
SCPI interface to SX1272 and SX1276
Dependencies: SX127x lib_gps lib_mma8451q lib_mpl3115a2 lib_sx9500 libscpi mbed
test_interactive.cpp
00001 /*- 00002 * Copyright (c) 2012-2013 Jan Breuer, 00003 * 00004 * All Rights Reserved 00005 * 00006 * Redistribution and use in source and binary forms, with or without 00007 * modification, are permitted provided that the following conditions are 00008 * met: 00009 * 1. Redistributions of source code must retain the above copyright notice, 00010 * this list of conditions and the following disclaimer. 00011 * 2. Redistributions in binary form must reproduce the above copyright 00012 * notice, this list of conditions and the following disclaimer in the 00013 * documentation and/or other materials provided with the distribution. 00014 * 00015 * THIS SOFTWARE IS PROVIDED BY THE AUTHORS ``AS IS'' AND ANY EXPRESS OR 00016 * IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00017 * WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00018 * DISCLAIMED. IN NO EVENT SHALL THE AUTHORS OR CONTRIBUTORS BE LIABLE 00019 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR 00020 * CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF 00021 * SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR 00022 * BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 00023 * WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE 00024 * OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN 00025 * IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00026 */ 00027 00028 /** 00029 * @file main.c 00030 * @date Thu Nov 15 10:58:45 UTC 2012 00031 * 00032 * @brief SCPI parser test 00033 * 00034 * 00035 */ 00036 00037 #include <stdio.h> 00038 #include <stdlib.h> 00039 #include <string.h> 00040 #include "scpi/scpi.h" 00041 #include "../common/scpi-def.h" 00042 #include "mbed.h" 00043 #include "timer.h" 00044 00045 00046 00047 Serial pc(USBTX, USBRX); 00048 00049 #ifdef TARGET_MOTE_L152RC 00050 #define LED_TIMEOUT 50000 // usec 00051 DigitalOut RedLed( PB_1 ); // Active Low 00052 DigitalOut YellowLed( PB_10 ); // Active Low 00053 DigitalOut UsrLed( PA_5 ); // Active High 00054 TimerEvent_t RedLedTimer; 00055 static void OnInputEvent( void ); 00056 #endif /* TARGET_MOTE_L152RC */ 00057 00058 #ifdef TARGET_MOTE_L152RC 00059 #endif /* TARGET_MOTE_L152RC */ 00060 00061 00062 char smbuffer[64]; 00063 00064 size_t SCPI_Write(scpi_t * context, const char * data, size_t len) { 00065 (void) context; 00066 return fwrite(data, 1, len, stdout); 00067 } 00068 00069 scpi_result_t SCPI_Flush(scpi_t * context) { 00070 fflush(stdout); 00071 return SCPI_RES_OK; 00072 } 00073 00074 int SCPI_Error(scpi_t * context, int_fast16_t err) { 00075 (void) context; 00076 00077 printf("**ERROR: %d, \"%s\"\r\n", (int32_t) err, SCPI_ErrorTranslate(err)); 00078 return 0; 00079 } 00080 00081 scpi_result_t SCPI_Control(scpi_t * context, scpi_ctrl_name_t ctrl, scpi_reg_val_t val) { 00082 if (SCPI_CTRL_SRQ == ctrl) { 00083 printf("**SRQ: 0x%X (%d)\r\n", val, val); 00084 } else { 00085 printf("**CTRL %02x: 0x%X (%d)\r\n", ctrl, val, val); 00086 } 00087 return SCPI_RES_OK; 00088 } 00089 00090 /** 00091 * Return 0 as OK and other number as error 00092 */ 00093 scpi_result_t SCPI_Test(scpi_t * context) { 00094 printf("**Test\r\n"); 00095 return (scpi_result_t)0; 00096 } 00097 00098 00099 00100 scpi_result_t SCPI_SystemCommTcpipControlQ(scpi_t * context) { 00101 return SCPI_RES_ERR; 00102 } 00103 /* 00104 * 00105 */ 00106 int get_kbd_str(char* buf, int size) 00107 { 00108 char c; 00109 int i; 00110 static int prev_len; 00111 00112 for (i = 0;;) { 00113 if (pc.readable()) { 00114 c = pc.getc(); 00115 if (c == 8) { // backspace 00116 if (i > 0) { 00117 pc.putc(8); 00118 pc.putc(' '); 00119 pc.putc(8); 00120 i--; 00121 } 00122 } else if (c == '\r') { 00123 if (i == 0) { 00124 return prev_len; // repeat previous 00125 } else { 00126 buf[i++] = c; 00127 buf[i] = 0; // null terminate 00128 prev_len = i; 00129 pc.putc('\r'); 00130 pc.putc('\n'); 00131 return i; 00132 } 00133 } else if (c == 3) { 00134 // ctrl-C abort 00135 //abort_key = true; 00136 return -1; 00137 } else if (i < size) { 00138 buf[i++] = c; 00139 pc.putc(c); 00140 } 00141 } else { 00142 /* service something */ 00143 service_radio(); 00144 } 00145 } // ...for() 00146 } 00147 00148 int main(int argc, char** argv) { 00149 (void) argc; 00150 (void) argv; 00151 //int result; 00152 00153 scpi_def_init(); 00154 00155 SCPI_Init(&scpi_context); 00156 00157 printf("\r\nreset\r\n"); 00158 00159 00160 #ifdef TARGET_MOTE_L152RC 00161 RedLed = 1; 00162 YellowLed = 1; 00163 UsrLed = 1; 00164 TimerInit( &RedLedTimer, OnInputEvent); 00165 #endif /* TARGET_MOTE_L152RC */ 00166 00167 00168 00169 while (1) { 00170 //fgets(smbuffer, 10, stdin); 00171 00172 int len = get_kbd_str(smbuffer, sizeof(smbuffer)); 00173 if (len > 0) { 00174 #ifdef TARGET_MOTE_L152RC 00175 /* Blink Red LED on keyboard entry */ 00176 RedLed = 0; 00177 TimerSetValue( &RedLedTimer, LED_TIMEOUT ); // set blink LED to 750ms 00178 TimerStart( &RedLedTimer ); 00179 #endif /* TARGET_MOTE_L152RC */ 00180 /*result =*/ SCPI_Input(&scpi_context, smbuffer, len); 00181 } 00182 } 00183 00184 //return (EXIT_SUCCESS); 00185 } 00186 00187 #ifdef TARGET_MOTE_L152RC 00188 static void OnInputEvent( void ) 00189 { 00190 TimerStop( &RedLedTimer); 00191 00192 RedLed = 1; 00193 } 00194 #endif /* TARGET_MOTE_L152RC */
Generated on Thu Jul 14 2022 02:00:57 by
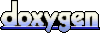