AudioRecord and FFT/MSE comparison. Call AudioRecord_demo for control record and AudioSample for subsequent recordings.
Dependencies: CMSIS_DSP_401 STM32L4xx_HAL_Driver
Fork of OneHopeOnePrayer by
main.h
00001 /** 00002 ****************************************************************************** 00003 * @file BSP/Inc/main.h 00004 * @author MCD Application Team 00005 * @version V1.1.0 00006 * @date 16-September-2015 00007 * @brief Header for main.c module 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 /* Define to prevent recursive inclusion -------------------------------------*/ 00039 #ifndef __MAIN_H 00040 #define __MAIN_H 00041 00042 /* Includes ------------------------------------------------------------------*/ 00043 #include "stdio.h" 00044 #include "string.h" 00045 #include "stm32l4xx_hal.h" 00046 #include "stm32l4xx_nucleo.h" 00047 00048 #include "arm_math.h" 00049 #include "arm_const_structs.h" 00050 #include "math.h" 00051 #include "core_cm4.h" 00052 #include "core_cm4_simd.h" 00053 #include "core_cmFunc.h" 00054 #include "core_cmInstr.h" 00055 #include "stdint.h" 00056 00057 #include "stm32l4xx_nucleo_audio.h" 00058 00059 /* Exported constants --------------------------------------------------------*/ 00060 #define DEMO_NAME_CHAR_NB 20 00061 00062 /* Defines for the Audio playing process */ 00063 #define PAUSE_STATUS ((uint32_t)0x00) /* Audio Player in Pause Status */ 00064 #define RESUME_STATUS ((uint32_t)0x01) /* Audio Player in Resume Status */ 00065 #define IDLE_STATUS ((uint32_t)0x02) /* Audio Player in Idle Status */ 00066 00067 /* Exported types ------------------------------------------------------------*/ 00068 typedef enum 00069 { 00070 IDD_RUN = 0x00, 00071 IDD_SLEEP = 0x01, 00072 IDD_LPR_2MHZ = 0x02, 00073 IDD_LPR_SLEEP = 0x03, 00074 IDD_STOP2 = 0x04, 00075 IDD_STANDBY = 0x05, 00076 IDD_SHUTDOWN = 0x06, 00077 IDD_TEST_NB = 0x07, 00078 } Idd_StateTypeDef; 00079 00080 typedef struct 00081 { 00082 void (*DemoFunc)(void); 00083 uint8_t DemoName[DEMO_NAME_CHAR_NB]; 00084 uint32_t DemoIndex; 00085 }BSP_DemoTypedef; 00086 00087 typedef enum { 00088 AUDIO_DEMO_NONE = 0, 00089 AUDIO_DEMO_PLAYBACK, 00090 AUDIO_DEMO_RECORD 00091 } Audio_DemoTypeDef; 00092 00093 /* Exported variables --------------------------------------------------------*/ 00094 /* Variable indicating which audio demo is currently running (playback v.s. record) */ 00095 extern Audio_DemoTypeDef AudioDemo; 00096 00097 /* Flag indicating that audio playback must be paused or resumed */ 00098 extern __IO uint32_t PauseResumeStatus; 00099 00100 /* Flag indicating that audio playback must be exited */ 00101 extern __IO uint8_t AudioPlaybackExit; 00102 00103 /* Flag indicating that audio playback volume level must be changed */ 00104 extern __IO uint8_t VolumeChange; 00105 00106 /* Actual audio playback volume level */ 00107 extern __IO uint8_t Volume; 00108 00109 /* Flag indicating that audio record must be exited */ 00110 extern __IO uint8_t AudioRecordExit; 00111 00112 /* Flag indicating that record sample rate has been selected */ 00113 extern __IO uint8_t AudioRecordSampleRateSelected; 00114 00115 /* Flag indicating that record sample rate must be changed changed */ 00116 extern __IO uint8_t AudioRecordSampleRateChange; 00117 00118 /* Index within SamplesRates[] */ 00119 extern __IO uint8_t SampleRateIndex; 00120 00121 /* Exported macro ------------------------------------------------------------*/ 00122 #define COUNT_OF_EXAMPLE(x) (sizeof(x)/sizeof(BSP_DemoTypedef)) 00123 #define ABS(x) (x < 0) ? (-x) : x 00124 00125 /* Exported functions ------------------------------------------------------- */ 00126 void AudioPlay_demo(void); 00127 void AudioRecord_demo(void); 00128 void Idd_demo(void); 00129 void Gyro_demo(void); 00130 void Joystick_demo (void); 00131 void LCDGlass_demo(void); 00132 void Led_demo(void); 00133 void QSPI_demo(void); 00134 uint8_t CheckForUserInput(void); 00135 void SystemClock_Config(void); 00136 void SystemLowClock_Config(void); 00137 void SystemHardwareInit(void); 00138 void SystemHardwareDeInit(void); 00139 uint32_t SystemRtcBackupRead(void); 00140 void SystemRtcBackupWrite(uint32_t SaveIndex); 00141 void fibonacci_flash(int n); 00142 00143 void Error_Handler(void); 00144 void Convert_IntegerIntoChar(uint32_t number, uint16_t *p_tab); 00145 00146 #endif /* __MAIN_H */ 00147 00148 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00149
Generated on Tue Jul 12 2022 19:27:52 by
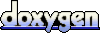