; 00017 // fread(mytext, 15, 1, fp); 00018 // uLCD.printf("%s", mytext); 00019 char buf[MAX_CHARS_PER_LINE]; 00020 // read each line of file 00021 //while (!feof(fp)) // while not end of file 00022 while (fgets(buf, sizeof(buf), fp)) 00023 { 00024 // read an entire line into memory 00025 //fgets(buf,MAX_CHARS_PER_LINE,fp); //need to check this out 00026 00027 //parse line into blank-delimited tokens 00028 int n=0; // for-loop index 00029 00030 // array to store memory addresses of the tokens in buf 00031 const char* token[MAX_TOKENS_PER_LINE] = {}; // initialize to 0 00032 00033 char * pch; 00034 //printf ("Splitting string \"%s\" into tokens:\n",str); 00035 uLCD.printf("original buf: %s\n", buf); 00036 pch = strtok (buf," "); 00037 while (pch != NULL) 00038 { 00039 uLCD.printf("%s\n",pch); 00040 pch = strtok (NULL, " "); 00041 } 00042 00043 // parse line 00044 /* 00045 token[0]=strtok(buf,DELIMITER); //reminder token 00046 { 00047 for (n = 1; n < MAX_TOKENS_PER_LINE; n++) 00048 { 00049 token[n] = strtok(, DELIMITER); // subsequent tokens 00050 if (!token[n]) break; // no more tokens 00051 } 00052 }*/ 00053 00054 // process the tokens 00055 /* 00056 for (int i = 0; i < n; i++) // n = #of tokens 00057 00058 uLCD.printf("%s",token[i]); 00059 */ 00060 fclose(fp); 00061 } 00062 } 00063 00064 00065 00066 // memcpy( hour, &arr_time[0], 2 ); 00067 // memcpy( min, &arr_time[3], 2 ); 00068 00069 // arrival_hour = atoi(hour); 00070 // arrival_min = atoi(min); 00071 //uLCD.printf("\narrival hour is %d\n",arrival_hour); 00072 //uLCD.printf("arrival min is %d\n",arrival_min); 00073 00074 //ready_time = atoi(r_time); 00075 //uLCD.printf("\nString ready time is %s",r_time); 00076 // uLCD.printf("Ready Time is %d\n",ready_time); 00077 //uLCD.printf("start address is %s\n", start_add); 00078 //uLCD.printf("dest address is %s\n", dest_add); 00079 00080 00081 00082 00083
Generated on Fri Jul 15 2022 21:14:49 by
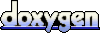