AudioRecord and FFT/MSE comparison. Call AudioRecord_demo for control record and AudioSample for subsequent recordings.
Dependencies: CMSIS_DSP_401 STM32L4xx_HAL_Driver
Fork of OneHopeOnePrayer by
Main.c
00001 #include "main.h" 00002 00003 uint32_t fftSize = 2048; 00004 uint32_t ifftFlag = 0; 00005 uint32_t doBitReverse = 0; 00006 00007 extern float32_t PWRCONTROLMSE; //CONTROL POWER MSE 00008 extern float32_t PHSCONTROLMSE; //CONTROL PHASE MSE 00009 extern float32_t CONTROLPWR0[512]; //CONTROL RECRODING 0 MAGNITUDE 00010 extern float32_t CONTROLPHASE0[512];//CONTROL RECORDING 0 PHASE 00011 00012 extern float32_t DISPLAYFFT[512]; 00013 00014 extern void AudioRecord_demo(void); 00015 extern void AudioSample(void); 00016 00017 int main(void) 00018 { 00019 HAL_Init(); 00020 00021 SystemClock_Config(); 00022 00023 //RECORDS AND COMPARES CONTROL SAMPLES 00024 AudioRecord_demo(); 00025 00026 //RECORDS AND COMPARES 1 SAMPLE TO CONTROL SAMPLE 00027 AudioSample(); 00028 00029 AudioSample(); 00030 while(1) 00031 {} 00032 00033 } 00034 void SystemClock_Config(void) 00035 { 00036 /* oscillator and clocks configs */ 00037 RCC_ClkInitTypeDef RCC_ClkInitStruct = {0}; 00038 RCC_OscInitTypeDef RCC_OscInitStruct = {0}; 00039 00040 /* The voltage scaling allows optimizing the power consumption when the device is 00041 clocked below the maximum system frequency, to update the voltage scaling value 00042 regarding system frequency refer to product datasheet. */ 00043 00044 /* Enable Power Control clock */ 00045 __HAL_RCC_PWR_CLK_ENABLE(); 00046 00047 HAL_PWREx_ControlVoltageScaling(PWR_REGULATOR_VOLTAGE_SCALE1); 00048 00049 /* Disable Power Control clock */ 00050 __HAL_RCC_PWR_CLK_DISABLE(); 00051 00052 /* 80 Mhz from MSI 8Mhz */ 00053 /* MSI is enabled after System reset, activate PLL with MSI as source */ 00054 RCC_OscInitStruct.OscillatorType = RCC_OSCILLATORTYPE_MSI; 00055 RCC_OscInitStruct.MSIState = RCC_MSI_ON; 00056 RCC_OscInitStruct.MSIClockRange = RCC_MSIRANGE_7; 00057 RCC_OscInitStruct.MSICalibrationValue = RCC_MSICALIBRATION_DEFAULT; 00058 RCC_OscInitStruct.PLL.PLLState = RCC_PLL_ON; 00059 RCC_OscInitStruct.PLL.PLLSource = RCC_PLLSOURCE_MSI; 00060 RCC_OscInitStruct.PLL.PLLM = 1; 00061 RCC_OscInitStruct.PLL.PLLN = 20; 00062 RCC_OscInitStruct.PLL.PLLR = 2; 00063 RCC_OscInitStruct.PLL.PLLP = 7; 00064 RCC_OscInitStruct.PLL.PLLQ = 4; 00065 00066 HAL_RCC_OscConfig(&RCC_OscInitStruct); 00067 00068 00069 /* Select PLL as system clock source and configure the HCLK, PCLK1 and PCLK2 00070 clocks dividers */ 00071 RCC_ClkInitStruct.ClockType = (RCC_CLOCKTYPE_SYSCLK | RCC_CLOCKTYPE_HCLK | RCC_CLOCKTYPE_PCLK1 | RCC_CLOCKTYPE_PCLK2); 00072 RCC_ClkInitStruct.SYSCLKSource = RCC_SYSCLKSOURCE_PLLCLK; 00073 RCC_ClkInitStruct.AHBCLKDivider = RCC_SYSCLK_DIV1; 00074 RCC_ClkInitStruct.APB1CLKDivider = RCC_HCLK_DIV1; 00075 RCC_ClkInitStruct.APB2CLKDivider = RCC_HCLK_DIV1; 00076 HAL_RCC_ClockConfig(&RCC_ClkInitStruct, FLASH_LATENCY_4); 00077 00078 /* The voltage scaling allows optimizing the power consumption when the device is 00079 clocked below the maximum system frequency, to update the voltage scaling value 00080 regarding system frequency refer to product datasheet. */ 00081 00082 /* Enable Power Control clock */ 00083 __HAL_RCC_PWR_CLK_ENABLE(); 00084 00085 HAL_PWREx_ControlVoltageScaling(PWR_REGULATOR_VOLTAGE_SCALE1); 00086 00087 /* Disable Power Control clock */ 00088 __HAL_RCC_PWR_CLK_DISABLE(); 00089 }
Generated on Tue Jul 12 2022 19:27:52 by
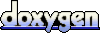